A Playlist should also support the following behaviors: ⚫ addSong() Adds a Song to the end of the playlist. Takes in the Song to add and returns nothing. Does not add null Song references. removeSong() Removes a specific Song from the playlist. -Takes in the Song to be removed and returns a boolean representing whether the song was removed. NOTE: don't rely on reference equality toArray() - Converts songs to an array and returns the array. topAndWorst Song () - Returns an array with two Songs. The first element in the array should be the top Song and the second element in the array should be the worst Song in the playlist. Both are decided based on the like factor. - If there is a tie between the top Song and the worst Song, return the same Song for the top Song and the worst Song. - If there is a tie for top or worst Song, use the first Song encountered. Returns (top song, worst song} toString() Returns in the format: - {title} has (number of songs} songs, is (duration) long, has a like factor of (like factor), and was created (dateCreated} days after the platform released. equals() -Should override Object's equals A Playlist is equal to another Playlist if they have the same name, owner, number of songs, and duration. compareTo() Playlists should be compared to each other based on their creation date. - For our platform, a playlist is greater than another if it was created more recently. Getter and setter methods for instance variables as required. For setters, note that if an invalid value is passed in, you should not modify the value of the instance variable. Any additional methods required to satisfy the interfaces Playlist implements.
Problem Description: Greetings aspiring music enthusiasts! You have been recruited by a top music streaming service to develop a cutting-edge music player application! Your mission is to create an app that allows users to
manage their playlists and listen to their favorite tunes.
The Challenge: The music industry faces a critical challenge – users crave a personalized and engaging
way to experience their favorite tunes! Your mission is to create an innovative application that goes
beyond basic playback.
Your Objectives: Develop the Maestro’s Toolkit: Define a robust music player which involves Users, Playlists,
and Songs. Each user will be able to create and manage their personal music collections using Playlists, which have a variety of Songs.
Craft the Perfect Symphony: Implement functionalities that make your music player stand out!
- Seamless Playlist Management: Users should be able to create or delete Playlists. Additionally, the functionality to customize existing Playlists is crucial.
- Curated Listening Experience: Let Users express their preferences through like and dislike functionality on both Playlists and Songs. Additionally, we want to allow Users to customize their Playlist with features like selecting the top and worst Song in the Playlist.
- Advanced Music Control: Users should be able to “play” and “pause” songs on demand.
By successfully completing this mission, you'll not only develop valuable programming skills, but you'll
also establish yourself as a legendary Music Maestro, crafting extraordinary musical experiences for all!
Solution Description: In the interest of encapsulation, data members for the following class should only be
accessible from other classes using getters and/or setters where needed. The described methods and
constructors for this assignment should be public. It is OK to write private helper methods. When you write your
solution, you’ll need to exactly match the instance variable names listed in this document. Further, your
Strings should exactly match our expected format. Minor discrepancies, including whitespace and
punctuation issues, can result in failed test cases.
- The String formats in the PDF sometimes wrap to another line due to their length -- you are not
expected to include newline characters in them.
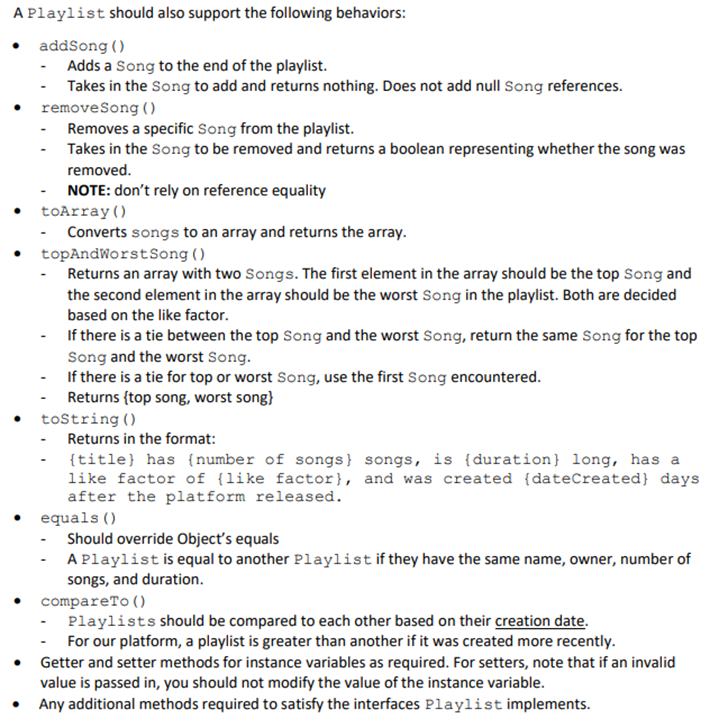
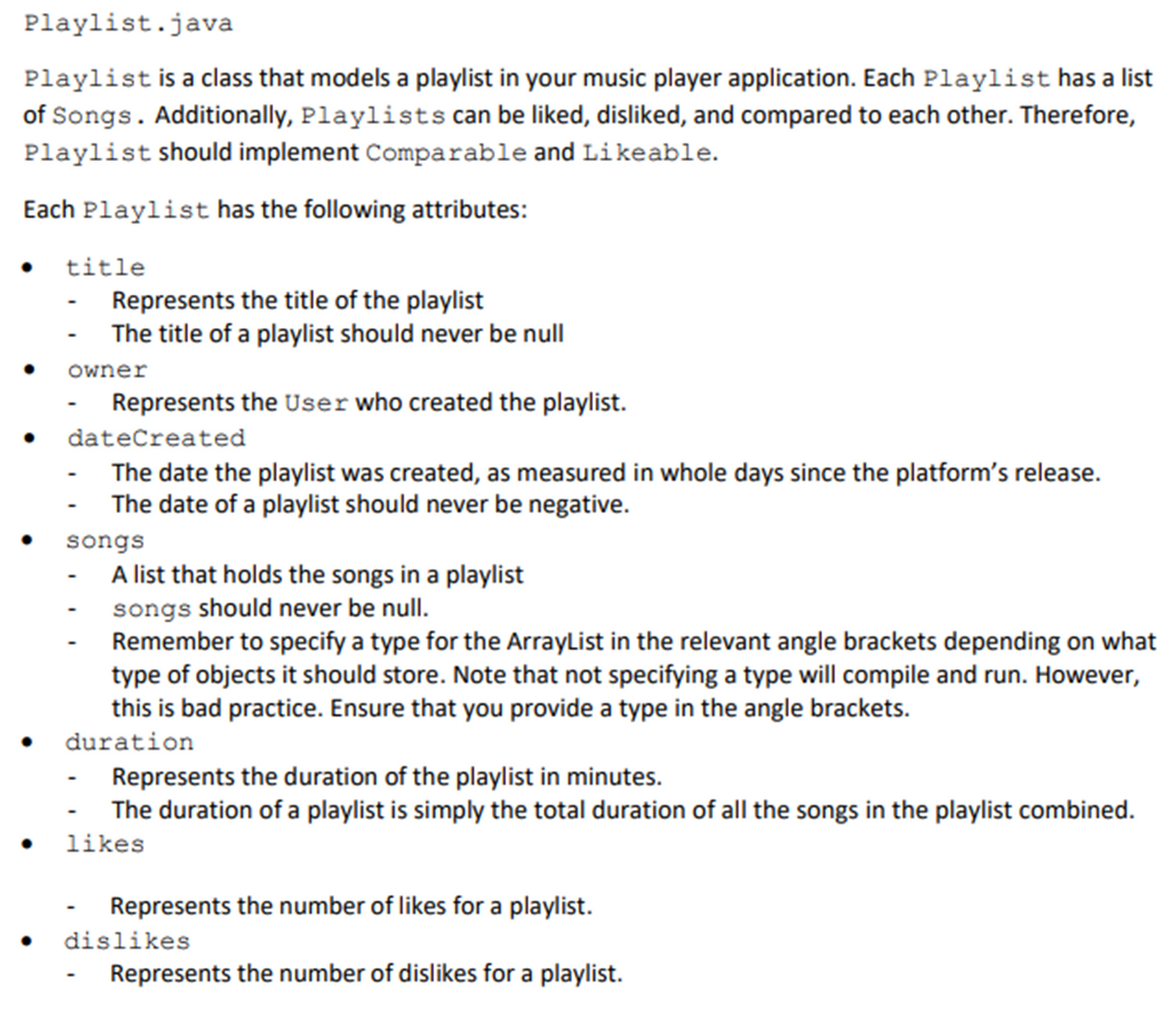

Step by step
Solved in 4 steps with 13 images

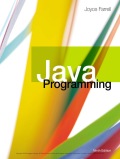
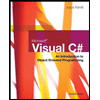
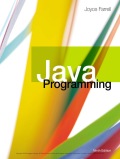
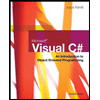