can you help me figure out why my program doesn't run? the fatal error I get is "Student must be defined in its own file", but that doesn't seem helpful? here's my code: package student; public class Sort { public static void sort(Student[] studentArr) { int minIndex = 0; for(int i = 0; i < studentArr.length; i++) { minIndex = i; for(int j = i+1; j< studentArr.length; j++) { if(studentArr[j].compareTo(studentArr[minIndex]) < 0) { minIndex = j; } } Student temp = studentArr[i]; studentArr[i] = studentArr[minIndex]; studentArr[minIndex] = temp; } } public static void main (String[] args) { Student[] studentArr = { new Student("Lat", 4.0), new Student("Ampere", 2.4), new Student("Jing", 3.0), new Student("Memphis", 3.1) }; System.out.println("The students are: "); for(Student s: studentArr) System.out.println(s); sort(studentArr); System.out.println("Students after sorting are:"); for(Student s: studentArr) System.out.println(s); } } public class Student implements Comparable { private int studentID; private String name; private double gpa; private static int maxStudentID; public Student() { maxStudentID++; this.studentID = maxStudentID; } public Student(String name, double gpa) { this(); this.name = name; this.gpa = gpa; } public int getStudentID() { return studentID; } public String getName() { return name; } public void setName(String name) { this.name = name; } public double getGpa() { return gpa; } public void setGpa(double dpa) { this.gpa = gpa; } @Override public String toString() { return "Student [ID=" + studentID + ", name:" + name + ", GPA=" + gpa+ "]"; } @Override public int compareTo(Student otherStudent) { if(this.studentID < otherStudent.studentID) return -1; if(this.studentID > otherStudent.studentID) return 1; return 0; } }
can you help me figure out why my program doesn't run? the fatal error I get is "Student must be defined in its own file", but that doesn't seem helpful?
here's my code:
package student;
public class Sort {
public static void sort(Student[] studentArr) {
int minIndex = 0;
for(int i = 0; i < studentArr.length; i++) {
minIndex = i;
for(int j = i+1; j< studentArr.length; j++) {
if(studentArr[j].compareTo(studentArr[minIndex]) < 0) {
minIndex = j;
}
}
Student temp = studentArr[i];
studentArr[i] = studentArr[minIndex];
studentArr[minIndex] = temp;
}
}
public static void main (String[] args) {
Student[] studentArr = {
new Student("Lat", 4.0),
new Student("Ampere", 2.4),
new Student("Jing", 3.0),
new Student("Memphis", 3.1)
};
System.out.println("The students are: ");
for(Student s: studentArr)
System.out.println(s);
sort(studentArr);
System.out.println("Students after sorting are:");
for(Student s: studentArr)
System.out.println(s);
}
}
public class Student implements Comparable <Student> {
private int studentID;
private String name;
private double gpa;
private static int maxStudentID;
public Student() {
maxStudentID++;
this.studentID = maxStudentID;
}
public Student(String name, double gpa) {
this();
this.name = name;
this.gpa = gpa;
}
public int getStudentID() {
return studentID;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getGpa() {
return gpa;
}
public void setGpa(double dpa) {
this.gpa = gpa;
}
@Override
public String toString() {
return "Student [ID=" + studentID + ", name:" + name + ", GPA=" + gpa+ "]";
}
@Override
public int compareTo(Student otherStudent) {
if(this.studentID < otherStudent.studentID)
return -1;
if(this.studentID > otherStudent.studentID)
return 1;
return 0;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

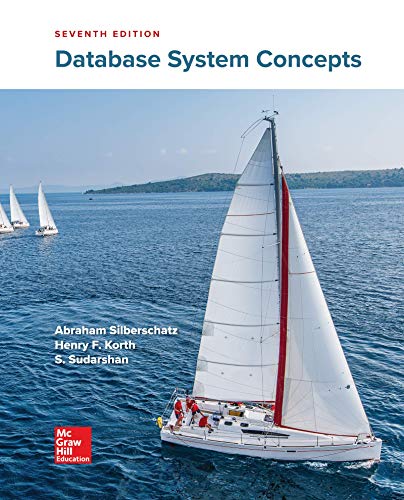
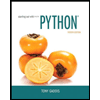
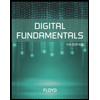
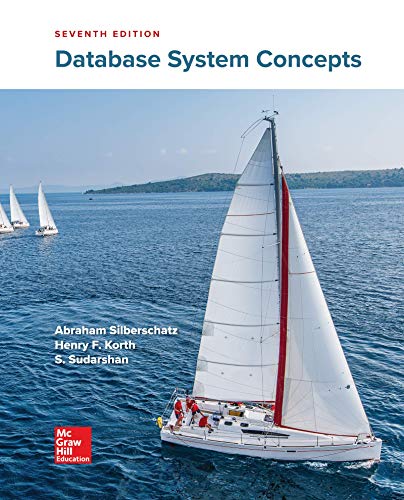
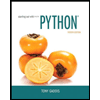
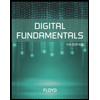
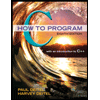
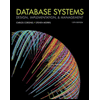
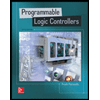