In this question, you will design two classes Flight and Itinerary and write a program to test them. Let's first look at the java.util. GregorianCalendar class which you will use in designing your Flight and Itinerary classes. This class provides the standard calendar system used by most of the world. It has a constructor GregorianCalender (int year, int month, int dayOfMonth, int hour, int minute, int second) which creates a GregorianCalendar object with the given date and time set for the default time zone with the default locale. The month parameter is 0 based, that is, 0 for January. It has a method get TimeInMillis() (inherited from its parent class java.util.Calendar) that returns this Calendar's time in milliseconds (elapsed since midnight, January 1, 1970 GMT) which is in long type. Now let's design the two classes Flight and Itinerary. The Flight class stores the information about a flight with the following members: • A private String data field named flight No for the flight number. • A private GregorianCalendar data field named departureTime. A private GregorianCalendar data field named arrivalTime.
In this question, you will design two classes Flight and Itinerary and write a program to test them. Let's first look at the java.util. GregorianCalendar class which you will use in designing your Flight and Itinerary classes. This class provides the standard calendar system used by most of the world. It has a constructor GregorianCalender (int year, int month, int dayOfMonth, int hour, int minute, int second) which creates a GregorianCalendar object with the given date and time set for the default time zone with the default locale. The month parameter is 0 based, that is, 0 for January. It has a method get TimeInMillis() (inherited from its parent class java.util.Calendar) that returns this Calendar's time in milliseconds (elapsed since midnight, January 1, 1970 GMT) which is in long type. Now let's design the two classes Flight and Itinerary. The Flight class stores the information about a flight with the following members: • A private String data field named flight No for the flight number. • A private GregorianCalendar data field named departureTime. A private GregorianCalendar data field named arrivalTime.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
Write the code in java and please dont plagarise or copy from other sources write it on your own and read the question carefully and do what in the question says thank you.
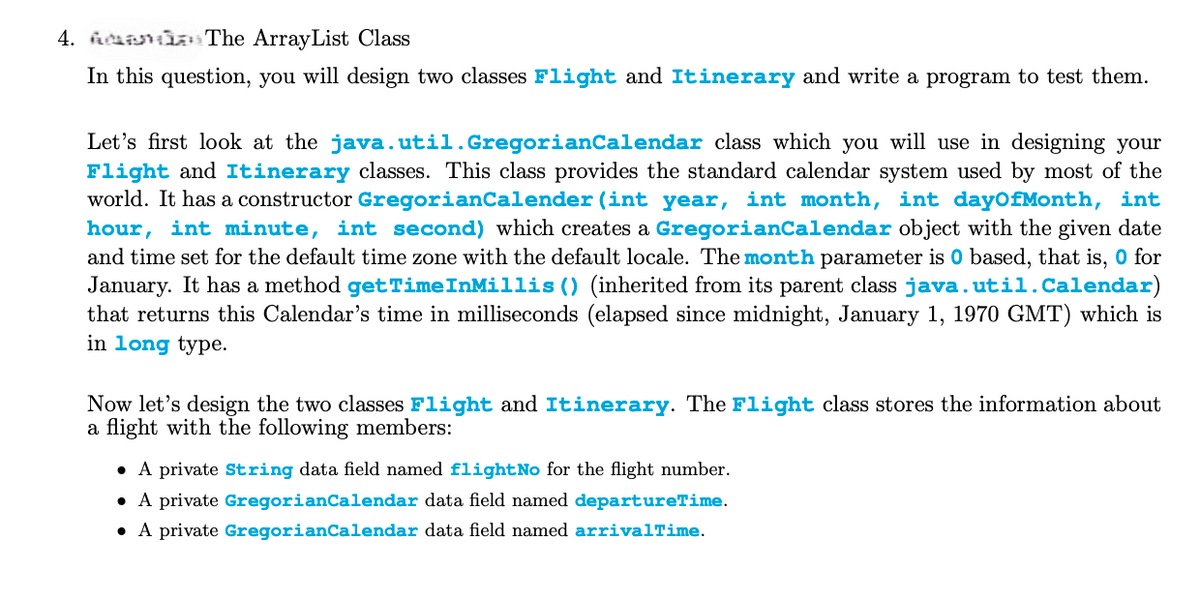
Transcribed Image Text:The ArrayList Class
4.
In this question, you will design two classes Flight and Itinerary and write a program to test them.
Let's first look at the java.util.GregorianCalendar class which you will use in designing your
Flight and Itinerary classes. This class provides the standard calendar system used by most of the
world. It has a constructor GregorianCalender (int year, int month, int dayOfMonth, int
hour, int minute, int second) which creates a GregorianCalendar object with the given date
and time set for the default time zone with the default locale. The month parameter is 0 based, that is, o for
January. It has a method get TimeInMillis() (inherited from its parent class java.util.Calendar)
that returns this Calendar's time in milliseconds (elapsed since midnight, January 1, 1970 GMT) which is
in long type.
Now let's design the two classes Flight and Itinerary. The Flight class stores the information about
a flight with the following members:
• A private String data field named flight No for the flight number.
• A private GregorianCalendar data field named departureTime.
• A private GregorianCalendar data field named arrivalTime.
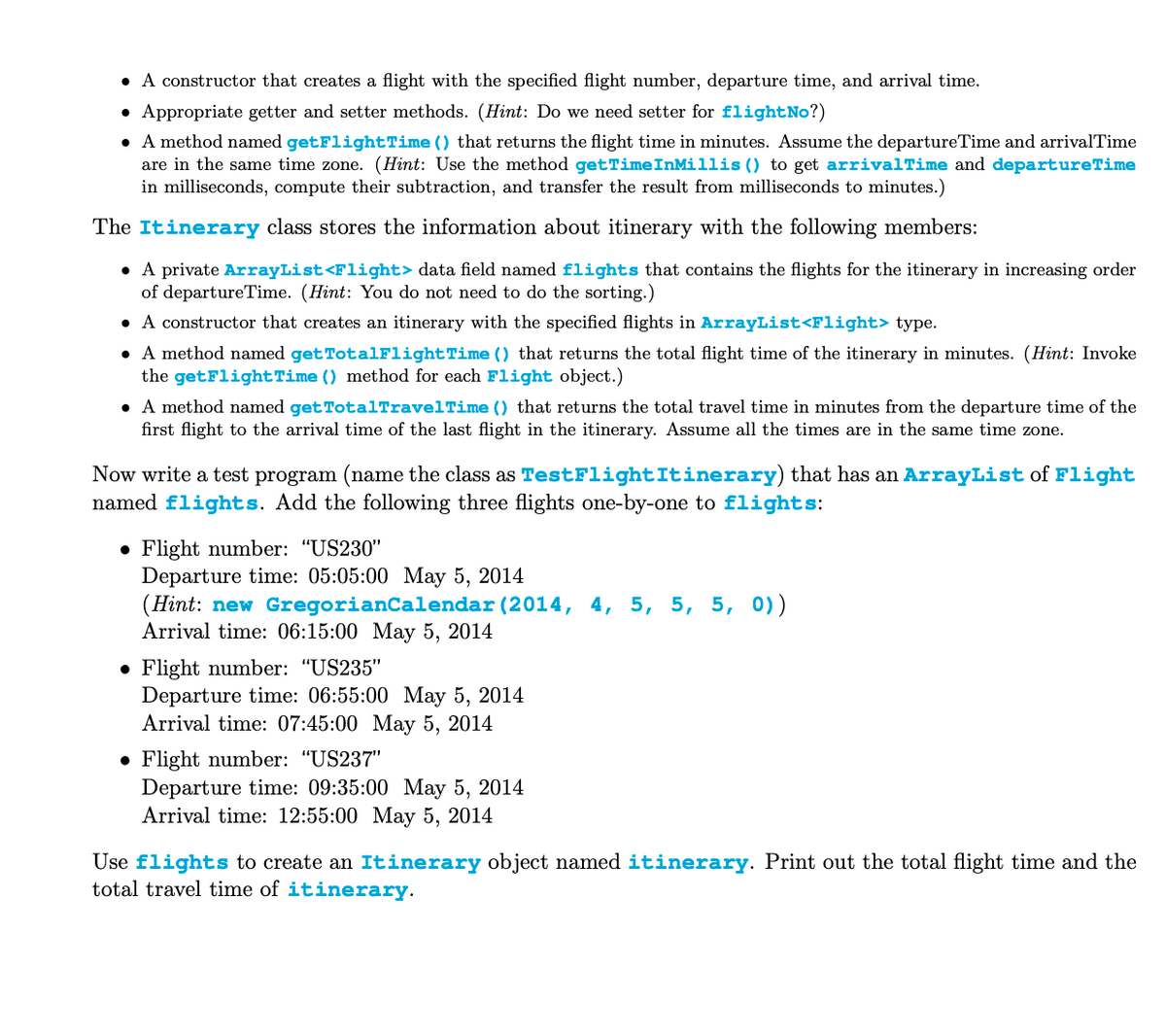
Transcribed Image Text:• A constructor that creates a flight with the specified flight number, departure time, and arrival time.
• Appropriate getter and setter methods. (Hint: Do we need setter for flight No?)
• A method named get Flight Time () that returns the flight time in minutes. Assume the departure Time and arrival Time
are in the same time zone. (Hint: Use the method getTime InMillis() to get arrival Time and departureTime
in milliseconds, compute their subtraction, and transfer the result from milliseconds to minutes.)
The Itinerary class stores the information about itinerary with the following members:
• A private ArrayList<Flight> data field named flights that contains the flights for the itinerary in increasing order
of departure Time. (Hint: You do not need to do the sorting.)
• A constructor that creates an itinerary with the specified flights in ArrayList<Flight> type.
• A method named get TotalFlight Time () that returns the total flight time of the itinerary in minutes. (Hint: Invoke
the getFlight Time () method for each Flight object.)
• A method named get TotalTravelTime () that returns the total travel time in minutes from the departure time of the
first flight to the arrival time of the last flight in the itinerary. Assume all the times are in the same time zone.
Now write a test program (name the class as TestFlight Itinerary) that has an ArrayList of Flight
named flights. Add the following three flights one-by-one to flights:
Flight number: "US230"
Departure time: 05:05:00 May 5, 2014
(Hint: new GregorianCalendar (2014, 4, 5, 5, 5, 0))
Arrival time: 06:15:00 May 5, 2014
• Flight number: "US235"
Departure time: 06:55:00 May 5, 2014
Arrival time: 07:45:00 May 5, 2014
• Flight number: "US237"
Departure time: 09:35:00 May 5, 2014
Arrival time: 12:55:00 May 5, 2014
Use flights to create an Itinerary object named itinerary. Print out the total flight time and the
total travel time of itinerary.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
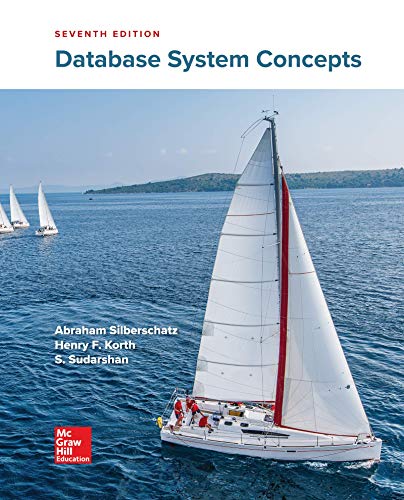
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
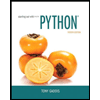
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
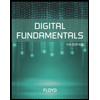
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
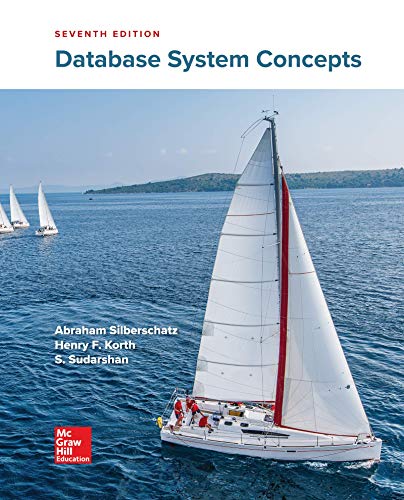
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
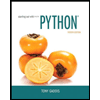
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
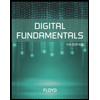
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
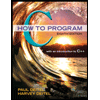
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
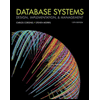
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
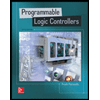
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education