Task: Overriding (Redesign the CanadianAddress class) This task will rewrite the CanadianAddress class you implemented in Lab 12 with overridden methods. The new class is also called CanadianAddress. If you do need the old CanadianAddress class, put this new class in a different package. Rewrite the CanadianAddress class: • From your implementation of the CanadianAddress class in Lab 12, delete the methods printAddress() and equalAddresses(). • You will replace the functionalities provided in the old printAddress() method with the following two steps: 1. In the CanadianAddress class, override the toString() method in the Object class, which returns a string in the format used by the old printAddress() method: streetAddress + "," + city + "\n" + province + " " + postalCode + ", Canada". Use the @Override annotation to make sure you override the toString() method correctly. 2. This toString() method will be implicitly used in the printing methods in your test program to print out an address. • You will replace the functionalities provided in the old equalAddresses() method in this step. Override the equals() method in the Object class. Note that the header of the method should be: public boolean equals(Object address), where the parameter is of Object type. Use the @Override annotation to make sure you override the equals() method correctly.
Task: Overriding (Redesign the CanadianAddress class) This task will rewrite the CanadianAddress class you implemented in Lab 12 with overridden methods. The new class is also called CanadianAddress. If you do need the old CanadianAddress class, put this new class in a different package. Rewrite the CanadianAddress class: • From your implementation of the CanadianAddress class in Lab 12, delete the methods printAddress() and equalAddresses(). • You will replace the functionalities provided in the old printAddress() method with the following two steps: 1. In the CanadianAddress class, override the toString() method in the Object class, which returns a string in the format used by the old printAddress() method: streetAddress + "," + city + "\n" + province + " " + postalCode + ", Canada". Use the @Override annotation to make sure you override the toString() method correctly. 2. This toString() method will be implicitly used in the printing methods in your test program to print out an address. • You will replace the functionalities provided in the old equalAddresses() method in this step. Override the equals() method in the Object class. Note that the header of the method should be: public boolean equals(Object address), where the parameter is of Object type. Use the @Override annotation to make sure you override the equals() method correctly.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write the answer in java and read the question carefully here is the lab 12 code :
public class lab12daneshelahi
{
public static void main (String[]args)
{
CanadianAddress testAddress = new CanadianAddress ();
CanadianAddress SMUAddress =
new CanadianAddress ("923 Robie St.", "Halifax", "Nova Scotia",
"B3H 3C3");
System.out.println ("The testAddress is created as:");
testAddress.printAddress ();
System.out.println ();
System.out.println ("The SMUAddress is created as:");
SMUAddress.printAddress ();
System.out.println ();
System.out.println ("The testAddress is equal to SMUAddress? :( " +
testAddress.equalAddress (SMUAddress));
System.out.println ();
testAddress.setStreetAddress ("923 Robie St.");
testAddress.setCity ("Halifax");
testAddress.setProvince ("Nova Scotia");
testAddress.setPostalCode ("B3H 3C3");
System.out.println ("The testAddress is updated as: :D ");
testAddress.printAddress ();
System.out.println ();
System.out.println ("The testAddress is equal to SMUAddress? :) " +
testAddress.equalAddress (SMUAddress));
}
}
class CanadianAddress
{
private String streetAddress;
private String city;
private String province;
private String postalCode;
public CanadianAddress ()
{
}
public CanadianAddress (String streetAddress, String city, String province,
String postalCode)
{
this.streetAddress = streetAddress;
this.city = city;
this.province = province;
this.postalCode = postalCode;
}
public String getStreetAddress ()
{
return streetAddress;
}
public void setStreetAddress (String streetAddress)
{
this.streetAddress = streetAddress;
}
public String getCity ()
{
return city;
}
public void setCity (String city)
{
this.city = city;
}
public String getProvince ()
{
return province;
}
public void setProvince (String province)
{
this.province = province;
}
public String getPostalCode ()
{
return postalCode;
}
public void setPostalCode (String postalCode)
{
this.postalCode = postalCode;
}
public void printAddress ()
{
System.out.println ((streetAddress != null ? streetAddress : "null") +
", " + (city != null ? city : "null") + ",");
System.out.println ((province != null ? province : "null") + " " +
(postalCode !=
null ? postalCode : "null") + ", Canada");
}
public boolean equalAddress (CanadianAddress address)
{
return (this.streetAddress == null ? address.streetAddress ==
null : this.streetAddress.equals (address.streetAddress))
&& (this.city == null ? address.city ==
null : this.city.equals (address.city))
&& (this.province == null ? address.province ==
null : this.province.equals (address.province))
&& (this.postalCode == null ? address.postalCode ==
null : this.postalCode.equals (address.postalCode));
}
}
Please follow what in the question says and dont copy from other sources and dont plagarise please. Thank you.
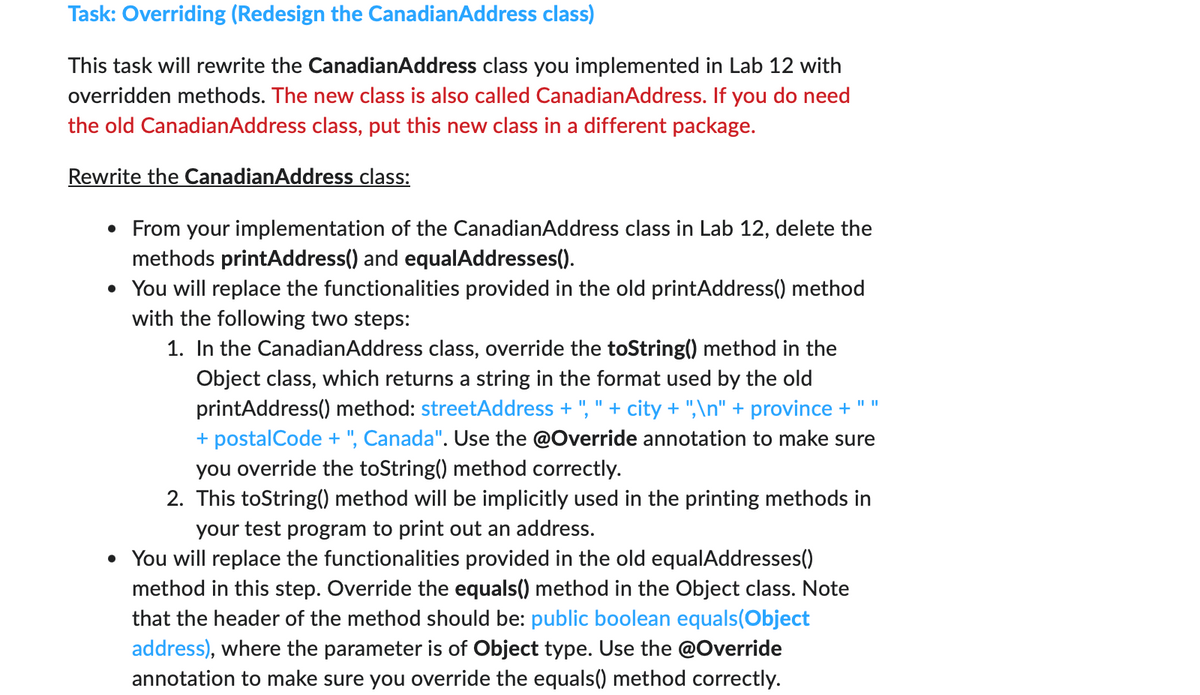
Transcribed Image Text:Task: Overriding (Redesign the CanadianAddress class)
This task will rewrite the CanadianAddress class you implemented in Lab 12 with
overridden methods. The new class is also called CanadianAddress. If you do need
the old CanadianAddress class, put this new class in a different package.
Rewrite the CanadianAddress class:
• From your implementation of the CanadianAddress class in Lab 12, delete the
methods printAddress() and equalAddresses().
• You will replace the functionalities provided in the old printAddress() method
with the following two steps:
1. In the CanadianAddress class, override the toString() method in the
Object class, which returns a string in the format used by the old
printAddress() method: streetAddress + "," + city + ",\n" + province + " "
+ postalCode + ", Canada". Use the @Override annotation to make sure
you override the toString() method correctly.
2. This toString() method will be implicitly used in the printing methods in
your test program to print out an address.
• You will replace the functionalities provided in the old equalAddresses()
method in this step. Override the equals() method in the Object class. Note
that the header of the method should be: public boolean equals(Object
address), where the parameter is of Object type. Use the @Override
annotation to make sure you override the equals() method correctly.
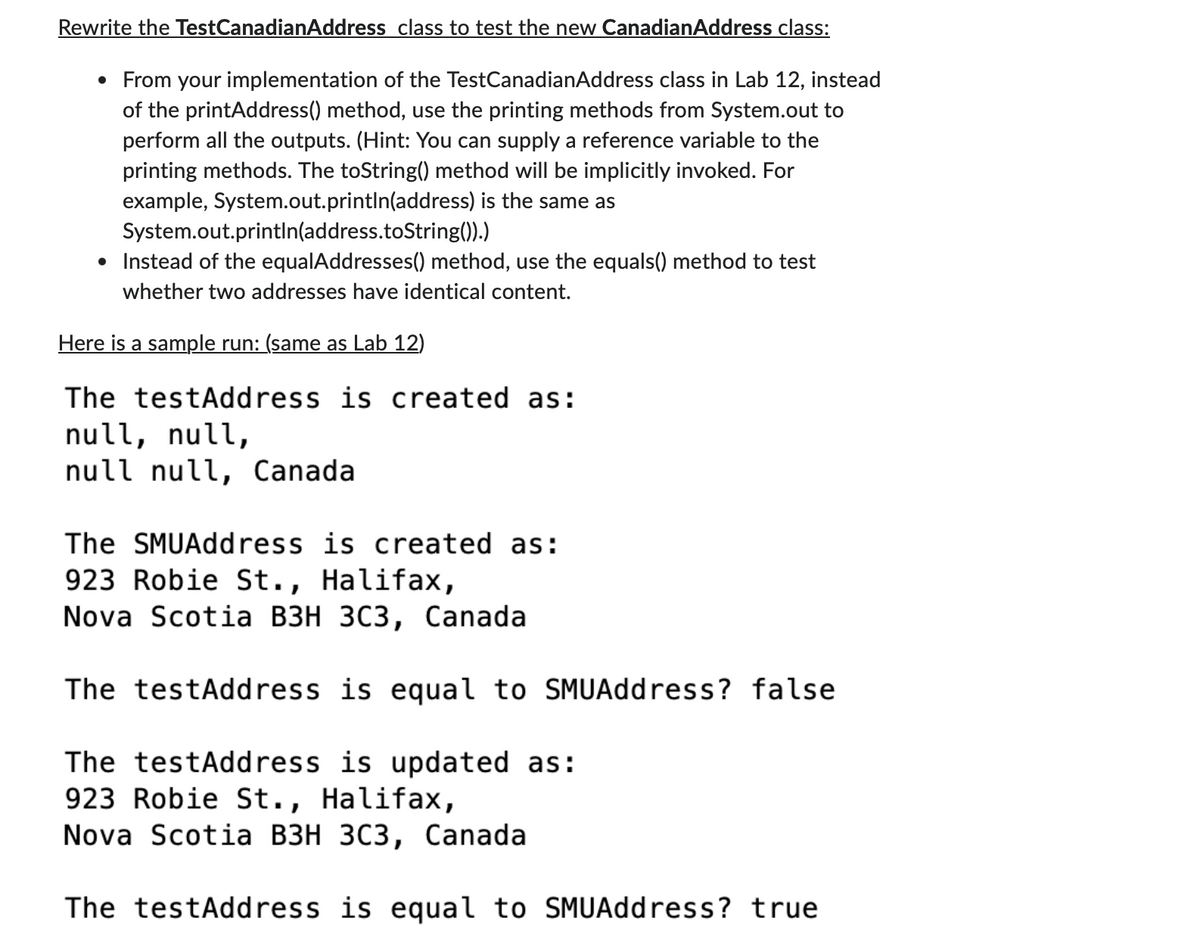
Transcribed Image Text:Rewrite the TestCanadianAddress class to test the new CanadianAddress class:
• From your implementation of the TestCanadianAddress class in Lab 12, instead
of the printAddress() method, use the printing methods from System.out to
perform all the outputs. (Hint: You can supply a reference variable to the
printing methods. The toString() method will be implicitly invoked. For
example, System.out.println(address) is the same as
System.out.println(address.toString()).)
• Instead of the equalAddresses() method, use the equals() method to test
whether two addresses have identical content.
Here is a sample run: (same as Lab 12)
The testAddress is created as:
null, null,
null null, Canada
The SMUAddress is created as:
923 Robie St., Halifax,
Nova Scotia B3H 3C3, Canada
The testAddress is equal to SMUAddress? false
The testAddress is updated as:
923 Robie St., Halifax,
Nova Scotia B3H 3C3, Canada
The testAddress is equal to SMUAddress? true
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
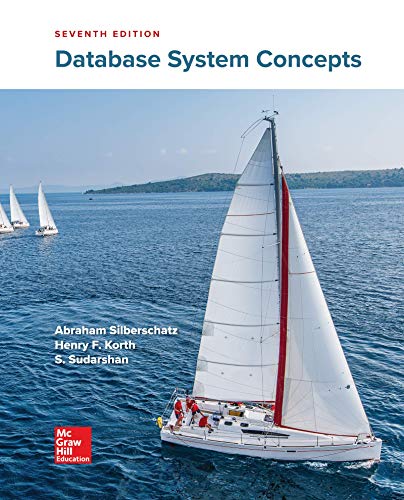
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
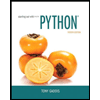
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
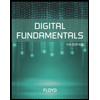
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
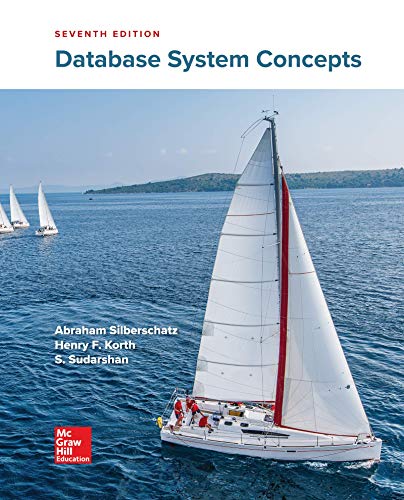
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
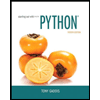
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
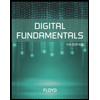
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
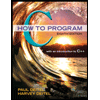
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
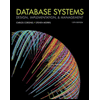
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
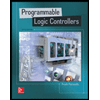
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education