1) largest Write a function int largest(int a],int n) that will find the largest int in the n ints in a. This should be the only function in the file. Generate assembly language for this code using -S. Identify the variables in this code, defining a table in comments in the code. Comment each line of code, indicating what it is doing in terms of the original C code.
This is what I have so far I need help with the commenting up the code
My c code
#include <stdio.h>
int largest(int a[], int n);
int largest(int a[], int n)
{
int large=a[0];
for(int i=1;i<n;i++)
{
if(large<a[i])
{
large=a[i];
}
}
return large;
}
int main()
{
int n;
int largest_num;
int a[50];
printf("enter the size of an array");
scanf("%d",&n);
printf("\n Enter the elements in an array:");
for(int i=0;i<n;i++)
{
scanf("%d",&a[i]);
}
largest_num=largest(a,n);
printf("Largest number in array:%d",largest_num);
return 0;
}
My assembly code
largest(int*, int):
push rbp
mov rbp, rsp
mov QWORD PTR [rbp-24], rdi
mov DWORD PTR [rbp-28], esi
mov rax, QWORD PTR [rbp-24]
mov eax, DWORD PTR [rax]
mov DWORD PTR [rbp-4], eax
mov DWORD PTR [rbp-8], 1
jmp .L2
.L4:
mov eax, DWORD PTR [rbp-8]
cdqe
lea rdx, [0+rax*4]
mov rax, QWORD PTR [rbp-24]
add rax, rdx
mov eax, DWORD PTR [rax]
cmp DWORD PTR [rbp-4], eax
jge .L3
mov eax, DWORD PTR [rbp-8]
cdqe
lea rdx, [0+rax*4]
mov rax, QWORD PTR [rbp-24]
add rax, rdx
mov eax, DWORD PTR [rax]
mov DWORD PTR [rbp-4], eax
.L3:
add DWORD PTR [rbp-8], 1
.L2:
mov eax, DWORD PTR [rbp-8]
cmp eax, DWORD PTR [rbp-28]
jl .L4
mov eax, DWORD PTR [rbp-4]
pop rbp
ret
.LC0:
.string "enter the size of an array"
.LC1:
.string "%d"
.LC2:
.string "\n Enter the elements in an array:"
.LC3:
.string "Largest number in array:%d"
main:
push rbp
mov rbp, rsp
sub rsp, 224
mov edi, OFFSET FLAT:.LC0
mov eax, 0
call printf
lea rax, [rbp-12]
mov rsi, rax
mov edi, OFFSET FLAT:.LC1
mov eax, 0
call __isoc99_scanf
mov edi, OFFSET FLAT:.LC2
mov eax, 0
call printf
mov DWORD PTR [rbp-4], 0
jmp .L7
.L8:
lea rdx, [rbp-224]
mov eax, DWORD PTR [rbp-4]
cdqe
sal rax, 2
add rax, rdx
mov rsi, rax
mov edi, OFFSET FLAT:.LC1
mov eax, 0
call __isoc99_scanf
add DWORD PTR [rbp-4], 1
.L7:
mov eax, DWORD PTR [rbp-12]
cmp DWORD PTR [rbp-4], eax
jl .L8
mov edx, DWORD PTR [rbp-12]
lea rax, [rbp-224]
mov esi, edx
mov rdi, rax
call largest(int*, int)
mov DWORD PTR [rbp-8], eax
mov eax, DWORD PTR [rbp-8]
mov esi, eax
mov edi, OFFSET FLAT:.LC3
mov eax, 0
call printf
mov eax, 0
leave
ret
![1) largest
Write a function int largest(int a[],int n) that will find the largest int in the n ints in a.
This should be the only function in the file.
Generate assembly language for this code using -S.
Identify the variables in this code, defining a table in comments in the code.
Comment each line of code, indicating what it is doing in terms of the original C code.
2) Before you move on, make a copy of your .s file.
3) Generate optimized code for the same C code. You do this with the -O (-Os if you are on MacOS) switch to gcc
For example,
gcc -S -O largest.c
will generate largest.s (clobbering your first version if you did not save it elsewhere!)
Build the same variable table and code comments for this version.
Submit largest.c and both copies of largest.s with your comments.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6d9e051c-fa16-49f1-928d-4e8269db7ea0%2F33c24c7a-af37-4eb3-b13d-67736bd3eb6e%2Fyljyxpb_processed.png&w=3840&q=75)

Step by step
Solved in 7 steps with 4 images

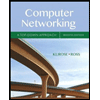
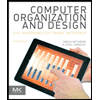
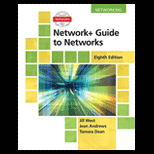
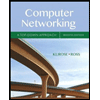
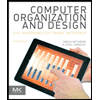
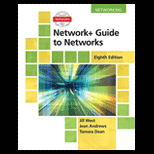
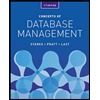
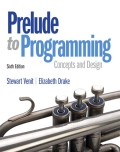
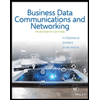