1) Write a program that reads information about employees of a company from a file. The information in the file is ordered based on the salary. An employee with the highest salary, for example, should appear at the top. Your program should first read the contents of the input file, create a linked list to store the contents, and then display the original list. Then, it will delete the employee with ID 444444444 from the list and will display the list again. The last step your program perform is to insert three new employees into the list and display the final results. Here is the information on the three new employees. Last Name First Name ID Phone Salary Wood Carpenter Iron 333556666 444444443 828-888-8888 73000 777777777 888-666-8888 86000 222-222-2222 40000 Ironman Goal-keeper Martin Here is the input file. Doe Doe Hardworker John Jan John 5555555 444 888888888 555446666 333-333-3333 65000 333-333-3333 75000 888-888-8888 5000 666-666-6666 76000 Programmer Travis Output: Original List Last Name First Name ID Phone Salary 555555s 4444 333-333-3333 85000 333-333-3333 76000 888-888-8888 75000 666-666-6666 45000 Doe Doe John Jan Hardworker Programmer John 888888888 Travis 555446666 List after removal of employee 444444444: First Name Last Name ID Phone Salary Doe Hardworker Ironman John John Iron 5 885 444444443 333-333-3333 85000 888-888-8888 75000 828-888-8888 73000 Programmer Travis 555446666 666-666-6666 45000 Final Output: Last Name First Name ID Phone Salary Goal-keeper Doe Hardworker Ironman Programmer Wood Martin John John 777ררךררר 55 88 444444443 555446666 888-666-8888 86000 333-333-3333 85000 888-888-8888 75000 828-888-8888 666-666-6666 45000 222-222-2222 Iron 73000 Travis Сamenter 333556666 40000
Can someone help about my assignment?
Instruction is on image:
Given:
Last Name First Name ID Phone Salary
=========== ========== ======== ======= =====
Wood Carpenter 333556666 222-222-2222 40000
Ironman Iron 444444443 828-888-8888 73000
Goal-keeper Martin 777777777 888-666-8888 86000
Here is the input file.
Doe John 555555555 333-333-3333 65000
Doe Jan 444444444 333-333-3333 75000
Hardworker John 888888888 888-888-8888 55000
Programmer Travis 555446666 666-666-6666 76000
Output:
Original List
Last Name First Name ID Phone Salary
=========== ========== ======== ======= =====
Doe John 555555555 333-333-3333 85000
Doe Jan 444444444 333-333-3333 76000
Hardworker John 888888888 888-888-8888 75000
Programmer Travis 555446666 666-666-6666 45000
List after removal of employee 444444444:
Last Name First Name ID Phone Salary
=========== ========== ======== ======= =====
Doe John 555555555 333-333-3333 85000
Hardworker John 888888888 888-888-8888 75000
Ironman Iron 444444443 828-888-8888 73000
Programmer Travis 555446666 666-666-6666 45000
Final Output:
Last Name First Name ID Phone Salary
=========== ========== ======== ======= =====
Goal-keeper Martin 777777777 888-666-8888 86000
Doe John 555555555 333-333-3333 85000
Hardworker John 888888888 888-888-8888 75000
Ironman Iron 444444443 828-888-8888 73000
Programmer Travis 555446666 666-666-6666 45000
Wood Carpenter 333556666 222-222-2222 40000
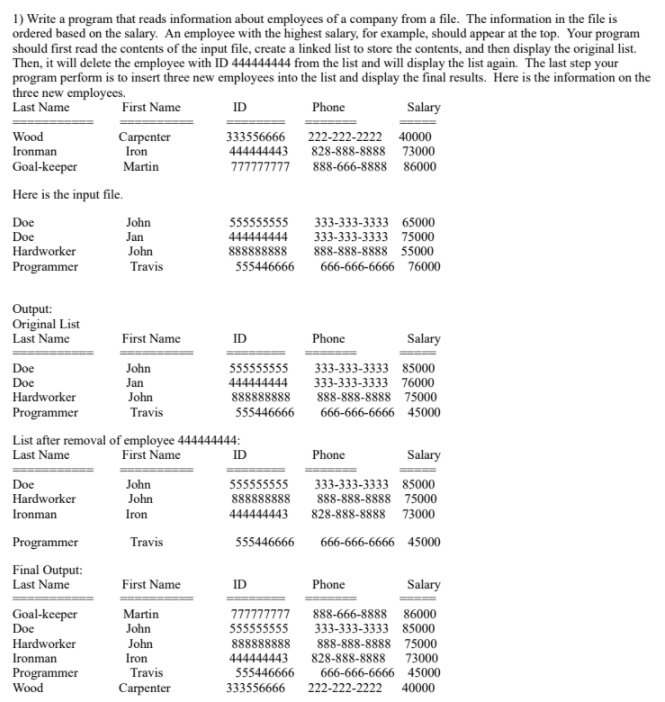

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 6 images
