1) Write a static method named computeOddSum that is passed an array of int's named numbers. The method must compute and return the sum of all the values in numbers that are odd. For example, if numbers is {3, 10, 11, 2, 6, 9, 5} then the value 28 should be returned since 3 + 11 + 9 + 5 = 28 is the sum of all the odd values in numbers. 2) Write a static method named replaceEvens that receives the parameter numbers which is an array of int's. The method must replace all elements of numbers that store even values with the negative of that value. That is, if the number 12 is found in a given position of numbers, then the method must replace the 12 with a -12. You can assume that all values in the array are positive numbers greater than zero. 3) Write a static, void method named replaceAll that accepts an array of int's named numbers as well as an int named num. The method must replace all occurrences of num that are found in even-numbered index positions with the value of 3. That is, if the value num is stored in numbers[4] then it must be replaced with the value 3 since 4 is an even number. But if num is found in numbers[5] , it must not be replaced since 5 is not an even number. For this exercise, zero is considered to be an even number. The method must work for an array of any size. 4) There is a non-empty array of String's named names. Write a code segment that removes the last letter of the String stored in the very last position of names. For bragging rights and if possible (and I'm not sure if it is), write a single statement that performs this task.
1) Write a static method named computeOddSum that is passed an array of int's named numbers. The method must compute and return the sum of all the values in numbers that are odd. For example, if numbers is {3, 10, 11, 2, 6, 9, 5} then the value 28 should be returned since 3 + 11 + 9 + 5 = 28 is the sum of all the odd values in numbers. 2) Write a static method named replaceEvens that receives the parameter numbers which is an array of int's. The method must replace all elements of numbers that store even values with the negative of that value. That is, if the number 12 is found in a given position of numbers, then the method must replace the 12 with a -12. You can assume that all values in the array are positive numbers greater than zero. 3) Write a static, void method named replaceAll that accepts an array of int's named numbers as well as an int named num. The method must replace all occurrences of num that are found in even-numbered index positions with the value of 3. That is, if the value num is stored in numbers[4] then it must be replaced with the value 3 since 4 is an even number. But if num is found in numbers[5] , it must not be replaced since 5 is not an even number. For this exercise, zero is considered to be an even number. The method must work for an array of any size. 4) There is a non-empty array of String's named names. Write a code segment that removes the last letter of the String stored in the very last position of names. For bragging rights and if possible (and I'm not sure if it is), write a single statement that performs this task.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Write a Java computer
![An array is a consecutive storage for multiple items of the same type. You can store a value in an
array using an index (location in the array). You can get a value from an array using an index.
An array is like a row of lockers, except that you can't cram lots of stuff into it. You can only store
one value at each array index. An array index is like a locker number. It helps you find a particular
place to store your stuff and retrieve stuff.
There are several ways to declare and create a one-dimensional array. An array named numbers
is being instantiated in the following statements:
int[] numbers = new int[5];
and
int[] numbers = {11, 12, -33, 54, 5};
Unless initialized, the values in an array of int's or double's are automatically initialized to zero.
The value stored in an index position of an array is called an element. The first element of an
array is considered to be stored in index position zero (aka subscript position zero).
To assign or change a value stored in the array, you can use an assignment statement like this
numbers [0] = 99; //which stores the value 99 in index position 0.
To display an element in an array, you can use the square brackets as in
System.out.println(numbers[0]); //to make the value 99 appear.
The length property of an array can be used as in
int[] scores =
{88, 77, 62, 98, 70};
System.out.println(scores.length);
which would printout the length of the array named scores which is 5 even though the value 70
is stored in index position 4.
Note that length is a public property and not a method. Therefore, parentheses must not be used.
Do not confuse this public property with the public method that is available in the String class.
Note the difference in the following statements:
int[] scores
{88, 77, 62, 98, 70};
System.out.println("The length of this array is
+ scores.length);
%3D
and
String name
= "Bob";
System.out.println("The length of this string is "
name.length());
+
Once an array has been instantiated, its length can't be changed. You can expand an array
indirectly though by creating a bigger array and then copying the contents of the original array
into the bigger array.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3046cdee-db5c-45b8-83b1-15e9b585ff09%2F997c6a2c-5163-4fa6-929a-825f95612b32%2F56mck4s_processed.png&w=3840&q=75)
Transcribed Image Text:An array is a consecutive storage for multiple items of the same type. You can store a value in an
array using an index (location in the array). You can get a value from an array using an index.
An array is like a row of lockers, except that you can't cram lots of stuff into it. You can only store
one value at each array index. An array index is like a locker number. It helps you find a particular
place to store your stuff and retrieve stuff.
There are several ways to declare and create a one-dimensional array. An array named numbers
is being instantiated in the following statements:
int[] numbers = new int[5];
and
int[] numbers = {11, 12, -33, 54, 5};
Unless initialized, the values in an array of int's or double's are automatically initialized to zero.
The value stored in an index position of an array is called an element. The first element of an
array is considered to be stored in index position zero (aka subscript position zero).
To assign or change a value stored in the array, you can use an assignment statement like this
numbers [0] = 99; //which stores the value 99 in index position 0.
To display an element in an array, you can use the square brackets as in
System.out.println(numbers[0]); //to make the value 99 appear.
The length property of an array can be used as in
int[] scores =
{88, 77, 62, 98, 70};
System.out.println(scores.length);
which would printout the length of the array named scores which is 5 even though the value 70
is stored in index position 4.
Note that length is a public property and not a method. Therefore, parentheses must not be used.
Do not confuse this public property with the public method that is available in the String class.
Note the difference in the following statements:
int[] scores
{88, 77, 62, 98, 70};
System.out.println("The length of this array is
+ scores.length);
%3D
and
String name
= "Bob";
System.out.println("The length of this string is "
name.length());
+
Once an array has been instantiated, its length can't be changed. You can expand an array
indirectly though by creating a bigger array and then copying the contents of the original array
into the bigger array.
![Deliverables:
1) Write a static method named computeOddSum that is passed an array of int's named
numbers. The method must compute and return the sum of all the values in numbers that
are odd. For example, if numbers is {3, 10, 11, 2, 6, 9, 5} then the value 28 should be
returned since 3 + 11 + 9 + 5 = 28 is the sum of all the odd values in numbers.
2) Write a static method named replaceEvens that receives the parameter numbers which
is an array of int's. The method must replace all elements of numbers that store even
values with the negative of that value. That is, if the number 12 is found in a given position
of numbers, then the method must replace the 12 with a -12. You can assume that all
values in the array are positive numbers greater than zero.
3) Write a static, void method named replaceAll that accepts an array of int's named
numbers as well as an int named num. The method must replace all occurrences of num
that are found in even-numbered index positions with the value of 3. That is, if the value
num is stored in numbers[4] then it must be replaced with the value 3 since 4 is an even
number. But if num is found in numbers[5] , it must not be replaced since 5 is not an even
number. For this exercise, zero is considered to be an even number. The method must
work for an array of any size.
4) There is a non-empty array of String's named names. Write a code segment that removes
the last letter of the String stored in the very last position of names. For bragging rights
and if possible (and l'm not sure if it is), write a single statement that performs this task.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3046cdee-db5c-45b8-83b1-15e9b585ff09%2F997c6a2c-5163-4fa6-929a-825f95612b32%2Fmd6dloj_processed.png&w=3840&q=75)
Transcribed Image Text:Deliverables:
1) Write a static method named computeOddSum that is passed an array of int's named
numbers. The method must compute and return the sum of all the values in numbers that
are odd. For example, if numbers is {3, 10, 11, 2, 6, 9, 5} then the value 28 should be
returned since 3 + 11 + 9 + 5 = 28 is the sum of all the odd values in numbers.
2) Write a static method named replaceEvens that receives the parameter numbers which
is an array of int's. The method must replace all elements of numbers that store even
values with the negative of that value. That is, if the number 12 is found in a given position
of numbers, then the method must replace the 12 with a -12. You can assume that all
values in the array are positive numbers greater than zero.
3) Write a static, void method named replaceAll that accepts an array of int's named
numbers as well as an int named num. The method must replace all occurrences of num
that are found in even-numbered index positions with the value of 3. That is, if the value
num is stored in numbers[4] then it must be replaced with the value 3 since 4 is an even
number. But if num is found in numbers[5] , it must not be replaced since 5 is not an even
number. For this exercise, zero is considered to be an even number. The method must
work for an array of any size.
4) There is a non-empty array of String's named names. Write a code segment that removes
the last letter of the String stored in the very last position of names. For bragging rights
and if possible (and l'm not sure if it is), write a single statement that performs this task.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 8 steps with 11 images

Recommended textbooks for you
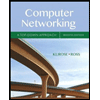
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
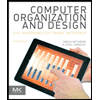
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
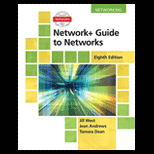
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
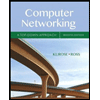
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
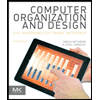
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
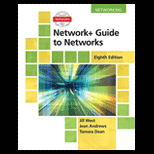
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
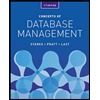
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
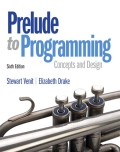
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
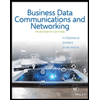
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY