1: Write code to declare a class named kittyCat that contains the following data members: name, color, yearOfBirth, and ownerName. There should be accessors and mutators for each of these data members. Define all ten of the member functions. 2: Write a main () that makes an array of five kittyCat objects named pets. Prompt the user for the four data members for each kittyCat object, and set them using the appropriate mutator. When this is done, neatly print out the information about each kittyCat using the appropriate accessor. t 3: Sometimes it's easiest to work with objects when you don't have to build them from scratch. Let's write a copy constructor so that we can copy existing kittyCat objects when declaring a new one. Here's the declaration that you should put in your class declaration: kittyCat (const kittyCat& oldCat); // Copy constructor The idea is that we'll use the oldCat parameter to give the four member variables their initial values. The definition of this constructor should be short: just four lines of code! Once it's written, you can test the code in your main (). Assuming that you still have the array from Assignment 2 above, you can copy one of those objects into the new kittyCat object by declaring a new variable below like this: kittyCat myNewCat (pets[0]); // Declaring a new kittyCat Now print out the stuff in myNewCat neatly (as you did before). Does it match what was in the first element of pets?
1: Write code to declare a class named kittyCat that contains the following data members: name, color, yearOfBirth, and ownerName. There should be accessors and mutators for each of these data members. Define all ten of the member functions. 2: Write a main () that makes an array of five kittyCat objects named pets. Prompt the user for the four data members for each kittyCat object, and set them using the appropriate mutator. When this is done, neatly print out the information about each kittyCat using the appropriate accessor. t 3: Sometimes it's easiest to work with objects when you don't have to build them from scratch. Let's write a copy constructor so that we can copy existing kittyCat objects when declaring a new one. Here's the declaration that you should put in your class declaration: kittyCat (const kittyCat& oldCat); // Copy constructor The idea is that we'll use the oldCat parameter to give the four member variables their initial values. The definition of this constructor should be short: just four lines of code! Once it's written, you can test the code in your main (). Assuming that you still have the array from Assignment 2 above, you can copy one of those objects into the new kittyCat object by declaring a new variable below like this: kittyCat myNewCat (pets[0]); // Declaring a new kittyCat Now print out the stuff in myNewCat neatly (as you did before). Does it match what was in the first element of pets?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
For this code I'm suppose to use a .h file a cpp. file and a main function. how to I do that?
![1:
Write code to declare a class named kittyCat that contains the following data
members: name, color, yearOfBirth, and ownerName. There should be accessors and
mutators for each of these data members. Define all ten of the member functions.
2:
Write a main () that makes an array of five kittyCat objects named pets. Prompt the
user for the four data members for each kittyCat object, and set them using the
appropriate mutator. When this is done, neatly print out the information about each
kittyCat using the appropriate accessor.
t 3:
Sometimes it's easiest to work with objects when you don't have to build them from
scratch. Let's write a copy constructor so that we can copy existing kittyCat objects
when declaring a new one. Here's the declaration that you should put in your class
declaration:
kittyCat (const kittyCat& oldCat); // Copy constructor
The idea is that we'll use the oldCat parameter to give the four member variables their
initial values. The definition of this constructor should be short: just four lines of code!
Once it's written, you can test the code in your main (). Assuming that you still have the
array from Assignment 2 above, you can copy one of those objects into the new kittyCat
object by declaring a new variable below like this:
kittyCat myNewCat (pets[0]); // Declaring a new kittyCat
Now print out the stuff in myNewCat neatly (as you did before). Does it match what was
in the first element of pets?](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2a4399a9-5724-42c8-89a0-9bc27dd1a0f2%2F209be289-8d71-46d2-a9dd-2e02686bc6c0%2F8cwcsik_processed.png&w=3840&q=75)
Transcribed Image Text:1:
Write code to declare a class named kittyCat that contains the following data
members: name, color, yearOfBirth, and ownerName. There should be accessors and
mutators for each of these data members. Define all ten of the member functions.
2:
Write a main () that makes an array of five kittyCat objects named pets. Prompt the
user for the four data members for each kittyCat object, and set them using the
appropriate mutator. When this is done, neatly print out the information about each
kittyCat using the appropriate accessor.
t 3:
Sometimes it's easiest to work with objects when you don't have to build them from
scratch. Let's write a copy constructor so that we can copy existing kittyCat objects
when declaring a new one. Here's the declaration that you should put in your class
declaration:
kittyCat (const kittyCat& oldCat); // Copy constructor
The idea is that we'll use the oldCat parameter to give the four member variables their
initial values. The definition of this constructor should be short: just four lines of code!
Once it's written, you can test the code in your main (). Assuming that you still have the
array from Assignment 2 above, you can copy one of those objects into the new kittyCat
object by declaring a new variable below like this:
kittyCat myNewCat (pets[0]); // Declaring a new kittyCat
Now print out the stuff in myNewCat neatly (as you did before). Does it match what was
in the first element of pets?

Transcribed Image Text:Assign
Suppose, though, that we don't have a whole object to copy, but we want to set some
initial values when we declare the object. We'll use a constructor with one parameter for
each of the four member variables:
kittyCat (/* a list of four data member types */);
The list of parameters will depend on what you chose for the data types of your data
members. This parameterized constructor might be used in main () like this:
kittyCat myOtherCat ("Tiger", "orange", 2015, "Mary");
5:
The constructor in Assignment 4 currently requires you to enter all of the parameters.
Modify it to have defaults for color, yearOfBirth, and ownerName. This will let you do
the following:
kittyCat myThirdCat ("Cleo"); // The other members get defaults
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 8 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
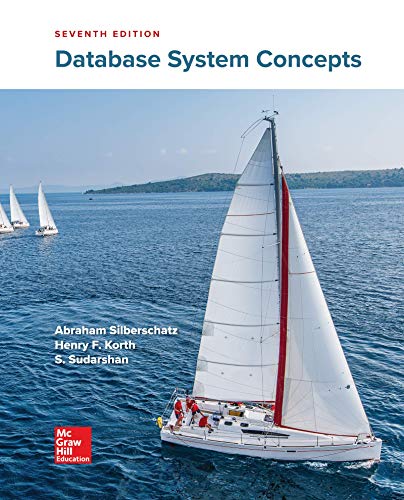
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
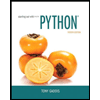
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
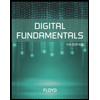
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
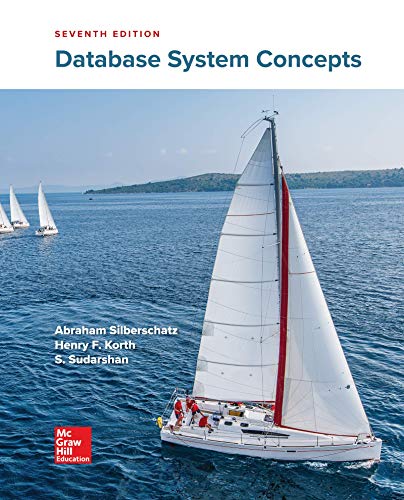
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
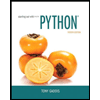
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
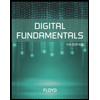
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
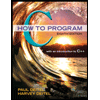
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
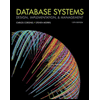
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
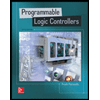
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education