1. Create a simple game that will allow user to guess the secret number. 2. Create a folder named LastName_FirstName (ex. Reyes_Mark) in your local drive. 3. Create a new project named LabExer7A. Set the project location to your own folder. 4. Add another class named RunNumberFinder. 5. For the LabExer7A class, instantiate an object of NumberFinder to create a JFrame in the main method. Set the object’s width to 600 and height to 200. Make sure the JFrame is visible. 6. For the LabExer7A class, perform the following: 6.1 Construct import statements to use the packages listed below: • javax.swing • java.awt • java.awt.event • java.util 6.2 Extend the JFrame class and implement the MouseListener interface. (The MouseListener interface is for receiving mouse events such as click and press on a component.) 6.3 Create the following objects/variables outside all methods (just below the class declaration): • One (1) FlowLayout object • Two (2) Font objects: For the first object, set the typeface to Arial, style to Bold, and point size to 100. For the second, set the typeface to Century, style to Bold, and point size to 20. Syntax: Font nameOfFontObj = new Font(“Typeface”, Font.STYLE, point size); • 10 JLabel objects: Nine (9) are for displaying numbers 1-9 while the tenth JLabel is for displaying the result (initially set to blank text). Syntax: JLabel nameOfJLabelObj = new JLabel(“1”); • Random object • Two (2) integers: One is for accepting the value of the random number from 1-9 while the other is for counting the number of guess attempts. Note: Additional objects/variables may be created. 6.4 Follow the instructions below for the content of the LabExer7A constructor. • Place the statement super(“Number Finder”); • Set the Java program to end when the Close or X button is clicked. • Set the previously created FlowLayout object as the layout. Syntax: setLayout(nameOfFlowLayoutObj); • Set the first Font object as the font setting of the nine (9) JLabel objects. Set the second Font object as the font setting of the tenth JLabel object. Ex. nameOfJLabelObj.setFont(nameOfFontObj); • Add the JLabel objects to the JFrame. Ex. add(nameOfJLabelObj); • Set the nine (9) JLabel objects as mouse listeners. Ex. nameOfJLabelObj.addMouseListener(this); 6.5 Construct the five (5) required methods of the MouseListener interface. The program will not execute if these methods are not complete. • mouseClicked • mousePressed • mouseReleased • mouseEntered • mouseExited Ex. public void mouseClicked(MouseEvent e) { } The program shall: • allow the user to click a number until the secret number is found • display a JLabel (the tenth JLabel created earlier) to indicate the result of the guess. Show “Try again.” if the guess is wrong. Otherwise, show “Correct!” and the number of guess attempts. 6.6 In the mouseClicked method, create multiple if-else statements to accomplish the program flow. See example below. if (e.getSource()==nameOfLabelObj) { //events that will occur when the JLabel object is clicked } Here are some of the methods which you may find useful: • getText() – to retrieve the current text of the JLabel Syntax: nameOfJLabelObj.getText().equals(nameOfAnother); • setEnabled() – to enable or disable the JLabel Syntax: nameOfJLabelObj.setEnabled(booleanValue); • toString() – to convert an integer to String Syntax: Integer.toString(nameOfIntVar);
1. Create a simple game that will allow user to guess the secret number.
2. Create a folder named LastName_FirstName (ex. Reyes_Mark) in your local drive.
3. Create a new project named LabExer7A. Set the project location to your own folder.
4. Add another class named RunNumberFinder.
5. For the LabExer7A class, instantiate an object of NumberFinder to create a JFrame in the main
method. Set the object’s width to 600 and height to 200. Make sure the JFrame is visible.
6. For the LabExer7A class, perform the following:
6.1 Construct import statements to use the packages listed below:
• javax.swing
• java.awt
• java.awt.event
• java.util
6.2 Extend the JFrame class and implement the MouseListener interface. (The MouseListener
interface is for receiving mouse events such as click and press on a component.)
6.3 Create the following objects/variables outside all methods (just below the class declaration):
• One (1) FlowLayout object
• Two (2) Font objects: For the first object, set the typeface to Arial, style to Bold, and point
size to 100. For the second, set the typeface to Century, style to Bold, and point size to 20.
Syntax: Font nameOfFontObj = new Font(“Typeface”, Font.STYLE, point size);
• 10 JLabel objects: Nine (9) are for displaying numbers 1-9 while the tenth JLabel is for
displaying the result (initially set to blank text). Syntax: JLabel nameOfJLabelObj = new
JLabel(“1”);
• Random object
• Two (2) integers: One is for accepting the value of the random number from 1-9 while the
other is for counting the number of guess attempts.
Note: Additional objects/variables may be created.
6.4 Follow the instructions below for the content of the LabExer7A constructor.
• Place the statement super(“Number Finder”);
• Set the Java program to end when the Close or X button is clicked.
• Set the previously created FlowLayout object as the layout. Syntax:
setLayout(nameOfFlowLayoutObj);
• Set the first Font object as the font setting of the nine (9) JLabel objects. Set the second
Font object as the font setting of the tenth JLabel object. Ex.
nameOfJLabelObj.setFont(nameOfFontObj);
• Add the JLabel objects to the JFrame. Ex. add(nameOfJLabelObj);
• Set the nine (9) JLabel objects as mouse listeners. Ex.
nameOfJLabelObj.addMouseListener(this);
6.5 Construct the five (5) required methods of the MouseListener interface. The program will not
execute if these methods are not complete.
• mouseClicked
• mousePressed
• mouseReleased
• mouseEntered
• mouseExited
Ex. public void mouseClicked(MouseEvent e) { }
The program shall:
• allow the user to click a number until the secret number is found
• display a JLabel (the tenth JLabel created earlier) to indicate the result of the guess.
Show “Try again.” if the guess is wrong. Otherwise, show “Correct!” and the number
of guess attempts.
6.6 In the mouseClicked method, create multiple if-else statements to accomplish the program flow.
See example below.
if (e.getSource()==nameOfLabelObj)
{
//events that will occur when the JLabel object is clicked
}
Here are some of the methods which you may find useful:
• getText() – to retrieve the current text of the JLabel
Syntax: nameOfJLabelObj.getText().equals(nameOfAnother);
• setEnabled() – to enable or disable the JLabel
Syntax: nameOfJLabelObj.setEnabled(booleanValue);
• toString() – to convert an integer to String
Syntax: Integer.toString(nameOfIntVar);
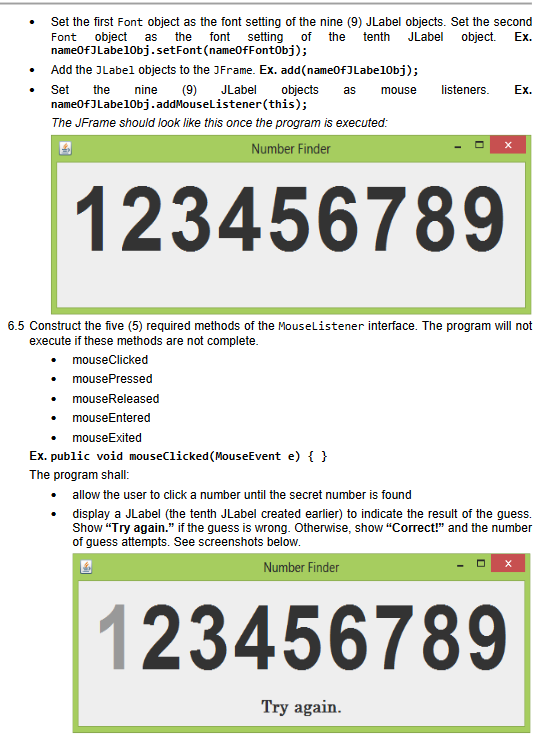
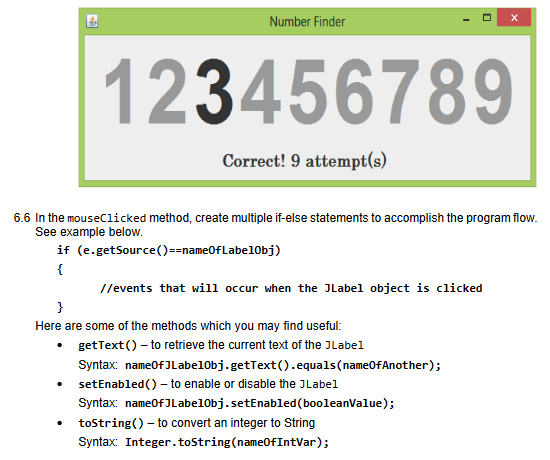

Step by step
Solved in 2 steps with 1 images

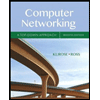
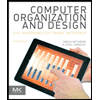
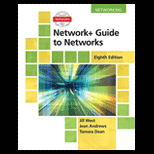
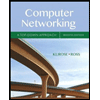
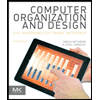
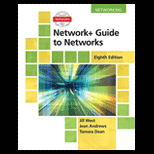
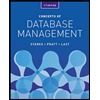
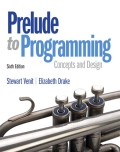
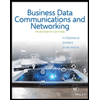