1. Create an Employee class and do the following: a) Declare the following member variables: Employee Id, Employee Name, and Salary b) Create a default constructor with no parameter. c) Create a parameterized constructor to initialize all the member variables. d) Create a method to display all the member variables. e) Create a method that will compute the Total Salary of the Employee and return it. The method will accept one parameter Bonus of type double. The Total Salary is computed as Salary + Bonus. f) Generate the getters and setters.
1. Create an Employee class and do the following: a) Declare the following member variables: Employee Id, Employee Name, and Salary b) Create a default constructor with no parameter. c) Create a parameterized constructor to initialize all the member variables. d) Create a method to display all the member variables. e) Create a method that will compute the Total Salary of the Employee and return it. The method will accept one parameter Bonus of type double. The Total Salary is computed as Salary + Bonus. f) Generate the getters and setters.
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 9RQ
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
basic java only
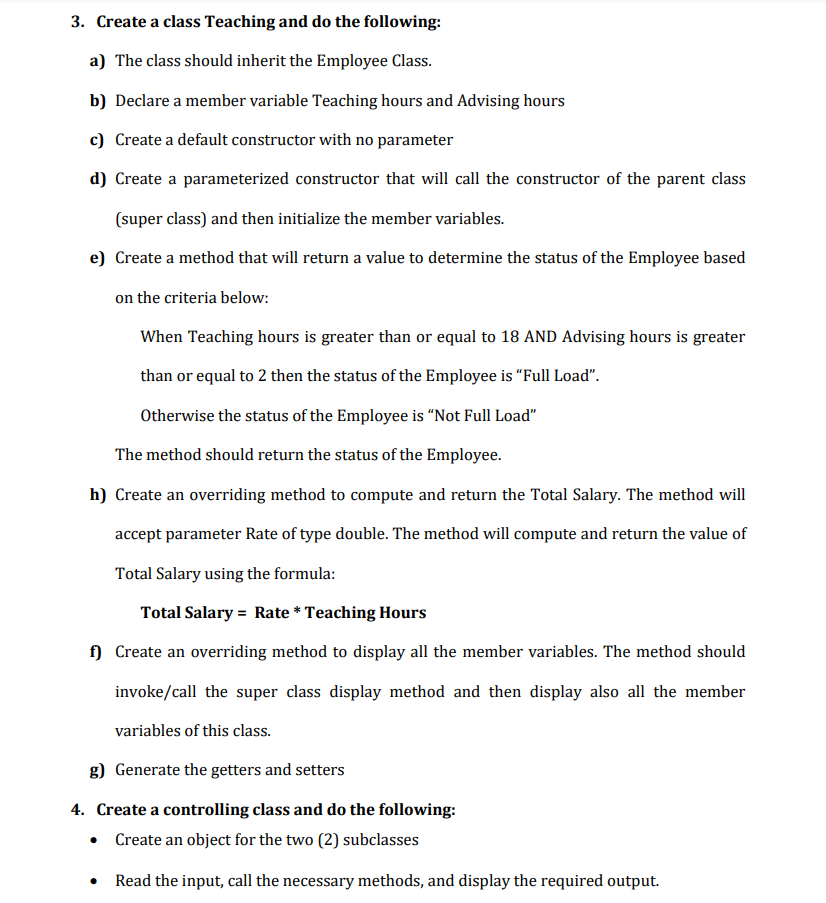
Transcribed Image Text:3. Create a class Teaching and do the following:
a) The class should inherit the Employee Class.
b) Declare a member variable Teaching hours and Advising hours
c) Create a default constructor with no parameter
d) Create a parameterized constructor that will call the constructor of the parent class
(super class) and then initialize the member variables.
e) Create a method that will return a value to determine the status of the Employee based
on the criteria below:
When Teaching hours is greater than or equal to 18 AND Advising hours is greater
than or equal to 2 then the status of the Employee is "Full Load".
Otherwise the status of the Employee is "Not Full Load"
The method should return the status of the Employee.
h) Create an overriding method to compute and return the Total Salary. The method will
accept parameter Rate of type double. The method will compute and return the value of
Total Salary using the formula:
Total Salary Rate * Teaching Hours
f) Create an overriding method to display all the member variables. The method should
invoke/call the super class display method and then display also all the member
variables of this class.
g) Generate the getters and setters
4. Create a controlling class and do the following:
• Create an object for the two (2) subclasses
Read the input, call the necessary methods, and display the required output.
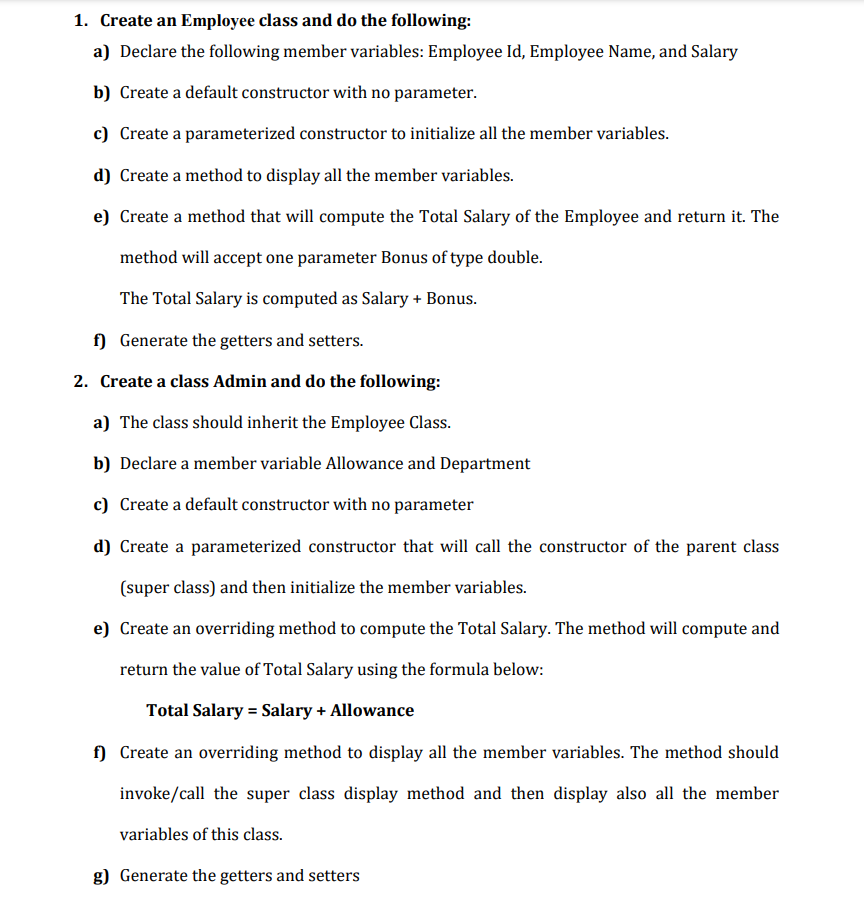
Transcribed Image Text:1. Create an Employee class and do the following:
a) Declare the following member variables: Employee Id, Employee Name, and Salary
b) Create a default constructor with no parameter.
c) Create a parameterized constructor to initialize all the member variables.
d) Create a method to display all the member variables.
e) Create a method that will compute the Total Salary of the Employee and return it. The
method will accept one parameter Bonus of type double.
The Total Salary is computed as Salary + Bonus.
f)
Generate the getters and setters.
2. Create a class Admin and do the following:
a) The class should inherit the Employee Class.
b) Declare a member variable Allowance and Department
c) Create a default constructor with no parameter
d) Create a parameterized constructor that will call the constructor of the parent class
(super class) and then initialize the member variables.
e) Create an overriding method to compute the Total Salary. The method will compute and
return the value of Total Salary using the formula below:
Total Salary = Salary + Allowance
f) Create an overriding method to display all the member variables. The method should
invoke/call the super class display method and then display also all the member
variables of this class.
g) Generate the getters and setters
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
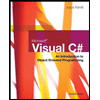
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
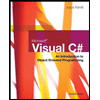
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,