1. Design a class, CipherDriver, that uses a repeating key to encode and decode a message read from the user. 2. Assume that the person that encrypts a message has the same key as the one that decodes it. 3. The key is generated randomly with integers in a range that contains both positive and negative numbers, such as -10 to 10. 4. Consider a key set with 7 values. 5. Given that Java uses Unicode, do not wrap the characters around when you reach the end of the alphabet. 6. To encode, use the list of keys to shift the characters to the right. 7. The encode operation shifts characters to the right with a value taken from the key set. 8. To decode an encoded message, the characters from the encoded text are shifted to the left with the same key set. 9. Define and instantiate two queues, one for encode and one for decode operations, filled with the same set of numbers. 10. Use the link-based implementation for the two queues. 11. Read a message from the user and encode it. 12. Take the encoded message and decode it. 13. As a demonstration, display the key set, the original message, the encoded one, and the decoded one. 14. If you implement all the required methods properly, the driver program should generate outputs similar to the following: keySet = {-6,1,-2,4,-6,8,1,} Enter a message to be encoded: Today is a good day for a Java problem! Original message: Today is a good day for a Java problem! Encoded Message: Npbes(jm!_$awp^!bes(gis#eERbpb@tlwcffk% Decoded Message: Today is a good day for a Java problem! Press any key to continue . .
1. Design a class, CipherDriver, that uses a repeating key to encode and decode a message read from the user. 2. Assume that the person that encrypts a message has the same key as the one that decodes it. 3. The key is generated randomly with integers in a range that contains both positive and negative numbers, such as -10 to 10. 4. Consider a key set with 7 values. 5. Given that Java uses Unicode, do not wrap the characters around when you reach the end of the alphabet. 6. To encode, use the list of keys to shift the characters to the right. 7. The encode operation shifts characters to the right with a value taken from the key set. 8. To decode an encoded message, the characters from the encoded text are shifted to the left with the same key set. 9. Define and instantiate two queues, one for encode and one for decode operations, filled with the same set of numbers. 10. Use the link-based implementation for the two queues. 11. Read a message from the user and encode it. 12. Take the encoded message and decode it. 13. As a demonstration, display the key set, the original message, the encoded one, and the decoded one. 14. If you implement all the required methods properly, the driver program should generate outputs similar to the following: keySet = {-6,1,-2,4,-6,8,1,} Enter a message to be encoded: Today is a good day for a Java problem! Original message: Today is a good day for a Java problem! Encoded Message: Npbes(jm!_$awp^!bes(gis#eERbpb@tlwcffk% Decoded Message: Today is a good day for a Java problem! Press any key to continue . .
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
In Java Please
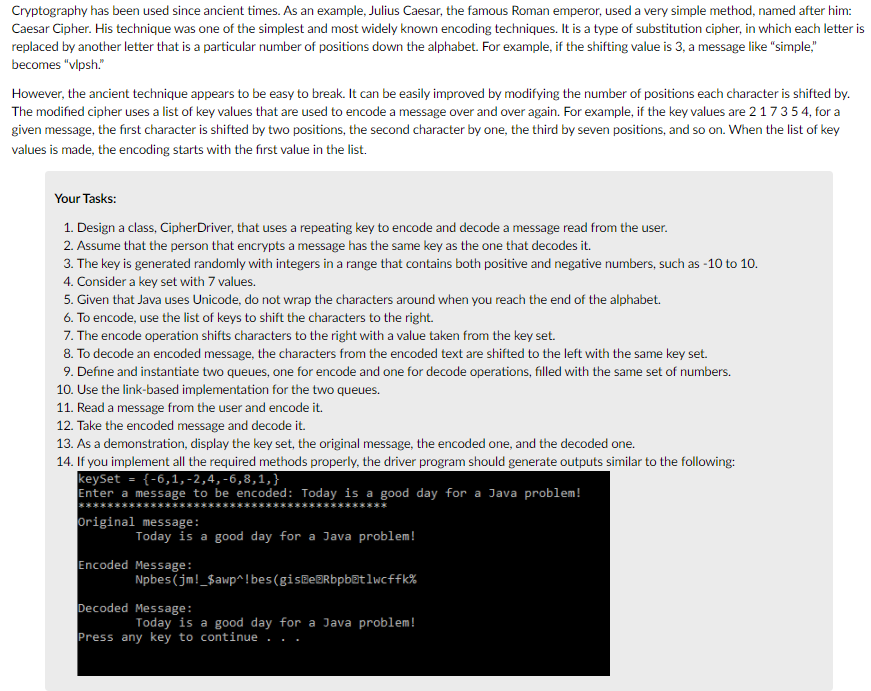
Transcribed Image Text:Cryptography has been used since ancient times. As an example, Julius Caesar, the famous Roman emperor, used a very simple method, named after him:
Caesar Cipher. His technique was one of the simplest and most widely known encoding techniques. It is a type of substitution cipher, in which each letter is
replaced by another letter that is a particular number of positions down the alphabet. For example, if the shifting value is 3, a message like "simple,"
becomes "vlpsh."
However, the ancient technique appears to be easy to break. It can be easily improved by modifying the number of positions each character is shifted by.
The modified cipher uses a list of key values that are used to encode a message over and over again. For example, if the key values are 217354, for a
given message, the first character is shifted by two positions, the second character by one, the third by seven positions, and so on. When the list of key
values is made, the encoding starts with the first value in the list.
Your Tasks:
1. Design a class, CipherDriver, that uses a repeating key to encode and decode a message read from the user.
2. Assume that the person that encrypts a message has the same key as the one that decodes it.
3. The key is generated randomly with integers in a range that contains both positive and negative numbers, such as -10 to 10.
4. Consider a key set with 7 values.
5. Given that Java uses Unicode, do not wrap the characters around when you reach the end of the alphabet.
6. To encode, use the list of keys to shift the characters to the right.
7. The encode operation shifts characters to the right with a value taken from the key set.
8. To decode an encoded message, the characters from the encoded text are shifted to the left with the same key set.
9. Define and instantiate two queues, one for encode and one for decode operations, filled with the same set of numbers.
10. Use the link-based implementation for the two queues.
11. Read a message from the user and encode it.
12. Take the encoded message and decode it.
13. As a demonstration, display the key set, the original message, the encoded one, and the decoded one.
14. If you implement all the required methods properly, the driver program should generate outputs similar to the following:
keySet = {-6,1,-2,4,-6,8,1,}
Enter a message to be encoded: Today is a good day for a Java problem!
******
*******
Original message:
Today is a good day for a Java problem!
Encoded Message:
Npbes(jm!_$awp^!bes(gisBeBRbpbētlwcffk%
Decoded Message:
Today is a good day for a Java problem!
Press any key to continue . .
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
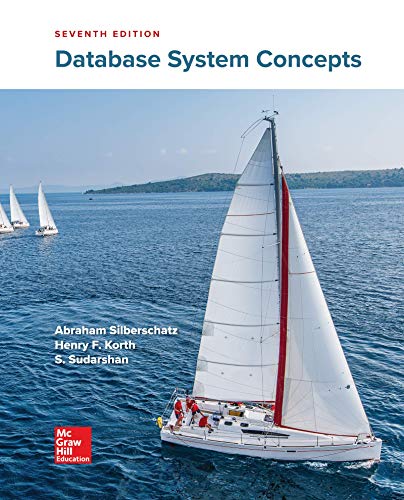
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
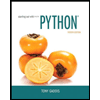
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
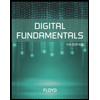
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
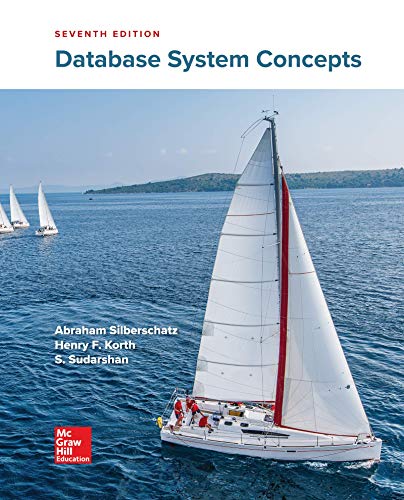
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
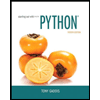
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
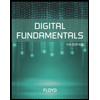
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
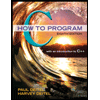
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
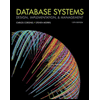
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
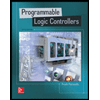
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education