1. Download ComputeMeanDeviation.java from M5 Methods module in folio. 2. Modify the program by adding the following two methods: public static double mean (int boundary, int n) public static double deviation (int boundary, int n) In the mean method, generate n random integers = [0, boundary], compute and return the result of the mean. In the deviation method, generate n random integers = [0, boundary], compute and return the result of the standard deviation. The formulas to compute the mean and the standard deviation are: i-1 mean deviation = n-1 3. Rewrite the main method so that it invokes the mean and deviation methods and displays the results of the mean and the standard deviation. A sample main method is as follows. Please type this main method in your own source code. public static void main(String[] args) { // Find mean double meanValue = mean (99, 1000); // Find standard deviation double deviationValue = deviation (99, 1000); // Display result "1 System.out.println("The mean is + meanValue); System.out.println ("The standard deviation is deviationValue); }| n [x²- WE n
I need help with an assignment. I am confused as to if the manual is asking to implement and modify the java code provided to us or create one following the code written in the java file provided. So please help me figure that out and provide with a sultion. Rest of the instruction manual is attatched in the images. I am pasting the java code provided to me below.
java code provided to me as mentioned in step 1 of manual.
package homework;
// ComputeMeanDeviation.java: Demonstrate using the math methods
public class ComputeMeanDeviation {
/** Main method */
public static void main(String[] args) {
int count = 10; // Total numbers
int number = 0; // Store a random number
double sum = 0; // Store the sum of the numbers
double squareSum = 0; // Store the sum of the squares
// Create numbers, find its sum, and its square sum
for (int i = 0; i < count; i++) {
// Generate a new random number
number = (int)(Math.random() * 1000);
// Add the number to sum
sum += number;
// Add the square of the number to squareSum
squareSum += Math.pow(number, 2); // Same as number*number;
}
// Find mean
double mean = sum / count;
// Find standard deviation
double deviation =
Math.sqrt((squareSum - sum * sum / count) / (count - 1));
// Display result
System.out.println("The standard deviation is " + deviation);
}
}
![But, before you submit your java file to Gradescope, please insert these three lines given
below at the beginning of your code.
package homework;
import brandon.math. Random;
import brandon.math.Math;
An illustration in the next page is done for your help.
package homework;
import brandon.math. Random;
import brandon.math.Math;
public class HW4 {
public static void main(String[] args) {
// Find mean
double meanValue = mean (99, 1000);
// Find standard deviation
double deviationValue = deviation (99, 1000);
// Display result
System.out.println("The mean is " + meanValue);
System.out.println("The standard deviation is
deviationValue);
+
}
public static double mean (int boundary, int n) {
//Complete on your own
}
public static double deviation (int boundary, int n) {
//Complete on your own
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F34215fe4-737d-4111-858a-1ca99d565feb%2Fb4fab77f-4ec3-4522-a964-182629749839%2Fpug1h6_processed.png&w=3840&q=75)
![1. Download
ComputeMeanDeviation.java from M5 Methods module in folio.
2. Modify the program by adding the following two methods:
public static double mean (int boundary, int n)
public static double deviation (int boundary, int n)
In the mean method, generate n random integers = [0, boundary], compute and
return the result of the mean.
In the deviation method, generate n random integers = [0, boundary], compute
and return the result of the standard deviation.
The formulas to compute the mean and the standard deviation are:
n
Σx,
i=1
mean =
(x)
i=1
Σx².
deviation
i=1
n
=
n-1
3. Rewrite the main method so that it invokes the mean and deviation methods and
displays the results of the mean and the standard deviation. A sample main
method is as follows. Please type this main method in your own source code.
public static void main(String[] args) {
// Find mean
double meanValue = mean (99, 1000);
// Find standard deviation
double deviationValue = deviation (99, 1000);
// Display result
System.out.println("The mean is + meanValue);
System.out.println("The standard deviation is +
deviationValue);
"1
}|
n](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F34215fe4-737d-4111-858a-1ca99d565feb%2Fb4fab77f-4ec3-4522-a964-182629749839%2F9k3ayjn_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

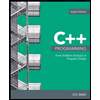
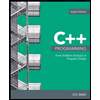