1. Implement a Student class. a. Create a class Student with the following private data members: 1. name 2. exam_1 grade 3. exam_2 grade b. Create all appropriate accessor and mutator functions. c. Assign appropriate access modifiers to insure encapsulation. d. Add a private calcGPA() function that calculates and returns the GPA based upon the two exam grades. e. Add a public getGrade() function that: 1. Obtains the GPA from the private calcGPA() function. 2. Returns a letter grade based upon the numerical GPA value. 90 to 100 = A 80 to 90 = B 70 to 80 = C 60 to 70 = D 0 to 60 = F f. Test all functions use following main. int main(){ Student a; a.setName("David"); a.setExam1(90); a.setExam2(80); cout<
1. Implement a Student class. a. Create a class Student with the following private data members: 1. name 2. exam_1 grade 3. exam_2 grade b. Create all appropriate accessor and mutator functions. c. Assign appropriate access modifiers to insure encapsulation. d. Add a private calcGPA() function that calculates and returns the GPA based upon the two exam grades. e. Add a public getGrade() function that: 1. Obtains the GPA from the private calcGPA() function. 2. Returns a letter grade based upon the numerical GPA value. 90 to 100 = A 80 to 90 = B 70 to 80 = C 60 to 70 = D 0 to 60 = F f. Test all functions use following main. int main(){ Student a; a.setName("David"); a.setExam1(90); a.setExam2(80); cout<
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
help with c++...paste indented code plzzz
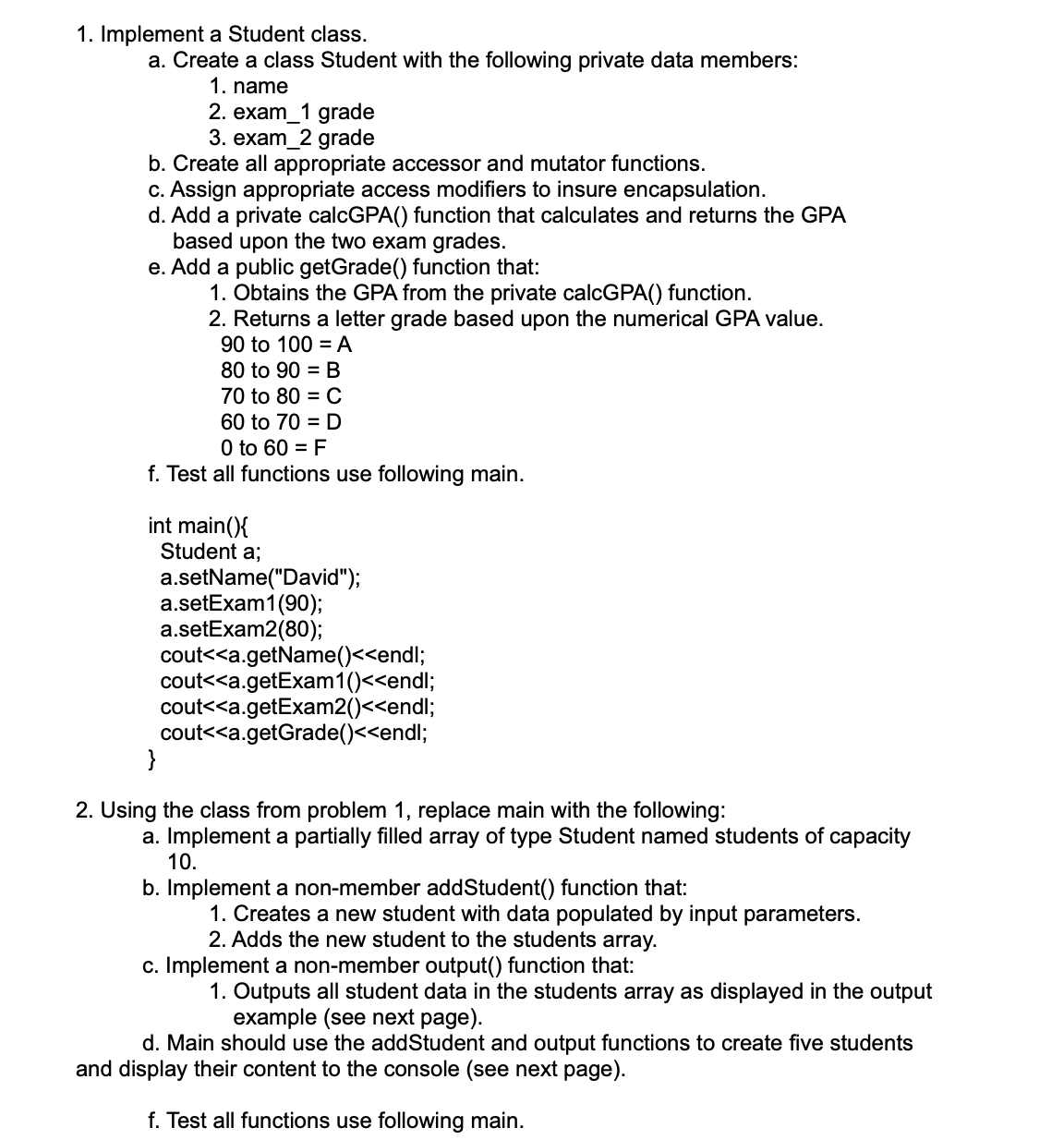
Transcribed Image Text:1. Implement a Student class.
a. Create a class Student with the following private data members:
1. name
2. exam_1 grade
3. exam_2 grade
b. Create all appropriate accessor and mutator functions.
c. Assign appropriate access modifiers to insure encapsulation.
d. Add a private calcGPA() function that calculates and returns the GPA
based upon the two exam grades.
e. Add a public getGrade() function that:
1. Obtains the GPA from the private calcGPA() function.
2. Returns a letter grade based upon the numerical GPA value.
90 to 100 = A
80 to 90 = B
70 to 80 = C
60 to 70 = D
0 to 60 = F
f. Test all functions use following main.
int main(){
Student a;
a.setName("David");
a.setExam1(90);
a.setExam2(80);
cout<<a.getName()<<endl;
cout<<a.getExam1()<<endl;
cout<<a.getExam2()<<endl;
cout<<a.getGrade()<<endl;
}
2. Using the class from problem 1, replace main with the following:
a. Implement a partially filled array of type Student named students of capacity
10.
b. Implement a non-member addStudent() function that:
1. Creates a new student with data populated by input parameters.
2. Adds the new student to the students array.
c. Implement a non-member output() function that:
1. Outputs all student data in the students array as displayed in the output
example (see next page).
d. Main should use the addStudent and output functions to create five students
and display their content to the console (see next page).
f. Test all functions use following main.
![int main(){
int capacity = 10;
Student students[capacity];
int num = 0;
addStudent(students,capacity,num,"Amy",95,90);
addStudent(students,capacity,num,"Bob",74,63);
addStudent(students,capacity,num,"Charlie",86,80);
addStudent(students,capacity,num,"Daisy",75,90);
addStudent(students,capacity,num,"Edward",24,66);
output(students,num);
}
Output Example
Name: Amy
Exam 1: 95
Exam 2: 9
GPA: A
Name: Bob
Exam 1: 74
Exam 2: 63
GPA: D
Name: Charlie
Exam 1: 86
Exam 2: 80
GPA: B
Name: Daisy
Exam 1: 75
Exam 2: 99
GPA: B
Name: David
Exam 1: 24
Exam 2: 66
GPA: F](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F67ea9fd4-b590-489e-a4cb-79b23e0f1970%2F4490186f-2cf9-4f13-a2b6-fd9fe93452b9%2Frzl885t_processed.png&w=3840&q=75)
Transcribed Image Text:int main(){
int capacity = 10;
Student students[capacity];
int num = 0;
addStudent(students,capacity,num,"Amy",95,90);
addStudent(students,capacity,num,"Bob",74,63);
addStudent(students,capacity,num,"Charlie",86,80);
addStudent(students,capacity,num,"Daisy",75,90);
addStudent(students,capacity,num,"Edward",24,66);
output(students,num);
}
Output Example
Name: Amy
Exam 1: 95
Exam 2: 9
GPA: A
Name: Bob
Exam 1: 74
Exam 2: 63
GPA: D
Name: Charlie
Exam 1: 86
Exam 2: 80
GPA: B
Name: Daisy
Exam 1: 75
Exam 2: 99
GPA: B
Name: David
Exam 1: 24
Exam 2: 66
GPA: F
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
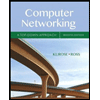
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
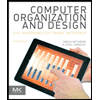
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
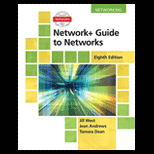
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
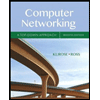
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
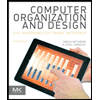
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
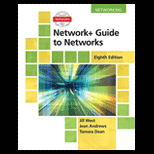
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
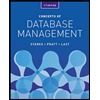
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
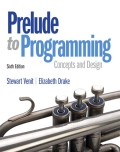
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
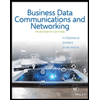
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY