1. Modify the recursive Fibonacci program given in the chapter so that it prints tracing information. Specifically, have the function print a message when it is called and when it returns. For example, the output should contain lines like these:
Q: Is it possible to sum up software engineering in a few words? What is it?
A: answer is
Q: Error messages are shown. Let us know which programmes have given you the best and worse error…
A: Definition: Talk about the finest and worst error messages you've ever gotten when using a software.…
Q: Discuss in a few lines the advantages and disadvantages of frequent software updates
A:
Q: Show that T(n) = 8n° + 2n + 1 is in O(n) using the definition of big-Oh. The definition of big-Oh:…
A:
Q: HOW CAN YOU AID IN PREVENTING INTERNAL AND EXTERNAL THREATS?
A: External assaults are generally pernicious, with disturbing help, vandalism, and burglary as the…
Q: Component-based programming and the impact it has had on the market for software components are the…
A: Before understanding component-based programming, it is vital to grasp the definition of a…
Q: Give two instances of an ALM environment and describe what it does.
A: Humans, tools, and procedures that govern the life cycle of an application from inception to end of…
Q: What do core software engineering ideas miss? Explain?
A: Introduction: Let's begin by defining what software engineering is. Software engineering is formed…
Q: Can someone explain how the output of this recursive function is 18? Recursion is confusing to me.…
A: The question is to find the reason: why the output of the given recursive function is 18. def R(n):…
Q: Please provide a brief description of the three primary forms of software maintenance. Why is it so…
A: NOTE :- Below i explain the answer in my own words by which you understand it well. The…
Q: “Integration testing is pointless,” your boss stated. Not required if each programme is sufficiently…
A: Software testing: Three layers of testing are often conducted in software testing. Unit testing…
Q: you create and use objects for primitive values using the wrapper classes (Byte, Short, Integer,…
A: Given: How and why do you create and use objects for primitive values using the wrapper classes…
Q: The ITEM table contains the following columns statement. Analyze the statement why it will fail…
A: Find the required answer given as below :
Q: Provide an overview of the three primary forms of software maintenance. Why is it so difficult to…
A: here we have given a brief note for question asked in terms of software maintenance and…
Q: The JavaScript statementlet pizzaPrice = rewardsCustomer ? 20.0 : 18.5;_____. a. assigns the value…
A: Solution and Explanation: Ternary operator: We use ternary operator to assign value based on…
Q: Write a program segment to display the content of the linked list.
A: Answer: Code: void display(){ struct MenuNode *ptr; if(front==NULL) {…
Q: g concepts are blind
A: Not Understanding The Needs Of The Business: One of the reasons software projects come up short is…
Q: There must be something fundamentally missing from the core concepts of software engineering.…
A: There must be something fundamentally missing from the core concepts of software engineering.…
Q: What are the advantages of a unified user interface (UX) in the context of SoS interface design?…
A: Given: Systems of systems (SOS) is the viewpoint of multiple dispersed, independent systems…
Q: What is the purpose of exceptions in process scheduling?
A: Introduction If a single operation is being performed numerous times, process scheduling is a…
Q: In JavaScript, if the variableflowerhas been assigned the value"bluebell", the…
A: Answer b) string
Q: Consider the graph in Figure. Find the shortest distance from node 0 to every other node in the…
A: This question belong to the Design and Analysis Algorithm domain of computer sciences and is solved…
Q: Kern32.dll is what kind of file?
A: answer is
Q: Given a page of HTML, you can add spans around some specific terms on the page. When the script is…
A: <TABLE border = 2><TR><TD><TABLE border =…
Q: socket
A: Explanation: <!DOCTYPE html> <html> <head> <meta charset="utf-8">…
Q: r operating sys
A: - Ubuntu is a Linux-based open-source working framework. Ubuntu is a Linux dispersion that might be…
Q: How much flow can you move from s to t? How did you determine this? 11 2 10 14 3 4 60 3.
A: We need to find the maximum possible flow from s to t.
Q: Using client-side scripting to handle light processing tasks such as data validation _____.
A: Here local processing of client computer can be found. A web application is a program that can be…
Q: is confusing to me. def R(n): if n>=5: return 2 return R(n+1) + 2
A: Given : def R(n): if n>=5: return 2 return R(n+1) + 2 print(R(0))
Q: According to server operating systems, what is unique about Ubuntu?
A: Ubuntu: Ubuntu is an OS based on Debian GNU/Linux. Ubuntu combines the capability of a Unix…
Q: n) but it is in 3M
A: Solution - In the given question, we have to prove the given relation schema is not in BCNF but it…
Q: Q8) Write a C++ program by creating an 'Employee' class having the following members Three Private…
A: Please find the answer below :
Q: As a student that has studied Human Resource Development, demonstrate the following: How…
A: According to the Bartleby guideline we are suppose to answer only one question at a time. Kindly…
Q: Which expressions have the most impact on C Programming? How do you use C variables, constants, and…
A: Impact on C Programming: How different forms of expressions affect C programming and the differences…
Q: What are the recommended procedures for assuring a local area network's resilience and availability?
A: The Answer start from step-2.
Q: A comparison of Jason Hickel's De-development framework with Martin Heidegger's The Question…
A: Explanation: The purpose of the developing framework is for sound or rich nations to "catch up" to…
Q: Convert there ExpressIons from OR Funchons to AHD Funchons only a = AB'C + A C d: AC' + A'B'C 2) any…
A:
Q: For example, what are the benefits and disadvantages of sequential and binary search algorithms?
A: Sequential and binary search are two basic algorithms used to execute a search within a given data…
Q: How to upload live html website using infinity?
A: Infinity: Infinity is the platform which provides the server to host the application online. It…
Q: Computer Science Write a PHP script demonstrating the use of regular expressions in server side…
A: Regular Expression Regular expressions are different pattern which are basically sub-strings that is…
Q: Identify from the SAD what security controls Transcon already has in place that could assist with…
A: Let's understand how spear-phishing works. There are actually 3 steps to show how it happens. In…
Q: distinction between yourdon and yourdon symbols in data and process modelling is there any…
A: Yourdon A proprietary software design process established by Ed Yourdon. It was one of the earliest…
Q: clarify the software development process's metricsștiiștiiștii? Everything must be thoroughly…
A: Software Metrics: A software metric is a measure of software characteristics which are measurable or…
Q: Can someone explain how the output of this recusrsive function is 5? I find recursion difficult to…
A: Here when values of n becomes 0, it return value 20. Orelse the value will call itself ie recursion…
Q: INSTRUCTIONS: Create a program that converts the PRICE CODE to PRICE VALUE. CODE C M U E R S VALUE 1…
A: We need to write a C++ program for the given scenario.
Q: Write a method that calculates and prints both the maximum humidity and maximum temperature values…
A: ANSWER:-
Q: es of softwa
A: The four fundamental activities of software development are as follows: 1- Specification of the…
Q: Describe how a database administrator backs up and recovers data in a client/server environment.
A: A database administrator is a specialized role within computer systems administration. Database…
Q: When compared to a database extension like mysqli for MySQL, what are the advantages and…
A: When compared to a database extension like mysqli for MySQL, what are the advantages and…
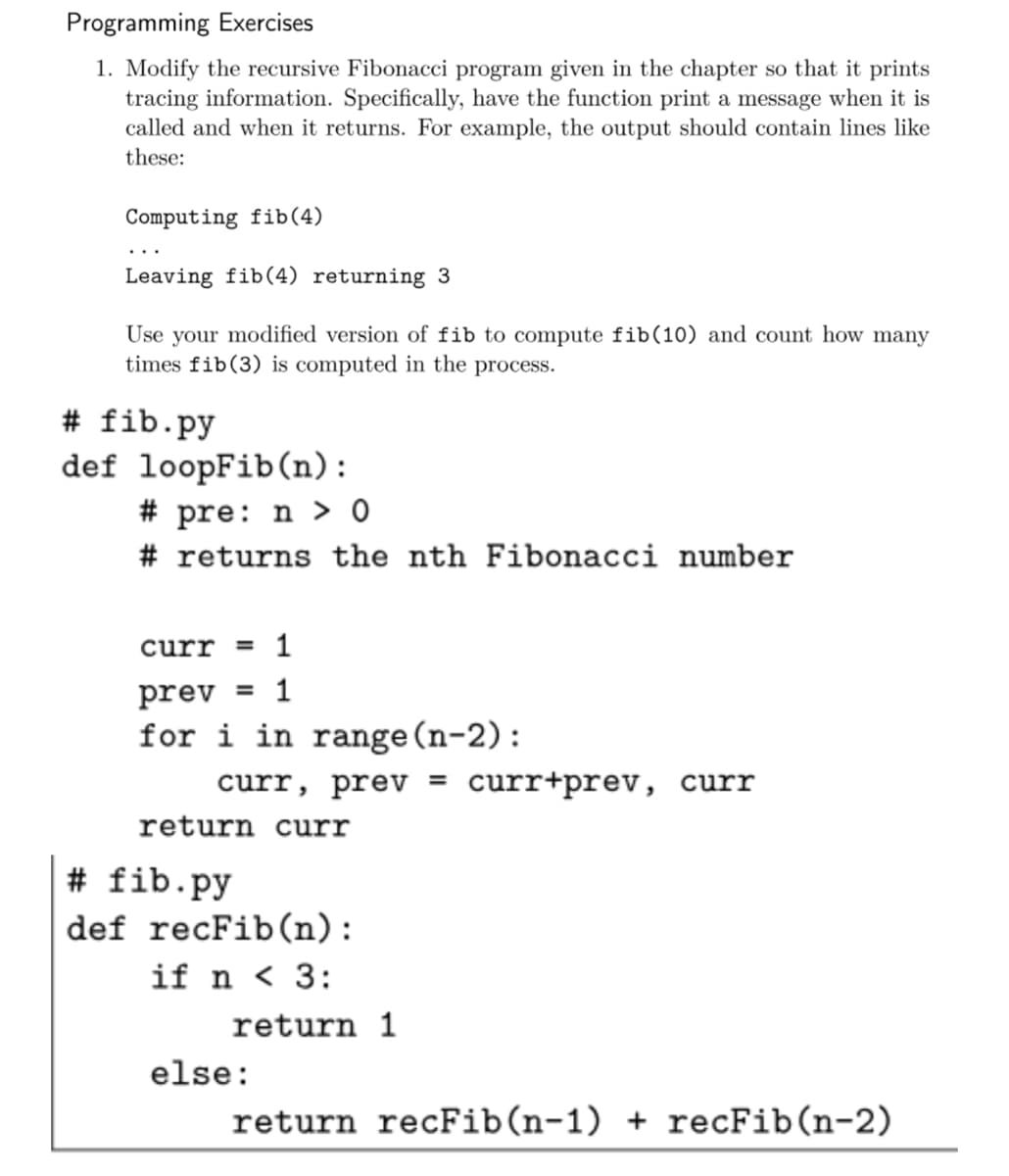

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

- Modify the recursive Fibonacci program given in this chapter so that itprints tracing information. Specifically, have the function print a messagewhen it is called and when it returns. For example, the output shouldcontain lines like these: Computing fib(4)0 0 0Leaving fib(4) returning 3 Use your modified version of fib to compute fib(10) and count howmany times fib(3) is computed in the process.For function log, write the missing base case condition and the recursive call. This function computes the log of n to the base b. As an example: log 8 to the base 2 equals 3 since 8 = 2*2*2. We can find this by dividing 8 by 2 until we reach 1, and we count the number of divisions we make. You should assume that n is exactly b to some integer power. Examples: log(2, 4) -> 2 and log(10, 100) -> 2 public int log(int b, int n ) { if <<Missing base case condition>> { return 0; } else { return <<Missing a Recursive case action>> }}A palindrome is a sentence that contains the same sequence of letters reading it either forwards or backwards. A classic example is '1\.ble was I, ereI saw Elba." Write a recursive function that detects whether a string is apalindrome. The basic idea is to check that the first and last letters of thestring are the same letter; if they are, then the entire string is a palindromeif everything between those letters is a palindrome.There are a couple of special cases to check for. If either the first orlast character of the string is not a letter, you can check to see if the restof the string is a palindrome with that character removed. Also, when youcompare letters, make sure that you do it in a case-insensitive way.Use your function in a program that prompts a user for a phrase andthen tells whether or not it is a palindrome. Here's another classic fortesting: '1\. man, a plan, a canal, Panama!"
- The Tower of Hanoi is a puzzle where n disks of different sizes arestacked in ascending order on one rod and there are two other rods with nodisks on them. The objective is to move all disks from the first rod to thethird, such that:- only one disk is moved at a time- a larger disk can never be placed on top of a smaller oneWrite a recursive function that outputs the sequence of steps needed tosolve the puzzle with n disks.Write a test program in C++ that allows the user to input number of disks andthen uses your function to output the steps needed to solve the puzzle.Hint: If you could move up n−1 of the disks from the first post to thethird post using the second post as a spare, the last disk could be moved fromthe first post to the second post. Then by using the same technique you canmove the n−1 disks from the third post to the second post, using the firstdisk as a spare. There! You have the puzzle solved. You only have to decidewhat the nonrecursive case is, what the recursive…Recursion can be direct or indirect. It is direct when a function calls itself and it is indirect recursion when a function calls another function that then calls the first function. To illustrate solving a problem using recursion, consider the Fibonacci series: - 1,1,2,3,5,8,13,21,34...The way to solve this problem is to examine the series carefully. The first two numbers are 1. Each subsequent number is the sum of the previous two numbers. Thus, the seventh number is the sum of the sixth and fifth numbers. More generally, the nth number is the sum of n - 2 and n - 1, as long as n > 2.Recursive functions need a stop condition. Something must happen to cause the program to stop recursing, or it will never end. In the Fibonacci series, n < 3 is a stop condition. The algorithm to use is this: 1. Ask the user for a position in the series.2. Call the fib () function with that position, passing in the value the user entered.3. The fib () function examines the argument (n). If n < 3…Write a recursive fibonacci (n) function with an expression body. The function should return an Int; it will be too slow to deal with inputs whose fibonacci numbers are too large anyway. You do *not* need to use memoization. Note that: fibonacci(0) = 0 fibonacci(1) = 1 fibonacci(n, where n is greater than 1) = fibonacci(n-2) + fibonacci(n - 1)
- Write a recursive function to implement the recursive algorithm (determining the number of ways to select a set of things from a given set of things). Also, write a program to test your function.Write a recursive function to implement the recursive algorithm (multiplying two positive integers using repeated addition). Also, write a program to test your function.Write a recursive function called print_num_pattern() to output the following number pattern. Given a positive integer as input (Ex: 12), subtract another positive integer (Ex: 3) continually until 0 or a negative value is reached, and then continually add the second integer until the first integer is again reached. For coding simplicity, output a space after every integer, including the last. Do not end output with a newline. Ex. If the input is: 12 3 the output is: 12 9 6 3 0 3 6 9 12 # TODO: Write recursive print_num_pattern() function if __name__ == "__main__": num1 = int(input()) num2 = int(input()) print_num_pattern(num1, num2)if (num1<=0): print(num1,end= '')
- Recursion efficiency in Julia can be improved using memorization technique to store the values of previously computed functions. The following Fibonacci function below does not employ memorization, please change into a recursive function with memorization: function fib(n) if n==1 return 1 elseif n==2 return 1 elseif n<=0 printstyled("Invalid input",color=:red) return else return fib(n-1)+fib(n-2) end endA recursive function is a function defined in terms of itself via self-referential expressions. This means that the function will continue to call itself and repeat its behavior until some condition is met to return a result. a- Write a python recursive function prod that takes x as an argument, and returns the result where, result=1*1/2*1/3*….*1/n b- Include a screenshot that shows a python program that uses the above function and prints the rounded result to three decimal places after prompting the user to enter a number, x. Use x=3. N.B: The code should be included in your answer.Write a recursive function in f#, named specialSum, that has the followeing signature: int * int -> int, where sum(m,n) = m + (m +1) + (m+2) + ... + (m + (n-1)) + (m+n) for m >= 0 and n >= 0: (Hint use two clauses with (m,0) and (m,n) A PATTERNS.) start code with let rec specialSum (m,n) match m,n with | m,0 -> | m,n - >
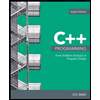
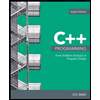