1. Problem Statement: North Flik is a smart digital ant. Write a python program that helps Flik to move in 4 directions: East, West, North, and South (See figure 1), just like we did with the 7Turtle, but be careful, you are West fast 0.0) not going to use graphics windows, and you are not going to use any of the methods in the Turtle module for that matter. All the outputs should be printed on the IPython Console pane. 2. Ant position: The current position of the bug is represented in a two-dimensional plane as a pair of coordinates (x, y) in the Figure I: Coordinates system Cartesian coordinate system shown in figure I. South 3. Tasks: The bug can perform the following tasks: • Move cast (i.e. to the right) by a steps. It requires updating the current position of the bug to the new position a steps to the right • Move west (ie. to the left) by a steps, It requires updating the current position of the bug to the new position a steps to the left. o Move north (ie. upward) by a steps. It requires updating the current position of the bug to the new position a steps upward. • Move south (i.e. downward) by a steps. It requires updating the current position of the bug to the new position a steps downward. o Calculate the Euclidian distance from the current position of the bug to its initial position. The Euclidian distance d between two points PI- (xl, yl) and P2- 2, y2) is: d= Jri - x2) + (y1 - y2) 4. Program Your program should perform the following tasks: • Read from a file called bugMoves.txt the initial position (x, y) of Flik • Then read line by line the remaining contents of the file and update the current position of Flik accordingly, in order to build the path that Flick has followed from the start. • Each subsequent line in the file represents a movement to be performed by the bug, See figure 2 (a). The movement of the bug is specified by the pair: [in meter). For instance: north 5 means move north by 5 meters. • Once the reading is completed, the program displays the following: See Figure 2(b) G The initial position of the ant Flik, • The path Flik has followed ie; the soquence of the successive bug's positions from its initial position to its final position, • Flik's final position, and o The Euclidian distance separating Flik from its original position. 5. Exception Handling: o Handle 10 exception when trying to open file that does not exist. O The 2D coordinates (x, y) of the bug's initial position ie; the first line of the input file must be intege numbers. In case the first line in the file contains floats, or non-digit characters, your program should raise a ValueError exception and the original position is set to (0,0) by default. See figure 3. • The number of steps to be executed by the bug should be a positive integer. In case the file contains erroneous values, your program should raise a ValueError exception and the instruction is simply ignored. See figure 3. 6. Input/Output Case I: Input Output corresponding to the case of eror-free file bugMoves.txt wit. dons 10 12 east 1 north 5 west 3 south 1 west 2 south 1 (a) Error-free input file Fick Hello, I an Flick the snart ant!!! I an waiting for your instructions... Flick I an at the initial position (10, 12) FLick This is my itinirary: (10, 12) (11, 12) (11, 17) (8, 17) (8. 16) (6, 16) (6, 15) FLICK I an at my final position (6, 15) FLick I an 5.00 meters fron the initial position Flick Nice working with you...Byet (b) Output corresponding to the Errer-free input file
1. Problem Statement: North Flik is a smart digital ant. Write a python program that helps Flik to move in 4 directions: East, West, North, and South (See figure 1), just like we did with the 7Turtle, but be careful, you are West fast 0.0) not going to use graphics windows, and you are not going to use any of the methods in the Turtle module for that matter. All the outputs should be printed on the IPython Console pane. 2. Ant position: The current position of the bug is represented in a two-dimensional plane as a pair of coordinates (x, y) in the Figure I: Coordinates system Cartesian coordinate system shown in figure I. South 3. Tasks: The bug can perform the following tasks: • Move cast (i.e. to the right) by a steps. It requires updating the current position of the bug to the new position a steps to the right • Move west (ie. to the left) by a steps, It requires updating the current position of the bug to the new position a steps to the left. o Move north (ie. upward) by a steps. It requires updating the current position of the bug to the new position a steps upward. • Move south (i.e. downward) by a steps. It requires updating the current position of the bug to the new position a steps downward. o Calculate the Euclidian distance from the current position of the bug to its initial position. The Euclidian distance d between two points PI- (xl, yl) and P2- 2, y2) is: d= Jri - x2) + (y1 - y2) 4. Program Your program should perform the following tasks: • Read from a file called bugMoves.txt the initial position (x, y) of Flik • Then read line by line the remaining contents of the file and update the current position of Flik accordingly, in order to build the path that Flick has followed from the start. • Each subsequent line in the file represents a movement to be performed by the bug, See figure 2 (a). The movement of the bug is specified by the pair: [in meter). For instance: north 5 means move north by 5 meters. • Once the reading is completed, the program displays the following: See Figure 2(b) G The initial position of the ant Flik, • The path Flik has followed ie; the soquence of the successive bug's positions from its initial position to its final position, • Flik's final position, and o The Euclidian distance separating Flik from its original position. 5. Exception Handling: o Handle 10 exception when trying to open file that does not exist. O The 2D coordinates (x, y) of the bug's initial position ie; the first line of the input file must be intege numbers. In case the first line in the file contains floats, or non-digit characters, your program should raise a ValueError exception and the original position is set to (0,0) by default. See figure 3. • The number of steps to be executed by the bug should be a positive integer. In case the file contains erroneous values, your program should raise a ValueError exception and the instruction is simply ignored. See figure 3. 6. Input/Output Case I: Input Output corresponding to the case of eror-free file bugMoves.txt wit. dons 10 12 east 1 north 5 west 3 south 1 west 2 south 1 (a) Error-free input file Fick Hello, I an Flick the snart ant!!! I an waiting for your instructions... Flick I an at the initial position (10, 12) FLick This is my itinirary: (10, 12) (11, 12) (11, 17) (8, 17) (8. 16) (6, 16) (6, 15) FLICK I an at my final position (6, 15) FLick I an 5.00 meters fron the initial position Flick Nice working with you...Byet (b) Output corresponding to the Errer-free input file
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
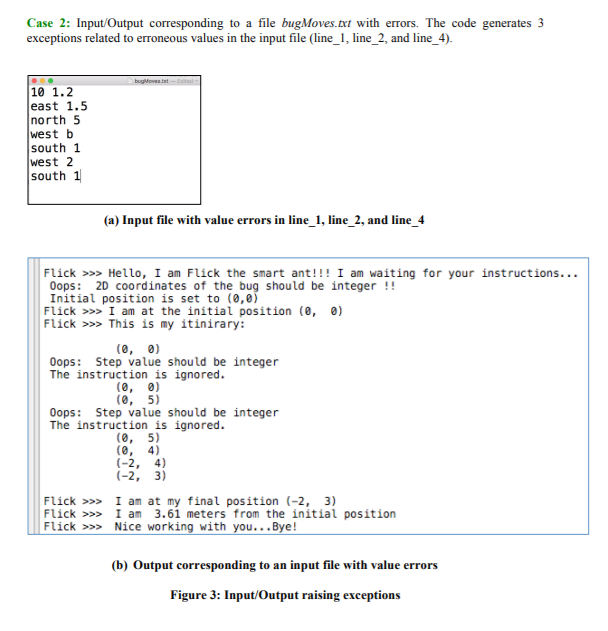
Transcribed Image Text:Case 2: Input/Output corresponding to a file bugMoves.txt with errors. The code generates 3
exceptions related to erroneous values in the input file (line_1, line_2, and line_4).
bugMovest
10 1.2
east 1.5
north 5
west b
south 1
west 2
south 1
(a) Input file with value errors in line_1, line_2, and line_4
Flick >>> Hello, I am Flick the smart ant!!! I am waiting for your instructions...
Oops: 20 coordinates of the bug should be integer !!
Initial position is set to (0,0)
Flick >>> I am at the initial position (0, 0)
Flick >>> This is my itinirary:
(0, 0)
Oops: Step value should be integer
The instruction is ignored.
(0, 0)
(0, 5)
Oops: Step value should be integer
The instruction is ignored.
(0, 5)
(0, 4)
(-2, 4)
(-2, 3)
Flick >>> I am at my final position (-2, 3)
Flick >>> I am 3.61 meters from the initial position
Flick >>> Nice working with you...Bye!
(b) Output corresponding to an input file with value errors
Figure 3: Input/Output raising exceptions
![1. Problem Statement:
North
Flik is a smart digital ant. Write a python program that helps Flik
to move in 4 directions: East, West, North, and South (See
figure 1), just like we did with the Turtle, but be careful, you are
not going to use graphics windows, and you are not going to use
any of the methods in the Turtle module for that matter. All the
outputs should be printed on the IPython Console pane.
West
East
(0,0)
2. Ant position: The current position of the bug is represented in a
two-dimensional plane as a pair of coordinates (x, y) in the Figure I: Coordinates system
Cartesian coordinate system shown in figure 1.
South
3. Tasks: The bug can perform the following tasks:
• Move east (i.e. to the right) by n steps. It requires updating the current position of the bug to the
new position n steps to the right.
o Move west (i.e. to the left) by a steps. It requires updating the current position of the bug to the
new position n steps to the left.
• Move north (i.e. upward) by a steps. It requires updating the current position of the bug to the
new position a steps upward.
• Move south (i.e. downward) by w steps. It requires updating the current position of the bug to the
new position n steps downward.
Calculate the Euclidian distance from the current position of the bug to its initial position. The
Euclidian distance d between two points PI= (xl, yl) and P2 = (x2, y2) is:
d = (x1 - x2) + (yl – y2)
4. Program
Your program should perform the following tasks:
• Read from a file called bugMoves.txt the initial position (x, y) of Flik
• Then read line by line the remaining contents of the file and update the current position of Flik
accordingly, in order to build the path that Flick has followed from the start.
• Each subsequent line in the file represents a movement to be performed by the bug, See figure
2 (a). The movement of the bug is specified by the pair: <direction> <the number of
steps> [in meter]. For instance: north 5 means move north by 5 meters.
• Once the reading is completed, the program displays the following: See Figure 2(b)
e The initial position of the ant Flik,
e The path Flik has followed ie.; the sequence of the successive bug's positions from its
initial position to its final position,
o Flik's final position, and
e The Euclidian distance separating Flik from its original position.
2
5. Exception Handling:
O Handle 10 exception when trying to open file that does not exist.
The 2D coordinates (x, y) of the bug's initial position i.e; the first line of the input file must be
integer numbers. In case the first line in the file contains floats, or non-digit characters, your
program should raise a ValueError exception and the original position is set to (0,0) by
default. See figure 3.
O The number of steps to be executed by the bug should be a positive integer. In case the file
contains erroneous values, your program should raise a ValueError exception and the
instruction is simply ignored. See figure 3.
6. Input/Output
Case 1: Input/Output corresponding to the case of error-free file bugMoves.txt wit.
dons
bugave
10 12
east 1
north 5
west 3
south 1
west 2
south 1
(a) Error-free input file
Flick > Hello, I an Flick the smart ant!!! I an waiting for your instructions...
Flick > I am at the initial position (18, 12)
Flick >> This is my itinirary:
(10, 12)
(11, 12)
(11, 17)
(8, 17)
(в, 16)
(6, 16)
(6, 15)
Flick >>> I an at my final position (6, 15)
Flick >> I an 5.00 meters fron the initial position
Flick > Nice working with you...Bye!
(b) Output corresponding to the Error-free input file
Figure 2: Input/Output with no exceptions](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa35c5bfe-a0b7-41ac-9d18-a6f9f83261ec%2Fd1c4580a-c6e5-41b6-9f1e-3083d6e92423%2Fm9q1fcm_processed.jpeg&w=3840&q=75)
Transcribed Image Text:1. Problem Statement:
North
Flik is a smart digital ant. Write a python program that helps Flik
to move in 4 directions: East, West, North, and South (See
figure 1), just like we did with the Turtle, but be careful, you are
not going to use graphics windows, and you are not going to use
any of the methods in the Turtle module for that matter. All the
outputs should be printed on the IPython Console pane.
West
East
(0,0)
2. Ant position: The current position of the bug is represented in a
two-dimensional plane as a pair of coordinates (x, y) in the Figure I: Coordinates system
Cartesian coordinate system shown in figure 1.
South
3. Tasks: The bug can perform the following tasks:
• Move east (i.e. to the right) by n steps. It requires updating the current position of the bug to the
new position n steps to the right.
o Move west (i.e. to the left) by a steps. It requires updating the current position of the bug to the
new position n steps to the left.
• Move north (i.e. upward) by a steps. It requires updating the current position of the bug to the
new position a steps upward.
• Move south (i.e. downward) by w steps. It requires updating the current position of the bug to the
new position n steps downward.
Calculate the Euclidian distance from the current position of the bug to its initial position. The
Euclidian distance d between two points PI= (xl, yl) and P2 = (x2, y2) is:
d = (x1 - x2) + (yl – y2)
4. Program
Your program should perform the following tasks:
• Read from a file called bugMoves.txt the initial position (x, y) of Flik
• Then read line by line the remaining contents of the file and update the current position of Flik
accordingly, in order to build the path that Flick has followed from the start.
• Each subsequent line in the file represents a movement to be performed by the bug, See figure
2 (a). The movement of the bug is specified by the pair: <direction> <the number of
steps> [in meter]. For instance: north 5 means move north by 5 meters.
• Once the reading is completed, the program displays the following: See Figure 2(b)
e The initial position of the ant Flik,
e The path Flik has followed ie.; the sequence of the successive bug's positions from its
initial position to its final position,
o Flik's final position, and
e The Euclidian distance separating Flik from its original position.
2
5. Exception Handling:
O Handle 10 exception when trying to open file that does not exist.
The 2D coordinates (x, y) of the bug's initial position i.e; the first line of the input file must be
integer numbers. In case the first line in the file contains floats, or non-digit characters, your
program should raise a ValueError exception and the original position is set to (0,0) by
default. See figure 3.
O The number of steps to be executed by the bug should be a positive integer. In case the file
contains erroneous values, your program should raise a ValueError exception and the
instruction is simply ignored. See figure 3.
6. Input/Output
Case 1: Input/Output corresponding to the case of error-free file bugMoves.txt wit.
dons
bugave
10 12
east 1
north 5
west 3
south 1
west 2
south 1
(a) Error-free input file
Flick > Hello, I an Flick the smart ant!!! I an waiting for your instructions...
Flick > I am at the initial position (18, 12)
Flick >> This is my itinirary:
(10, 12)
(11, 12)
(11, 17)
(8, 17)
(в, 16)
(6, 16)
(6, 15)
Flick >>> I an at my final position (6, 15)
Flick >> I an 5.00 meters fron the initial position
Flick > Nice working with you...Bye!
(b) Output corresponding to the Error-free input file
Figure 2: Input/Output with no exceptions
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 6 images

Recommended textbooks for you
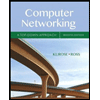
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
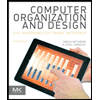
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
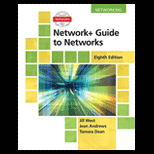
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
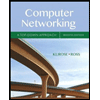
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
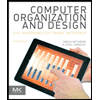
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
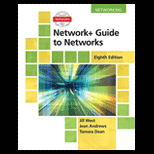
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
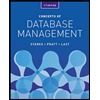
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
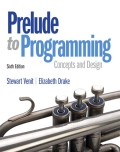
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
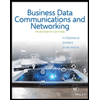
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY