1. Write a function called is equal that takes two numbers and returns True if the numbers are equal and False if they are not equal. This function will be tested using unit tests and requires no input and does not need to be called from the main() function.
1. Write a function called is equal that takes two numbers and returns True if the numbers are equal and False if they are not equal. This function will be tested using unit tests and requires no input and does not need to be called from the main() function.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section6.2: Returning A Single Value
Problem 13E
Related questions
Question
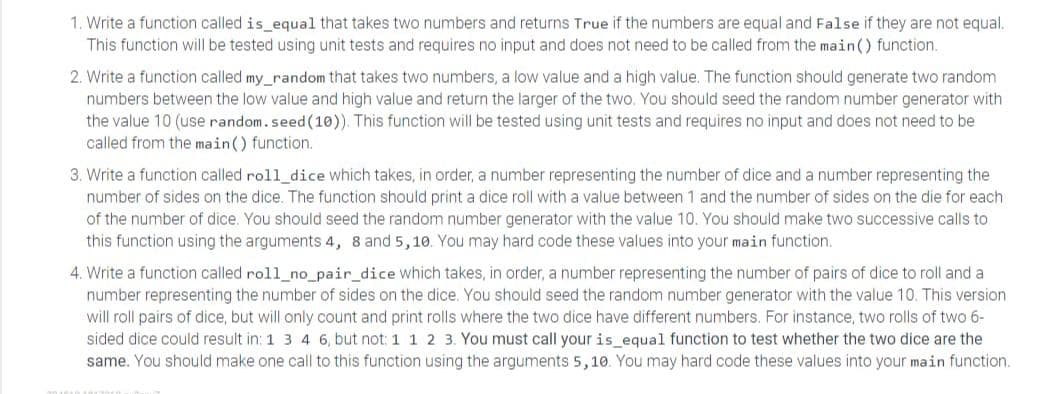
Transcribed Image Text:1. Write a function called is_equal that takes two numbers and returns True if the numbers are equal and False if they are not equal.
This function will be tested using unit tests and requires no input and does not need to be called from the main() function.
2. Write a function called my_random that takes two numbers, a low value and a high value. The function should generate two random
numbers between the low value and high value and return the larger of the two. You should seed the random number generator with
the value 10 (use random.seed (10)). This function will be tested using unit tests and requires no input and does not need to be
called from the main() function.
3. Write a function called roll_dice which takes, in order, a number representing the number of dice and a number representing the
number of sides on the dice. The function should print a dice roll with a value between 1 and the number of sides on the die for each
of the number of dice. You should seed the random number generator with the value 10. You should make two successive calls to
this function using the arguments 4, 8 and 5,10. You may hard code these values into your main function.
4. Write a function called roll_no_pair_dice which takes, in order, a number representing the number of pairs of dice to roll and a
number representing the number of sides on the dice. You should seed the random number generator with the value 10. This version
will roll pairs of dice, but will only count and print rolls where the two dice have different numbers. For instance, two rolls of two 6-
sided dice could result in: 1 3 4 6, but not: 1 1 2 3. You must call your is_equal function to test whether the two dice are the
same. You should make one call to this function using the arguments 5,10. You may hard code these values into your main function.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
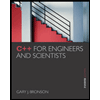
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
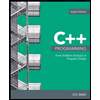
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
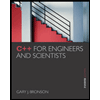
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
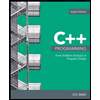
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning