10.15 LAB: Plant information (vector) C++ Given a base Plant class and a derived Flower class, complete main() to create a vector called myGarden. The vector should be able to store objects that belong to the Plant class or the Flower class. Create a function called PrintVector(), that uses the PrintInfo() functions defined in the respective classes and prints each element in myGarden. The program should read plants or flowers from input (ending with -1), adding each Plant or Flower to the myGarden vector, and output each element in myGarden using the PrintInfo() function. Ex. If the input is: plant Spirea 10 flower Hydrangea 30 false lilac flower Rose 6 false white plant Mint 4 -1 the output is: Plant Information: Plant name: Spirea Cost: 10 Plant Information: Plant name: Hydrengea Cost: 30 Annual: false Color of flowers: lilac Plant Information: Plant name: Rose Cost: 6 Annual: false Color of flowers: white Plant Information: Plant name: Mint Cost: 4 I need help with the main.cpp main.cpp: #include "Plant.h" #include "Flower.h" #include #include #include using namespace std; // TODO: Define a PrintVector function that prints an vector of plant (or flower) object pointers int main(int argc, char* argv[]) { // TODO: Declare a vector called myGarden that can hold object of type plant pointer // TODO: Declare variables - plantName, plantCost, flowerName, flowerCost, // colorOfFlowers, isAnnual string input; cin >> input; while(input != "-1") { // TODO: Check if input is a plant or flower // Store as a plant object or flower object // Add to the vector myGarden cin >> input; } // TODO: Call the method PrintVector to print myGarden for (size_t i = 0; i < myGarden.size(); ++i) { delete myGarden.at(i); } return 0; } Plant.h: #ifndef PLANTH #define PLANTH #include using namespace std; class Plant { public: virtual ~Plant(); void SetPlantName(string userPlantName); string GetPlantName() const; void SetPlantCost(int userPlantCost); int GetPlantCost() const; virtual void PrintInfo() const; protected: string plantName; int plantCost; }; #endif Plant.cpp: #include "Plant.h" #include Plant::~Plant() {}; void Plant::SetPlantName(string userPlantName) { plantName = userPlantName; } string Plant::GetPlantName() const { return plantName; } void Plant::SetPlantCost(int userPlantCost) { plantCost = userPlantCost; } int Plant::GetPlantCost() const { return plantCost; } void Plant::PrintInfo() const { cout << "Plant Information:" << endl; cout << " Plant name: " << plantName << endl; cout << " Cost: " << plantCost << endl; } Flower.h: #ifndef FLOWERH #define FLOWERH #include "Plant.h" #include using namespace std; class Flower : public Plant { public: void SetPlantType(bool userIsAnnual); bool GetPlantType() const; void SetColorOfFlowers(string userColorOfFlowers); string GetColorOfFlowers() const; void PrintInfo() const; private: bool isAnnual; string colorOfFlowers; }; #endif Flower.cpp: #include "Flower.h" #include void Flower::SetPlantType(bool userIsAnnual) { isAnnual = userIsAnnual; } bool Flower::GetPlantType() const { return isAnnual; } void Flower::SetColorOfFlowers(string userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } string Flower::GetColorOfFlowers() const { return colorOfFlowers; } void Flower::PrintInfo() const { cout << "Plant Information:" << endl; cout << " Plant name: " << plantName << endl; cout << " Cost: " << plantCost << endl; cout << " Annual: " << boolalpha << isAnnual << endl; cout << " Color of flowers: " << colorOfFlowers << endl; }
10.15 LAB: Plant information (vector) C++ Given a base Plant class and a derived Flower class, complete main() to create a vector called myGarden. The vector should be able to store objects that belong to the Plant class or the Flower class. Create a function called PrintVector(), that uses the PrintInfo() functions defined in the respective classes and prints each element in myGarden. The program should read plants or flowers from input (ending with -1), adding each Plant or Flower to the myGarden vector, and output each element in myGarden using the PrintInfo() function. Ex. If the input is: plant Spirea 10 flower Hydrangea 30 false lilac flower Rose 6 false white plant Mint 4 -1 the output is: Plant Information: Plant name: Spirea Cost: 10 Plant Information: Plant name: Hydrengea Cost: 30 Annual: false Color of flowers: lilac Plant Information: Plant name: Rose Cost: 6 Annual: false Color of flowers: white Plant Information: Plant name: Mint Cost: 4 I need help with the main.cpp main.cpp: #include "Plant.h" #include "Flower.h" #include #include #include using namespace std; // TODO: Define a PrintVector function that prints an vector of plant (or flower) object pointers int main(int argc, char* argv[]) { // TODO: Declare a vector called myGarden that can hold object of type plant pointer // TODO: Declare variables - plantName, plantCost, flowerName, flowerCost, // colorOfFlowers, isAnnual string input; cin >> input; while(input != "-1") { // TODO: Check if input is a plant or flower // Store as a plant object or flower object // Add to the vector myGarden cin >> input; } // TODO: Call the method PrintVector to print myGarden for (size_t i = 0; i < myGarden.size(); ++i) { delete myGarden.at(i); } return 0; } Plant.h: #ifndef PLANTH #define PLANTH #include using namespace std; class Plant { public: virtual ~Plant(); void SetPlantName(string userPlantName); string GetPlantName() const; void SetPlantCost(int userPlantCost); int GetPlantCost() const; virtual void PrintInfo() const; protected: string plantName; int plantCost; }; #endif Plant.cpp: #include "Plant.h" #include Plant::~Plant() {}; void Plant::SetPlantName(string userPlantName) { plantName = userPlantName; } string Plant::GetPlantName() const { return plantName; } void Plant::SetPlantCost(int userPlantCost) { plantCost = userPlantCost; } int Plant::GetPlantCost() const { return plantCost; } void Plant::PrintInfo() const { cout << "Plant Information:" << endl; cout << " Plant name: " << plantName << endl; cout << " Cost: " << plantCost << endl; } Flower.h: #ifndef FLOWERH #define FLOWERH #include "Plant.h" #include using namespace std; class Flower : public Plant { public: void SetPlantType(bool userIsAnnual); bool GetPlantType() const; void SetColorOfFlowers(string userColorOfFlowers); string GetColorOfFlowers() const; void PrintInfo() const; private: bool isAnnual; string colorOfFlowers; }; #endif Flower.cpp: #include "Flower.h" #include void Flower::SetPlantType(bool userIsAnnual) { isAnnual = userIsAnnual; } bool Flower::GetPlantType() const { return isAnnual; } void Flower::SetColorOfFlowers(string userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } string Flower::GetColorOfFlowers() const { return colorOfFlowers; } void Flower::PrintInfo() const { cout << "Plant Information:" << endl; cout << " Plant name: " << plantName << endl; cout << " Cost: " << plantCost << endl; cout << " Annual: " << boolalpha << isAnnual << endl; cout << " Color of flowers: " << colorOfFlowers << endl; }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
10.15 LAB: Plant information (vector ) C++
Given a base Plant class and a derived Flower class, complete main() to create a vector called myGarden. The vector should be able to store objects that belong to the Plant class or the Flower class. Create a function called PrintVector(), that uses the PrintInfo() functions defined in the respective classes and prints each element in myGarden. The program should read plants or flowers from input (ending with -1), adding each Plant or Flower to the myGarden vector, and output each element in myGarden using the PrintInfo() function.
Ex. If the input is:
plant Spirea 10
flower Hydrangea 30 false lilac
flower Rose 6 false white
plant Mint 4
-1
the output is:
Plant Information:
Plant name: Spirea
Cost: 10
Plant Information:
Plant name: Hydrengea
Cost: 30
Annual: false
Color of flowers: lilac
Plant Information:
Plant name: Rose
Cost: 6
Annual: false
Color of flowers: white
Plant Information:
Plant name: Mint
Cost: 4
I need help with the main.cpp
main.cpp:
#include "Plant.h"
#include "Flower.h"
#include
#include
#include
using namespace std;
// TODO: Define a PrintVector function that prints an vector of plant (or flower) object pointers
int main(int argc, char* argv[]) {
// TODO: Declare a vector called myGarden that can hold object of type plant pointer
// TODO: Declare variables - plantName, plantCost, flowerName, flowerCost,
// colorOfFlowers, isAnnual
string input;
cin >> input;
while(input != "-1") {
// TODO: Check if input is a plant or flower
// Store as a plant object or flower object
// Add to the vector myGarden
cin >> input;
}
// TODO: Call the method PrintVector to print myGarden
for (size_t i = 0; i < myGarden.size(); ++i) {
delete myGarden.at(i);
}
return 0;
}
Plant.h:
#ifndef PLANTH
#define PLANTH
#include
using namespace std;
class Plant {
public:
virtual ~Plant();
void SetPlantName(string userPlantName);
string GetPlantName() const;
void SetPlantCost(int userPlantCost);
int GetPlantCost() const;
virtual void PrintInfo() const;
protected:
string plantName;
int plantCost;
};
#endif
Plant.cpp:
#include "Plant.h"
#include
Plant::~Plant() {};
void Plant::SetPlantName(string userPlantName) {
plantName = userPlantName;
}
string Plant::GetPlantName() const {
return plantName;
}
void Plant::SetPlantCost(int userPlantCost) {
plantCost = userPlantCost;
}
int Plant::GetPlantCost() const {
return plantCost;
}
void Plant::PrintInfo() const {
cout << "Plant Information:" << endl;
cout << " Plant name: " << plantName << endl;
cout << " Cost: " << plantCost << endl;
}
Flower.h:
#ifndef FLOWERH
#define FLOWERH
#include "Plant.h"
#include
using namespace std;
class Flower : public Plant {
public:
void SetPlantType(bool userIsAnnual);
bool GetPlantType() const;
void SetColorOfFlowers(string userColorOfFlowers);
string GetColorOfFlowers() const;
void PrintInfo() const;
private:
bool isAnnual;
string colorOfFlowers;
};
#endif
Flower.cpp:
#include "Flower.h"
#include
void Flower::SetPlantType(bool userIsAnnual) {
isAnnual = userIsAnnual;
}
bool Flower::GetPlantType() const {
return isAnnual;
}
void Flower::SetColorOfFlowers(string userColorOfFlowers) {
colorOfFlowers = userColorOfFlowers;
}
string Flower::GetColorOfFlowers() const {
return colorOfFlowers;
}
void Flower::PrintInfo() const {
cout << "Plant Information:" << endl;
cout << " Plant name: " << plantName << endl;
cout << " Cost: " << plantCost << endl;
cout << " Annual: " << boolalpha << isAnnual << endl;
cout << " Color of flowers: " << colorOfFlowers << endl;
}
![LAB
10.15.1: LAB: Plant information (vector)
0/10
АCTIVITY
Current file: main.cpp
Load defa
1 #include "Plant.h"
2 #include "Flower.h"
3 #include <vector>
4 #include <string>
5 #include <iostream>
7 using namespace std;
8
9 // TODO: Define a PrintVector function that prints an vector of plant (or flower) object pointers
10
11 int main(int argc, char* argv[]) {
12
// TODO: Declare a vector called myGarden that can hold object of type plant pointer
13
14
// TODO: Declare variables - plantName, plantCost, flowerName, flowerCost,
15
//
colorofFlowers, tiSAnnual
16
17
18
string input;
cin >> input;
19
20
21
22
while(input !- "-1") {
// TODO: Check if input is a plant or flower
Store as a plant object or flower object
Add to the vector myGarden
//
23
24
25
//
cin > input;
}
26
27
// TODO: Call the method PrintVector to print myGarden
28
29
for (size_t i - 0; i < myGarden.size(); ++i) {
delete myGarden.at(i);
30
31
32
33
return 0;
34](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F26fe0435-62a7-4f5e-a128-5f4edfda4971%2F2333e9a6-e772-4abd-8f38-41930056f3e7%2Fndplaxb_processed.jpeg&w=3840&q=75)
Transcribed Image Text:LAB
10.15.1: LAB: Plant information (vector)
0/10
АCTIVITY
Current file: main.cpp
Load defa
1 #include "Plant.h"
2 #include "Flower.h"
3 #include <vector>
4 #include <string>
5 #include <iostream>
7 using namespace std;
8
9 // TODO: Define a PrintVector function that prints an vector of plant (or flower) object pointers
10
11 int main(int argc, char* argv[]) {
12
// TODO: Declare a vector called myGarden that can hold object of type plant pointer
13
14
// TODO: Declare variables - plantName, plantCost, flowerName, flowerCost,
15
//
colorofFlowers, tiSAnnual
16
17
18
string input;
cin >> input;
19
20
21
22
while(input !- "-1") {
// TODO: Check if input is a plant or flower
Store as a plant object or flower object
Add to the vector myGarden
//
23
24
25
//
cin > input;
}
26
27
// TODO: Call the method PrintVector to print myGarden
28
29
for (size_t i - 0; i < myGarden.size(); ++i) {
delete myGarden.at(i);
30
31
32
33
return 0;
34
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 7 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
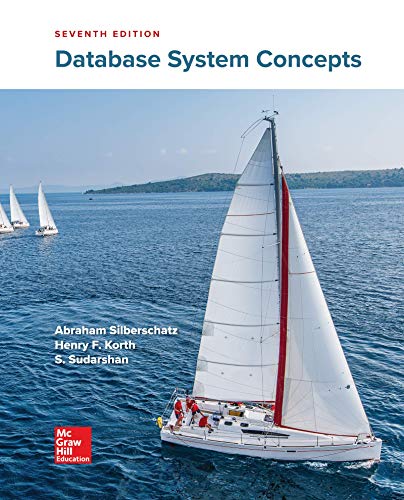
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
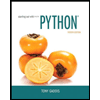
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
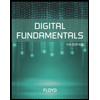
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
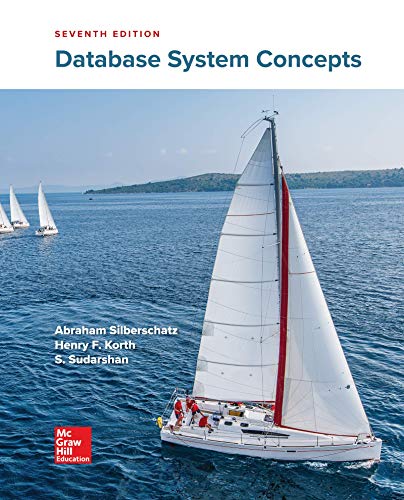
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
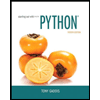
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
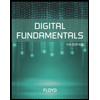
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
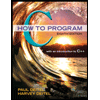
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
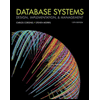
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
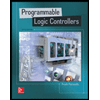
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education