11.7. Non-sequential Collections This says to set the count associated with word w to be one more than the current count for w. There is one small complication with using a dictionary here. The first time we encounter a word, it will not yet be in counts. Attempting to access a non- existent key produces a run-time KeyError. To guard against this, we need a decision in our algorithm: if w is already in counts: add one to the count for w else: set count for w to 1 This decision ensures that the first time a word is encountered, it will be entered into the dictionary with a count of 1. One way to implement this decision is to use the in operator: if w in counts: counts [w] counts [w] + 1 else: counts [w] 1 A more elegant approach is to use the get method: counts [v] counts.get (,0) + 1 If w is not already in the dictionary, this get will return 0, and the result is that the entry for w is set to 1. The dictionary updating code will form the heart of our program. We just need to fill in the parts around it. We will need to split our text document into a sequence of words. Before splitting, though, it's useful to convert all the text to lowercase (so occurrences of "Foo" match "foo") and eliminate punctuation (so "foo," matches "foo"). Here's the code to do those three tasks: fname input ("File to analyze: ") #read file as one long string text= open(fname, "r").read() #convert all letters to lower case text text.lower()
11.7. Non-sequential Collections This says to set the count associated with word w to be one more than the current count for w. There is one small complication with using a dictionary here. The first time we encounter a word, it will not yet be in counts. Attempting to access a non- existent key produces a run-time KeyError. To guard against this, we need a decision in our algorithm: if w is already in counts: add one to the count for w else: set count for w to 1 This decision ensures that the first time a word is encountered, it will be entered into the dictionary with a count of 1. One way to implement this decision is to use the in operator: if w in counts: counts [w] counts [w] + 1 else: counts [w] 1 A more elegant approach is to use the get method: counts [v] counts.get (,0) + 1 If w is not already in the dictionary, this get will return 0, and the result is that the entry for w is set to 1. The dictionary updating code will form the heart of our program. We just need to fill in the parts around it. We will need to split our text document into a sequence of words. Before splitting, though, it's useful to convert all the text to lowercase (so occurrences of "Foo" match "foo") and eliminate punctuation (so "foo," matches "foo"). Here's the code to do those three tasks: fname input ("File to analyze: ") #read file as one long string text= open(fname, "r").read() #convert all letters to lower case text text.lower()
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Python
wordfreq.py
![405
11.7. Non-sequential Collections
This says to set the count associated with word w to be one more than the current
count for w.
There is one small complication with using a dictionary here. The first time
we encounter a word, it will not yet be in counts. Attempting to access a non-
existent key produces a run-time KeyError. To guard against this, we need a
decision in our algorithm:
if w is already in counts:
add one to the count for w
else:
set count for w to 1
This decision ensures that the first time a word is encountered, it will be entered
into the dictionary with a count of 1.
One way to implement this decision is to use the in operator:
if w in counts:
counts [w] = counts [w] + 1
else:
counts [w] = 1
A more elegant approach is to use the get method:
counts [w] counts.get (w,0) + 1
If w is not already in the dictionary, this get will return 0, and the result is that
the entry for w is set to 1.
The dictionary updating code will form the heart of our program. We just
need to fill in the parts around it. We will need to split our text document into a
sequence of words. Before splitting, though, it's useful to convert all the text to
lowercase (so occurrences of "Foo" match "foo") and eliminate punctuation (so
"foo," matches "foo"). Here's the code to do those three tasks:
fname = input ("File to analyze: ")
#read file as one long string
text= open(fname, "r").read()
#convert all letters to lower case
text= text.lower()
J
A](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F664a59d7-783e-42dd-9b24-7e03a0a10493%2F95fd7033-d541-4f94-92a3-27d6942a5a3a%2F3sxdxf9_processed.jpeg&w=3840&q=75)
Transcribed Image Text:405
11.7. Non-sequential Collections
This says to set the count associated with word w to be one more than the current
count for w.
There is one small complication with using a dictionary here. The first time
we encounter a word, it will not yet be in counts. Attempting to access a non-
existent key produces a run-time KeyError. To guard against this, we need a
decision in our algorithm:
if w is already in counts:
add one to the count for w
else:
set count for w to 1
This decision ensures that the first time a word is encountered, it will be entered
into the dictionary with a count of 1.
One way to implement this decision is to use the in operator:
if w in counts:
counts [w] = counts [w] + 1
else:
counts [w] = 1
A more elegant approach is to use the get method:
counts [w] counts.get (w,0) + 1
If w is not already in the dictionary, this get will return 0, and the result is that
the entry for w is set to 1.
The dictionary updating code will form the heart of our program. We just
need to fill in the parts around it. We will need to split our text document into a
sequence of words. Before splitting, though, it's useful to convert all the text to
lowercase (so occurrences of "Foo" match "foo") and eliminate punctuation (so
"foo," matches "foo"). Here's the code to do those three tasks:
fname = input ("File to analyze: ")
#read file as one long string
text= open(fname, "r").read()
#convert all letters to lower case
text= text.lower()
J
A
![>>> list (passwd.keys())
['turing', 'bill', 'newuser', 'guido']
>>> list (passwd.values())
['genius', 'bluescreen', 'ImANewbie', 'superprogrammer']
>>> list (passwd.items())
[('turing', 'genius'), ('bill', 'bluescreen'),\
('newuser', 'ImANewbie'), ('guido', 'superprogrammer')]
>>> "bill" in passwd
True
>>> 'fred' in passwd
False
>>> passwd.get('bill', 'unknown')
'bluescreen'
>>> passwd.get('john', 'unknown')
unknown'
>>> passwd.clear()
>>> passwd
11.7.3 Example Program: Word Frequency
Let's write a program that analyzes text documents and counts how many times
each word appears in the document. This kind of analysis is sometimes used
as a crude measure of the style similarity between two documents and is also
used by automatic indexing and archiving programs (such as Internet search
engines).
At the highest level, this is just a multi-accumulator problem. We need a
count for each word that appears in the document. We can use a loop that iter-
ates through each word in the document and adds one to the appropriate count.
The only catch is that we will need hundreds or thousands of accumulators, one
for each unique word in the document. This is where a Python dictionary comes
in handy.
We will use a dictionary where the keys are strings representing words in the
document and the values are ints that count how many times the word appears.
Let's call our dictionary counts. To update the count for a particular word, w,
we just need a line of code something like this:
counts [w]
= counts [w] + 1
W
Q
1
1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F664a59d7-783e-42dd-9b24-7e03a0a10493%2F95fd7033-d541-4f94-92a3-27d6942a5a3a%2Fj654q6_processed.jpeg&w=3840&q=75)
Transcribed Image Text:>>> list (passwd.keys())
['turing', 'bill', 'newuser', 'guido']
>>> list (passwd.values())
['genius', 'bluescreen', 'ImANewbie', 'superprogrammer']
>>> list (passwd.items())
[('turing', 'genius'), ('bill', 'bluescreen'),\
('newuser', 'ImANewbie'), ('guido', 'superprogrammer')]
>>> "bill" in passwd
True
>>> 'fred' in passwd
False
>>> passwd.get('bill', 'unknown')
'bluescreen'
>>> passwd.get('john', 'unknown')
unknown'
>>> passwd.clear()
>>> passwd
11.7.3 Example Program: Word Frequency
Let's write a program that analyzes text documents and counts how many times
each word appears in the document. This kind of analysis is sometimes used
as a crude measure of the style similarity between two documents and is also
used by automatic indexing and archiving programs (such as Internet search
engines).
At the highest level, this is just a multi-accumulator problem. We need a
count for each word that appears in the document. We can use a loop that iter-
ates through each word in the document and adds one to the appropriate count.
The only catch is that we will need hundreds or thousands of accumulators, one
for each unique word in the document. This is where a Python dictionary comes
in handy.
We will use a dictionary where the keys are strings representing words in the
document and the values are ints that count how many times the word appears.
Let's call our dictionary counts. To update the count for a particular word, w,
we just need a line of code something like this:
counts [w]
= counts [w] + 1
W
Q
1
1
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
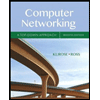
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
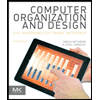
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
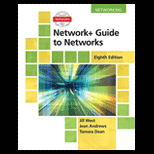
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
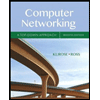
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
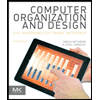
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
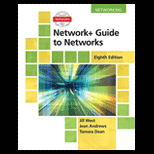
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
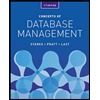
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
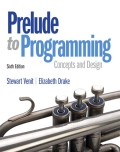
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
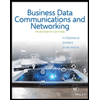
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY