2. Copy the previous program to a new file. a. Modify the Cake class. 1. Change topping from a single value to a dynamic array of toppings. 2. Update all code to reflect the change.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
previous code
//include the required header files
#include<iostream>
using namespace std;
//define first class Food
class Food
{
//public access
public:
//class member name
string name;
//define the constructor
Food()
{
// do Nothing
}
//accessor
string getName()
{
//return the value of name
return name;
}
//mutator
void setName(string name)
{
//set the name
this->name = name;
}
//copy constructor
Food(Food &f)
{
//set the value of name
this->name = f.name;
}
//parameterized constructor
Food(string name)
{
//set the value of name
this->name = name;
}
//destructor
~Food()
{
}
//assignment overload
void operator=(const Food &f )
{
//set the name
name = f.name;
}
//define the method virtual
virtual void output()
{
//print the name
cout<<"Food name: " <<this->name<<endl;
}
};
//define class Cake
class Cake : public Food
{
//public access
public:
//declare string variable toping
string topping ;
//constructor
Cake()
{
// Nothing
}
//define accessor
string getTopping ()
{
//return the value of topping
return topping ;
}
//define mutator
void setTopping (string name)
{
//set the value of topping
this->topping = topping ;
}
//copy constructor
Cake(Cake &c)
{
//inherited class member name
this->name = c.name;
//set the value of topping
this->topping = c.topping ;
}
//parameterized constructor
Cake(string food,string topping)
{
//set the value of name
this->name = food;
//set the value of topping
this->topping = topping ;
}
//destructor
~Cake()
{
}
//assignment overload
void operator=(const Cake &c )
{
//set the values
this->name = c.name;
this->topping = c.topping;
}
//define the method output
void output()
{
//print the name and topping
cout<<this->name <<"," <<this->topping<<endl;
}
};
//define main method
int main()
{
//define the object of Cake
Cake c1("Ice Cream Cake", "Chocolate Icing");
//call output()
c1.output();
//copy constructor
cout << "\ns2: Copy Constructor" << endl;
Cake c2(c1);
//print the output
c2.output();
//assignment overload
cout << "\ns3: Assignment Overload" << endl;
//define object c3 of Cake
Cake c3;
//assign c2 to c3
c3 = c2;
//call the method output using c3
c3.output();
//new line
cout << endl;
//return 0
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

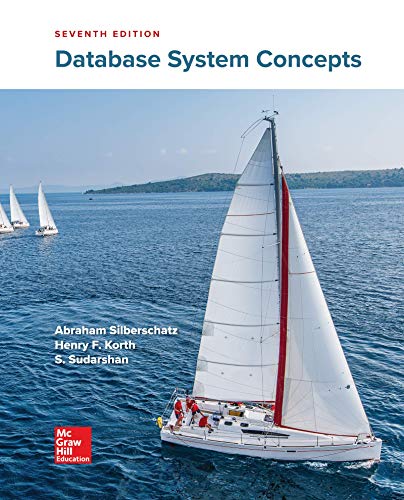
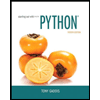
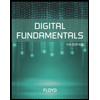
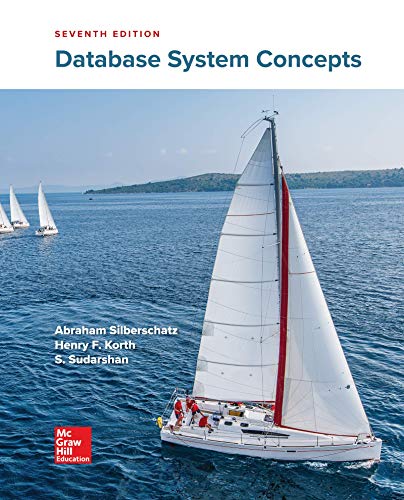
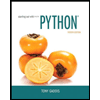
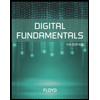
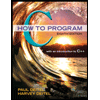
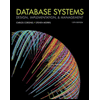
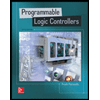