2. Number theory is a branch of mathematics that concerns itself with the properties of the integers. One function of considerable interest in number theory is the prime counting function. It is traditionally given the namen (but it has nothing to do with circles). For example n(6) = 3 because there are three prime numbers less than or equal to 6 (namely, 2, 3, and 5). Start by adding a function to package Number_Theory that computes t(n) for positive values n greater than or equal to 2. Note that the package already has a function Is_Prime that you will no doubt find useful. You might also want to make use of the defined subtype Prime_Argument_Type. Ada allows you to use Greek letters in variable names, but I suggest using the name Prime_Counting for n instead. 3. Modify the main file main.adb to exercise your function (ask the user to input a value n and then output r(n)). Here are some values of n(n) you can check. π(n) 10 4 100 25 1_000 168 10_000 1_229 100_000 9_592 1_000_000 78_498 10_000_000 664_579 100_000_000 5_761_455 1_000_000_000 50_847_534|
C code is not helping me
This code is in the Ada programming language
please give your answer with Ada code
Otherwise, it will just confuse me more
Below is Number_Theory.adb
with Ada.Numerics.Generic_Elementary_Functions;
package body Number_Theory is
-- Instantiate the library for floating point math using Floating_Type.
package Floating_Functions is new Ada.Numerics.Generic_Elementary_Functions(Floating_Type);
use Floating_Functions;
function Factorial(N : in Factorial_Argument_Type) return Positive is
begin
-- TODO: Finish me!
--
-- 0! is 1
-- N! is N * (N-1) * (N-2) * ... * 1
return 1;
end Factorial;
function Is_Prime(N : in Prime_Argument_Type) return Boolean is
Upper_Bound : Positive;
Current_Divisor : Prime_Argument_Type;
begin
-- Handle 2 as a special case.
if N = 2 then
return True;
end if;
Upper_Bound := N - 1;
Current_Divisor := 2;
while Current_Divisor < Upper_Bound loop
if N rem Current_Divisor = 0 then
return False;
end if;
Upper_Bound := N / Current_Divisor;
Current_Divisor := Current_Divisor + 1;
end loop;
return True;
end Is_Prime;
function Prime_Counting(N : in Prime_Argument_Type) return Natural is
begin
-- TODO: Finish me!
--
-- See the page for more information.
return 0;
end Prime_Counting;
function Logarithmic_Integral(N : in Prime_Argument_Type) return Floating_Type is
begin
-- TODO: Finish me!
--
-- See the page for more information.
return 1.0;
end Logarithmic_Integral;
end Number_Theory;
Below is Number_Theory.ads
-- This package contains subprograms for doing number theoretic computations.
package Number_Theory is
-- Constant Definitions
-----------------------
Gamma : constant := 0.57721_56649_01532_86060_65120_90082_40243_10421_59335_93992;
-- Type Definitions
-------------------
-- The range of values for which N! can be computed without overflow.
subtype Factorial_Argument_Type is Integer range 0 .. 12;
-- The range of values that might meaningfully be asked: are you prime?
subtype Prime_Argument_Type is Integer range 2 .. Integer'Last;
-- A floating point type with at least 15 significant decimal digits.
type Floating_Type is digits 15;
-- Subprogram Declarations
--------------------------
-- Returns N!
function Factorial(N : Factorial_Argument_Type) return Positive;
-- Returns True if N is prime; False otherwise.
function Is_Prime(N : in Prime_Argument_Type) return Boolean;
-- Returns the number of prime numbers less than or equal to N.
function Prime_Counting(N : in Prime_Argument_Type) return Natural;
-- The logarithmic integral function, which is an approximation of the prime counting function.
function Logarithmic_Integral(N : in Prime_Argument_Type) return Floating_Type;
end Number_Theory;
Below is main.adb
-- Some packages that we will need.
with Ada.Text_IO;
with Ada.Integer_Text_IO;
with Number_Theory;
-- Make the contents of the standard library packages "directly visible."
use Ada.Text_IO;
use Ada.Integer_Text_IO;
procedure Main is
-- We need to print values of type Number_Theory.Floating_Type, so generate a package for that.
package Floating_IO is new Ada.Text_IO.Float_IO(Number_Theory.Floating_Type);
use Floating_IO;
N : Number_Theory.Prime_Argument_Type;
-- Add any other local variables you might need here.
begin
Put_Line("Counting Primes!");
-- TODO: Finish me!
end Main;
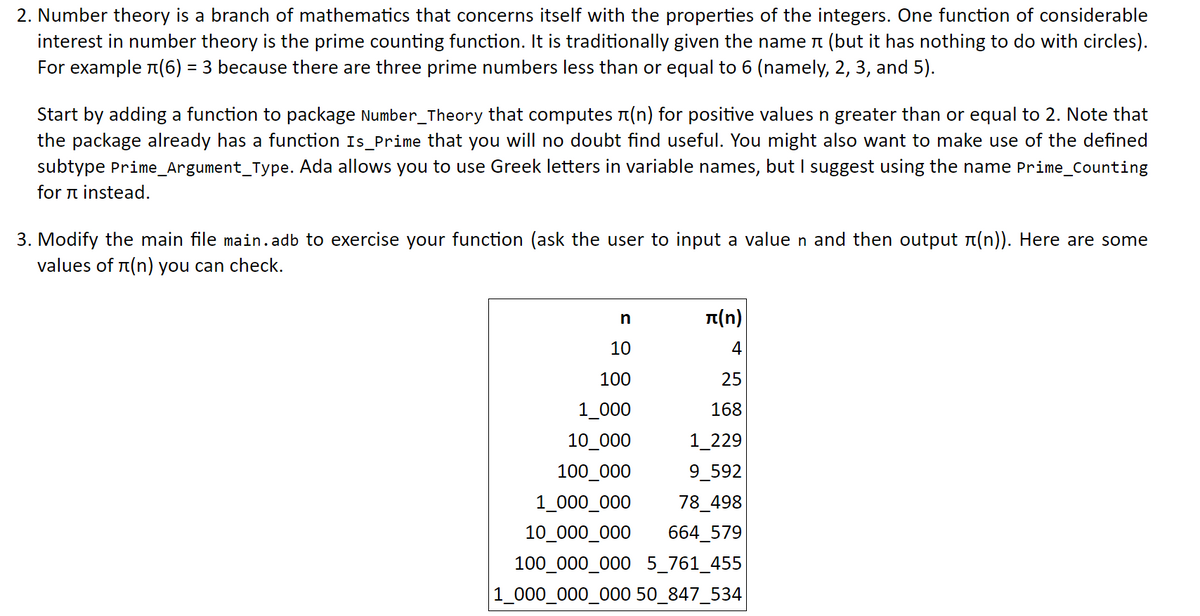

Step by step
Solved in 3 steps

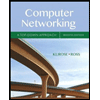
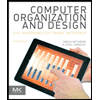
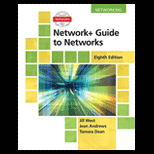
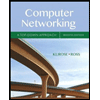
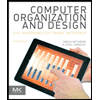
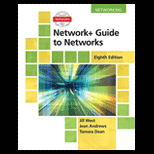
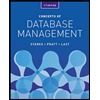
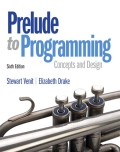
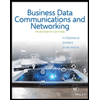