3 3 g 3 3 class Stack(): 3 3 3 3 3 3 3 3 3 3 3 3 3 # Declares an initially empty stack def __init__(self): 3 self.size = 0 self.stack = [] # Pushes an item to the top of the stack def push(self, item): self.stack.append(item) self.size += 1 # Removes the top item from the stack def pop (self): if (self size <= 0): print("You are attempting to delete from an empty stack.") print("In a proper code environment we would throw an exception here") else: self.size = 1 return self.stack.pop() # Returns the top item of the stack (without removing it) def peek(self): if (self, size == 0): print("Currently the stack is empty!") else: return self.stack[self.size 1] def balance_check(the_sequence): stack Stack() # Returns the size of the stack def get_size(self): return self.size # Tostring method for the stack def_str__(self): result = "" for i in range (len(self.stack) - 1,-1, -1): result += "Item %d: %s\n" % ((i + 1), self.stack[i]) return result for j in the sequence: if j not in ["(", ")"]: return "Fail precondition check" if len(the sequence) == 0: return "Fail precondition check" for i in the sequence: if i in ["("]: stack.push(i) elif i in [")"]: if stack.get_size() == 0: return False elif stack.get_size() > 0: stack.pop() if stack.get_size() == 0: return True elif stack.get_size() != 0: return False
3 3 g 3 3 class Stack(): 3 3 3 3 3 3 3 3 3 3 3 3 3 # Declares an initially empty stack def __init__(self): 3 self.size = 0 self.stack = [] # Pushes an item to the top of the stack def push(self, item): self.stack.append(item) self.size += 1 # Removes the top item from the stack def pop (self): if (self size <= 0): print("You are attempting to delete from an empty stack.") print("In a proper code environment we would throw an exception here") else: self.size = 1 return self.stack.pop() # Returns the top item of the stack (without removing it) def peek(self): if (self, size == 0): print("Currently the stack is empty!") else: return self.stack[self.size 1] def balance_check(the_sequence): stack Stack() # Returns the size of the stack def get_size(self): return self.size # Tostring method for the stack def_str__(self): result = "" for i in range (len(self.stack) - 1,-1, -1): result += "Item %d: %s\n" % ((i + 1), self.stack[i]) return result for j in the sequence: if j not in ["(", ")"]: return "Fail precondition check" if len(the sequence) == 0: return "Fail precondition check" for i in the sequence: if i in ["("]: stack.push(i) elif i in [")"]: if stack.get_size() == 0: return False elif stack.get_size() > 0: stack.pop() if stack.get_size() == 0: return True elif stack.get_size() != 0: return False
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Hi, could you tell me the big O notation of the balance_check function
![3
class Stack():
# Declares an initially empty stack
def __init__(self):
self.size = 0
self.stack = []
# Pushes an item to the top of the stack
def push(self, item):
self.stack.append(item)
self.size += 1
# Removes the top item from the stack
def pop (self):
if (self-size <=
print("You are attempting to delete from an empty stack.")
print("In a proper code environment we would throw an exception here")
else:
self.size -= 1
return self.stack.pop()
# Returns the top item of the stack (without removing it)
def peek(self):
if (self.size == 0):
print("Currently the stack is empty!")
else:
return self.stack[self.size - 1]
# Returns the size of the stack
def get_size(self):
return self.size
# Tostring method for the stack
def __str__(self):
result = ""
for i in range (len(self.stack) - 1,-1, -1):
result += "Item %d: %s\n" % ((i + 1), self.stack[i])
return result
def balance_check(the_sequence):
stack = Stack()
for j in the sequence:
if j not in ["(", ")"]:
return "Fail precondition check"
if len(the_sequence) == 0:
return "Fail precondition check"
for i in the sequence:
if i in ["("]:
stack.push(i)
elif i in [")"]:
if stack.get_size() == 0:
return False
elif stack.get_size() > 0:
stack.pop()
if stack.get_size() == 0:
return True
elif stack.get_size() != 0:
return False](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F442cdeaf-d6f5-4c90-9eca-defd0049fe9f%2F19c01b4f-b142-402a-94e5-13ce54a0af95%2Fv0pisk_processed.png&w=3840&q=75)
Transcribed Image Text:3
class Stack():
# Declares an initially empty stack
def __init__(self):
self.size = 0
self.stack = []
# Pushes an item to the top of the stack
def push(self, item):
self.stack.append(item)
self.size += 1
# Removes the top item from the stack
def pop (self):
if (self-size <=
print("You are attempting to delete from an empty stack.")
print("In a proper code environment we would throw an exception here")
else:
self.size -= 1
return self.stack.pop()
# Returns the top item of the stack (without removing it)
def peek(self):
if (self.size == 0):
print("Currently the stack is empty!")
else:
return self.stack[self.size - 1]
# Returns the size of the stack
def get_size(self):
return self.size
# Tostring method for the stack
def __str__(self):
result = ""
for i in range (len(self.stack) - 1,-1, -1):
result += "Item %d: %s\n" % ((i + 1), self.stack[i])
return result
def balance_check(the_sequence):
stack = Stack()
for j in the sequence:
if j not in ["(", ")"]:
return "Fail precondition check"
if len(the_sequence) == 0:
return "Fail precondition check"
for i in the sequence:
if i in ["("]:
stack.push(i)
elif i in [")"]:
if stack.get_size() == 0:
return False
elif stack.get_size() > 0:
stack.pop()
if stack.get_size() == 0:
return True
elif stack.get_size() != 0:
return False
Expert Solution

Step 1
Stack:
A data structure that adheres to the Last In First Out (LIFO) concept is the stack. This means that the last element that was added to the stack will be the first one to be removed. It is typically used when a sequence of operations needs to be performed in a specific order. It can also be used when data needs to be accessed in a specific order, such as when a recursive algorithm is used. The basic operations of a stack are push, which adds an element to the top of the stack, and pop, which removes the element from the top of the stack.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
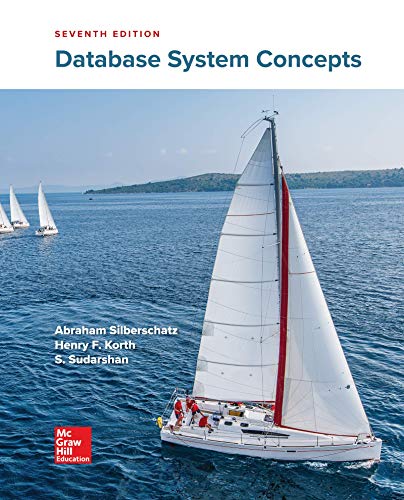
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
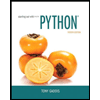
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
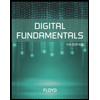
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
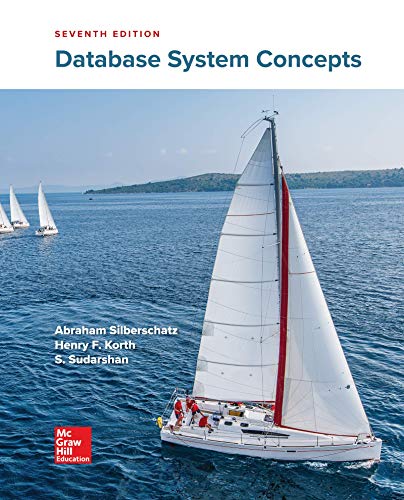
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
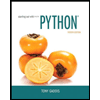
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
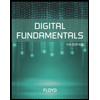
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
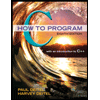
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
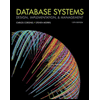
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
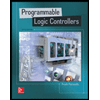
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education