3.11 (Modified Account Class) Modify class Account (Fig. 3.8) to provide a method called with- draw that withdraws money from an Account. Ensure that the withdrawal amount does not exceed the Account's balance. If it does, the balance should be left unchanged and the method should print a message indicating "withdrawal amount exceeded account balance." Modify class AccountTest (Fig. 3.9) to test method wi thdraw.
3.11 (Modified Account Class) Modify class Account (Fig. 3.8) to provide a method called with- draw that withdraws money from an Account. Ensure that the withdrawal amount does not exceed the Account's balance. If it does, the balance should be left unchanged and the method should print a message indicating "withdrawal amount exceeded account balance." Modify class AccountTest (Fig. 3.9) to test method wi thdraw.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter9: Records (struct)
Section: Chapter Questions
Problem 12SA
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question

Transcribed Image Text:3.11 (Modified Account Class) Modify class Account (Fig. 3.8) to provide a method called wi th-
draw that withdraws money from an Account. Ensure that the withdrawal amount does not exceed
the Account's balance. If it does, the balance should be left unchanged and the method should print
a message indicating "Withdrawal amount exceeded account balance." Modify class AccountTest
(Fig. 3.9) to test method withdraw.
![I // Fig. 3.8: Account.java
// Account class with a double instance variable balance and a constructor
// and deposit method that perform validation.
2.
4
5 public class Account {
private String name; // instance variable
private double balance; // instance variable
6
7
8
// Account constructor that receives two parameters
public Account(String name, double balance) {
this.name = name; // assign name to instance vari able name
9
10
12
// validate that the balance is greater than 0.0; if it's not,
// instance variable balance keeps its default initial value of 0.0
if (balance > 0.0) { // if the balance is valid
this.balance = balance; // assign it to instance variable balance
13
14
15
16
17
18
19
// method that deposits (adds) only a valid amount to the balance
public void deposit(double depositAmount) {
if (depositAmount > 0.0) { // if the depositAmount is valid
balance = balance + depositAmount; // add it to the balance
20
21
22
23
24
25
// Fig. 3.9: AccountTest.java
// Inputting and outputting floating-point numbers with Account objects.
3 import java.util.Scanner;
4
public class AccountTest {
public static void main(String[] args) {
Account accountl = new Account("Jane Green", 50.00);
Account account2 = new Account("John Blue", -7.53);
// display initial balance of each object
System.out.printf("%s balance: $%.2f%n",
accountl.getName (), accountl.getBalance():
System.out.printf("%s balance: $%.2f%n%n",
account2.getName(), account2.getBalance());
10
%3D
12
13
14
15
// create a Scanner to obtain input from the command window
Scanner input = new Scanner(System.in);
16
17
18
19
System.out.print("Enter deposit amount for accountl: "); // prompt
double depositAmount = input.nextDouble(); // obtain user input
System.out.printf("%nadding %.2f to accountl balance%n%n",
depositAmount);
accountl.deposit(depositAmount); // add to accountl's balance
20
21
22
23
24
// display balances
System.out.printf("%s balance: $%.2f%n",
accountl.getName(), accountl.getBalance(0);
System.out.printf("%s balance: $%.2f%n%n",
account2.getName (), account2.getBalance());
25
26
27
28
29
30
31
System.out.print("Enter deposit amount for account2: "); // prompt
depositAmount = input.nextDouble(); // obtain user input
System.out.printf("%nadding %.2f to account2 balance%n%n",
depositAmount);
account2.deposit(depositAmount); // add to account2 balance
32
33
34
35
36
// display balances
System.out.printf("%s balance: $%.2f%n",
account1.getName (), accountl.getBalance();
System.out.printf("%s balance: $%.2f%n%n",
account2.getName(), account2.getBalance());
37
38
39
40
41
42 }
43 }](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff89e551b-b1f1-4813-9e4c-059c3e255e20%2F81a22ed8-861a-4c7a-969c-d9f8d01e7cd0%2Fginvpq_processed.png&w=3840&q=75)
Transcribed Image Text:I // Fig. 3.8: Account.java
// Account class with a double instance variable balance and a constructor
// and deposit method that perform validation.
2.
4
5 public class Account {
private String name; // instance variable
private double balance; // instance variable
6
7
8
// Account constructor that receives two parameters
public Account(String name, double balance) {
this.name = name; // assign name to instance vari able name
9
10
12
// validate that the balance is greater than 0.0; if it's not,
// instance variable balance keeps its default initial value of 0.0
if (balance > 0.0) { // if the balance is valid
this.balance = balance; // assign it to instance variable balance
13
14
15
16
17
18
19
// method that deposits (adds) only a valid amount to the balance
public void deposit(double depositAmount) {
if (depositAmount > 0.0) { // if the depositAmount is valid
balance = balance + depositAmount; // add it to the balance
20
21
22
23
24
25
// Fig. 3.9: AccountTest.java
// Inputting and outputting floating-point numbers with Account objects.
3 import java.util.Scanner;
4
public class AccountTest {
public static void main(String[] args) {
Account accountl = new Account("Jane Green", 50.00);
Account account2 = new Account("John Blue", -7.53);
// display initial balance of each object
System.out.printf("%s balance: $%.2f%n",
accountl.getName (), accountl.getBalance():
System.out.printf("%s balance: $%.2f%n%n",
account2.getName(), account2.getBalance());
10
%3D
12
13
14
15
// create a Scanner to obtain input from the command window
Scanner input = new Scanner(System.in);
16
17
18
19
System.out.print("Enter deposit amount for accountl: "); // prompt
double depositAmount = input.nextDouble(); // obtain user input
System.out.printf("%nadding %.2f to accountl balance%n%n",
depositAmount);
accountl.deposit(depositAmount); // add to accountl's balance
20
21
22
23
24
// display balances
System.out.printf("%s balance: $%.2f%n",
accountl.getName(), accountl.getBalance(0);
System.out.printf("%s balance: $%.2f%n%n",
account2.getName (), account2.getBalance());
25
26
27
28
29
30
31
System.out.print("Enter deposit amount for account2: "); // prompt
depositAmount = input.nextDouble(); // obtain user input
System.out.printf("%nadding %.2f to account2 balance%n%n",
depositAmount);
account2.deposit(depositAmount); // add to account2 balance
32
33
34
35
36
// display balances
System.out.printf("%s balance: $%.2f%n",
account1.getName (), accountl.getBalance();
System.out.printf("%s balance: $%.2f%n%n",
account2.getName(), account2.getBalance());
37
38
39
40
41
42 }
43 }
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
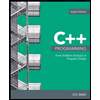
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
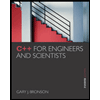
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
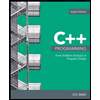
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
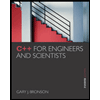
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr