4. Define a concrete dlass Sphere which extends the Shape class. a. It has radius field of type double. b. Provide single constructor that takes two input parameters: name and radius resp. C. Provide the accessor method. d. Implement the unimplemented method getArea() so that it retums the surface area of the sphere object. e. Override tostring() method so that it retums the following information: L (type): (name) - [(radius)]" e.g., sphere: my_sphere_1 - [3.0] 5. Now imagine you are given an array of different shapes and are required to sort them based on the area. a. Define a static method sort in the class ShapeUtil that will sort the array of different Shape objects. (You may use any sorting algorithm, just make sure you implement it yourself) b. Define another static method printShapes in the class ShapeUtil that prints an array of Shape objects in a single row. Consider using toString() methods of those. 6. The customer is willing to paint all the Shape objects. If he provides us with the number of liters of paint per can and the number of cans he bought, could you define a method (static method getPaintedShape in dass PaintManager] that takes a. the array of shapes b. the volume of a single can c. the number of cans and return the shapes that will be completely painted. d. If the amount of paint is not sufficient, he desires to paint as many shapes as possible. 7. Customer also need you to provide her with the following functionality: a. As there are many objects and she likes to name each of them, sometimes she forgets about some. For simplicity, assume that the names are unique (there are no two objects with the same name) b. Define a method (findShapeByName in the class ShapeUtil which will take an array of Shape objects and a String name, which returns L the Shape object with that name if exists or null if there are no object with that name.
4. Define a concrete dlass Sphere which extends the Shape class. a. It has radius field of type double. b. Provide single constructor that takes two input parameters: name and radius resp. C. Provide the accessor method. d. Implement the unimplemented method getArea() so that it retums the surface area of the sphere object. e. Override tostring() method so that it retums the following information: L (type): (name) - [(radius)]" e.g., sphere: my_sphere_1 - [3.0] 5. Now imagine you are given an array of different shapes and are required to sort them based on the area. a. Define a static method sort in the class ShapeUtil that will sort the array of different Shape objects. (You may use any sorting algorithm, just make sure you implement it yourself) b. Define another static method printShapes in the class ShapeUtil that prints an array of Shape objects in a single row. Consider using toString() methods of those. 6. The customer is willing to paint all the Shape objects. If he provides us with the number of liters of paint per can and the number of cans he bought, could you define a method (static method getPaintedShape in dass PaintManager] that takes a. the array of shapes b. the volume of a single can c. the number of cans and return the shapes that will be completely painted. d. If the amount of paint is not sufficient, he desires to paint as many shapes as possible. 7. Customer also need you to provide her with the following functionality: a. As there are many objects and she likes to name each of them, sometimes she forgets about some. For simplicity, assume that the names are unique (there are no two objects with the same name) b. Define a method (findShapeByName in the class ShapeUtil which will take an array of Shape objects and a String name, which returns L the Shape object with that name if exists or null if there are no object with that name.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
// define the interface
interface Measurable {
public abstract double getArea();
}
// create an abstract class shape
abstract class Shape implements Measurable {
String type;
String name;
// constructor of Shape class
Shape(String name) {
this.name = name;
}
// accessor for type
public String getType() {
return type;
}
// accessor for name
public String name() {
return name;
}
// setter for name
public void setName(String name) {
this.name = name;
}
// define toString method
public String toString() {
return (type + " : " + name);
}
}
// define Rectangle class that extends Shape class
class Rectangle extends Shape {
double width;
double height;
Rectangle(String name, double width, double height) {
super(name);
this.type = "rectangle";
this.width = width;
this.height = height;
}
public double getWidth() {
return width;
}
public double getHeight() {
return height;
}
public double getArea() {
return width * height;
}
public String toString() {
return (type + " : " + name + " - [" + width + " , " + height + "]");
}
}
![4. Define a concrete dass Sphere which extends the Shape class.
a. It has radius field of type double.
b. Provide single constructor that takes two input parameters: name and radius resp.
c. Provide the accessor method.
d. Implement the unimplemented method getArea() so that it returms the surface area of
the sphere object.
e. Override toString() method so that it returms the following information:
i
"(type): (name) - [(radius}]" e.g., sphere: my_sphere_1- [3.0]
5. Now imagine you are given an array of different shapes and are required to sort them based
on the area.
a. Define a static method sort in the cdass ShapeUtil that will sort the array of different
Shape objects. (You may use any sorting algorithm, just make sure you implement it
yourself)
b. Define another static method printShapes in the class ShapeUtil that prints an array of
Shape objects in a single row. Consider using toString() methods of those.
6. The customer is willing to paint all the Shape objects. If he provides us with the number of
liters of paint per can and the number of cans he bought, could you define a method [static
method getPaintedShape in cass PaintManager] that takes
a. the array of shapes
b. the volume of a single can
c. the number of cans
and return the shapes that will be completely painted.
d. If the amount of paint is not sufficient, he desires to paint as many shapes as possible.
7. Customer also need you to provide her with the following functionality:
a. As there are many objects and she likes to name each of them, somefimes she forgets
about some. For simplicity, assume that the names are unique (there are no two objects
with the same name)
b. Define a method [findShapeByName in the class ShapeUtil which will take an array of
Shape objects and a String name, which returns
i the Shape object with that name if exists or
i. null if there are no object with that name.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4da1d97d-b676-4731-b282-a828a767be39%2F109a66cd-7e92-4b64-8a17-36254428daf5%2Fd0ysy6s_processed.png&w=3840&q=75)
Transcribed Image Text:4. Define a concrete dass Sphere which extends the Shape class.
a. It has radius field of type double.
b. Provide single constructor that takes two input parameters: name and radius resp.
c. Provide the accessor method.
d. Implement the unimplemented method getArea() so that it returms the surface area of
the sphere object.
e. Override toString() method so that it returms the following information:
i
"(type): (name) - [(radius}]" e.g., sphere: my_sphere_1- [3.0]
5. Now imagine you are given an array of different shapes and are required to sort them based
on the area.
a. Define a static method sort in the cdass ShapeUtil that will sort the array of different
Shape objects. (You may use any sorting algorithm, just make sure you implement it
yourself)
b. Define another static method printShapes in the class ShapeUtil that prints an array of
Shape objects in a single row. Consider using toString() methods of those.
6. The customer is willing to paint all the Shape objects. If he provides us with the number of
liters of paint per can and the number of cans he bought, could you define a method [static
method getPaintedShape in cass PaintManager] that takes
a. the array of shapes
b. the volume of a single can
c. the number of cans
and return the shapes that will be completely painted.
d. If the amount of paint is not sufficient, he desires to paint as many shapes as possible.
7. Customer also need you to provide her with the following functionality:
a. As there are many objects and she likes to name each of them, somefimes she forgets
about some. For simplicity, assume that the names are unique (there are no two objects
with the same name)
b. Define a method [findShapeByName in the class ShapeUtil which will take an array of
Shape objects and a String name, which returns
i the Shape object with that name if exists or
i. null if there are no object with that name.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
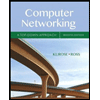
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
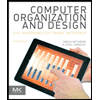
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
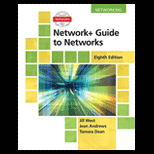
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
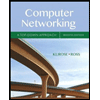
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
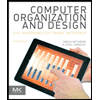
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
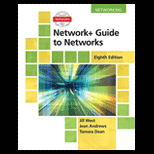
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
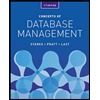
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
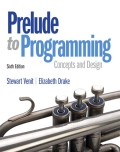
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
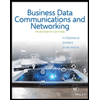
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY