8.12 (Simulation: The Tortoise and the Hare) In this exercise, you’ll re-create the classic race of the tortoise and the hare. You’ll use random-number generation to develop a simulation of this memorable event. Our contenders begin the race at “square 1” of 70 squares. Each square represents a possible position along the race course. The finish line is at square 70. The first contender to reach or pass square 70 is rewarded with a pail of fresh carrots and lettuce. The course weaves its way up the side of a slippery mountain, so occasionally the contenders lose ground. There is a clock that ticks once per second. With each tick of the clock, your program should use function moveTortoise and moveHare to adjust the position of the animals according to the rules in Fig. 8.18 (view your book for the rules in the table). These functions should use pointer-based pass-by-reference to modify the position of the tortoise and the hare. Use variables to keep track of the positions of the animals (i.e., position numbers are 1–70). Start each animal at position 1 (i.e., the “starting gate”). If an animal slips left before square 1, move the animal back to square 1. Generate the percentages in Fig. 8.18 by producing a random integer i in the range 1≤i≤10. For the tortoise, perform a “fast plod” when 1≤i≤5, a “slip” when 6≤i≤7 or a “slow plod” when 8≤i≤10. Use a similar technique to move the hare. Begin the race by displaying BANG !!!!! AND THEY'RE OFF !!!!! For each tick of the clock (i.e., each iteration of a loop), display a 70-position line showing the letter T in the tortoise’s position and the letter H in the hare’s position. Occasionally, the contenders land on the same square. In this case, the tortoise bites the hare and your program should display OUCH!!! beginning at that position. All positions other than the T, the H or the OUCH!!! (in case of a tie) should be blank. After displaying each line, test whether either animal has reached or passed square 70. If so, display the winner and terminate the simulation. If the tortoise wins, display TORTOISE WINS!!! YAY!!! If the hare wins, display Hare wins. Yuch. If both animals win on the same clock tick, you may want to favor the tortoise (the “underdog”), or you may want to display It's a tie. If neither animal wins, perform the loop again to simulate the next tick of the clock.
8.12 (Simulation: The Tortoise and the Hare) In this exercise, you’ll re-create the classic race of the tortoise and the hare.
You’ll use random-number generation to develop a simulation of this memorable event.
Our contenders begin the race at “square 1” of 70 squares. Each square represents a possible position along the race course. The finish line is at square 70. The first contender to reach or pass square 70 is rewarded with a pail of fresh carrots and lettuce. The course weaves its way up the side of a slippery mountain, so occasionally the contenders lose ground.
There is a clock that ticks once per second. With each tick of the clock, your program should use function moveTortoise and moveHare to adjust the position of the animals according to the rules in Fig. 8.18 (view your book for the rules in the table). These functions should use pointer-based pass-by-reference to modify the position of the tortoise and the hare.
Use variables to keep track of the positions of the animals (i.e., position numbers are 1–70). Start each animal at position 1 (i.e., the “starting gate”). If an animal slips left before square 1, move the animal back to square 1.
Generate the percentages in Fig. 8.18 by producing a random integer i in the range 1≤i≤10. For the tortoise, perform a “fast plod” when 1≤i≤5, a “slip” when 6≤i≤7 or a “slow plod” when 8≤i≤10. Use a similar technique to move the hare.
Begin the race by displaying
BANG !!!!!
AND THEY'RE OFF !!!!!
For each tick of the clock (i.e., each iteration of a loop), display a 70-position line showing the letter T in the tortoise’s position and the letter H in the hare’s position. Occasionally, the contenders land on the same square. In this case, the tortoise bites the hare and your program should display OUCH!!! beginning at that position. All positions other than the T, the H or the OUCH!!! (in case of a tie) should be blank.
After displaying each line, test whether either animal has reached or passed square 70. If so, display the winner and terminate the simulation. If the tortoise wins, display TORTOISE WINS!!! YAY!!! If the hare wins, display Hare wins. Yuch. If both animals win on the same clock tick, you may want to favor the tortoise (the “underdog”), or you may want to display It's a tie. If neither animal wins, perform the loop again to simulate the next tick of the clock.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Please share another code. This code is not compiling and it gives lots of errors.
Kindly see some of the errors below;
/usr/include/c++/4.8.2/bits/basic_string.h:2830:22: note: cannot convert ‘__idx’ (type ‘size_t* {aka long unsigned int*}’) to type ‘int*’
__idx, __base); }
^
/usr/include/c++/4.8.2/bits/basic_string.h: In function ‘long unsigned int std::stoul(const string&, size_t*, int)’:
/usr/include/c++/4.8.2/bits/basic_string.h:2835:22: error: no matching function for call to ‘__stoa(long unsigned int (*)(const char*, char**, int)throw (), const char [6], const char*, size_t*&, int&)’
__idx, __base); }
^
/usr/include/c++/4.8.2/bits/basic_string.h:2835:22: note: candidate is:
In file included from /usr/include/c++/4.8.2/bits/basic_string.h:2815:0,
from /usr/include/c++/4.8.2/string:52,
from /usr/include/c++/4.8.2/random:41,
from challenge4.cpp:4:
/usr/include/c++/4.8.2/ext/string_conversions.h:54:5: note: template<class _TRet, class _Ret, class _CharT, class ... _Base> _Ret __gnu_cxx::__stoa(_TRet (*)(const _CharT*, _CharT**, _Base ...), const char*, const _CharT*, int*, _Base ...)
__stoa(_TRet (*__convf) (const _CharT*, _CharT**, _Base...),
^
/usr/include/c++/4.8.2/ext/string_conversions.h:54:5: note: template argument deduction/substitution failed:
In file included from /usr/include/c++/4.8.2/string:52:0,
from /usr/include/c++/4.8.2/random:41,
from challenge4.cpp:4:
/usr/include/c++/4.8.2/bits/basic_string.h:2835:22: note: cannot convert ‘__idx’ (type ‘size_t* {aka long unsigned int*}’) to type ‘int*’
__idx, __base); }
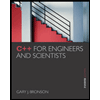
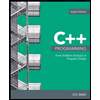
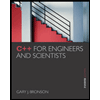
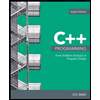