9 from breezypythongui import EasyFrame 10 11 class TemperatureConverter(EasyFrame): 12 """A termperature conversion program.""" 13 14 def -_init__(self): 15 I""Sets up the window and widgets.""" 16 EasyFrame._init__(self, title = "Temperature Converter") 17 18 # Label and field for Celsius 19 self.addLabel(text = "Celsius", 20 row = 0, column = 0) 21 self.celsiusField = self.addFloatField(value = 0.0, row = 1, column = 0, 22 23 24 precision = 2) 25 # Label and field for Fahrenheit self.addLabel(text = "Fahrenheit", 26 27 28 row = 0, column = 1) 29 self.fahrField = self.addFloatField(value = 32.0, 30 row = 1, 31 column = 1, 32 precision = 2) 33 34 # Celsius to Fahrenheit button 35 self.addButton(text = ">>>»", row = 2, column = 0, command = self.computeFahr) 36 37 38 39 # Fahrenheit to Celsius button 40 self.addButton(text = "<«««", row = 2, column = 1, command = self.computeCelsius) 41 42 43 44 # The controller methods def computeFahr(self): " "Inputs the Celsius degrees 45 46 and outputs the Fahrenheit degrees.""" degrees = self.celsiusField.getNumber() degrees = degrees * 9 / 5 + 32 self.fahrField.setNumber(degrees) 47 48 49 50 51 def computeCelsius(self): ""Inputs the Fahrenheit degrees 52 53 and outputs the Celsius degrees.""" degrees = self.fahrField.getNumber() degrees = (degrees - 32) * 5 / 9 self.celsiusField.setNumber(degrees) 54 55 56 57 58 59 def main(): 60 "Instantiate and pop up the window.""" 61 TemperatureConverter().mainloop() 62 63 if -_name__ == "-_main__": main() 64 65
9 from breezypythongui import EasyFrame 10 11 class TemperatureConverter(EasyFrame): 12 """A termperature conversion program.""" 13 14 def -_init__(self): 15 I""Sets up the window and widgets.""" 16 EasyFrame._init__(self, title = "Temperature Converter") 17 18 # Label and field for Celsius 19 self.addLabel(text = "Celsius", 20 row = 0, column = 0) 21 self.celsiusField = self.addFloatField(value = 0.0, row = 1, column = 0, 22 23 24 precision = 2) 25 # Label and field for Fahrenheit self.addLabel(text = "Fahrenheit", 26 27 28 row = 0, column = 1) 29 self.fahrField = self.addFloatField(value = 32.0, 30 row = 1, 31 column = 1, 32 precision = 2) 33 34 # Celsius to Fahrenheit button 35 self.addButton(text = ">>>»", row = 2, column = 0, command = self.computeFahr) 36 37 38 39 # Fahrenheit to Celsius button 40 self.addButton(text = "<«««", row = 2, column = 1, command = self.computeCelsius) 41 42 43 44 # The controller methods def computeFahr(self): " "Inputs the Celsius degrees 45 46 and outputs the Fahrenheit degrees.""" degrees = self.celsiusField.getNumber() degrees = degrees * 9 / 5 + 32 self.fahrField.setNumber(degrees) 47 48 49 50 51 def computeCelsius(self): ""Inputs the Fahrenheit degrees 52 53 and outputs the Celsius degrees.""" degrees = self.fahrField.getNumber() degrees = (degrees - 32) * 5 / 9 self.celsiusField.setNumber(degrees) 54 55 56 57 58 59 def main(): 60 "Instantiate and pop up the window.""" 61 TemperatureConverter().mainloop() 62 63 if -_name__ == "-_main__": main() 64 65
Chapter16: Graphics
Section: Chapter Questions
Problem 2PE
Related questions
Question
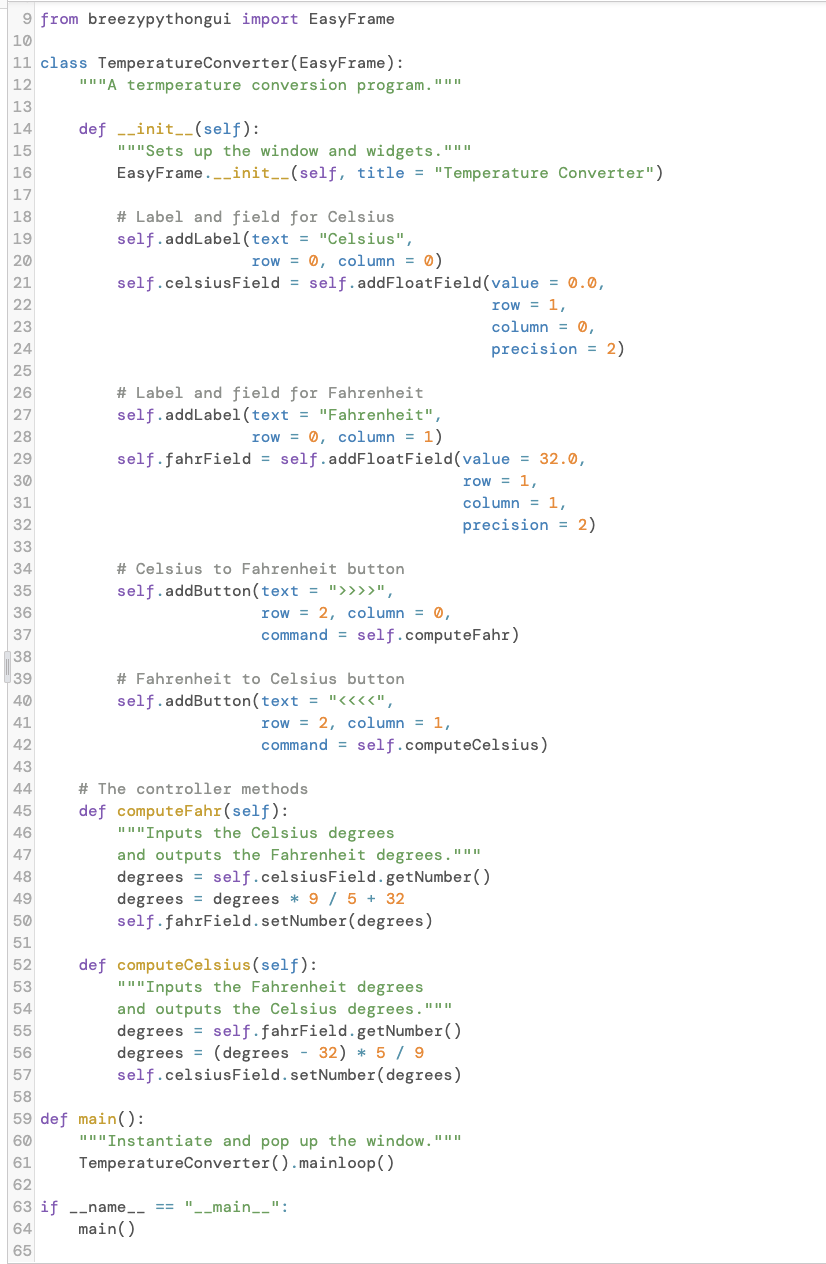
Transcribed Image Text:9 from breezypythongui import EasyFrame
10
11 class TemperatureConverter(EasyFrame):
12
""A termperature conversion program."""
II II II
13
def -_init__(self):
"I"Sets up the window and widgets."""
EasyFrame._init_-(self, title = "Temperature Converter")
14
15
II IIII
16
17
18
# Label and field for Celsius
self.addLabel(text = "Celsius",
19
20
row = 0, column = 0)
21
self.celsiusField = self.addFloatField(value = 0.0,
22
row = 1,
column = Ø,
23
24
precision = 2)
25
# Label and field for Fahrenheit
self.addLabel(text = "Fahrenheit",
26
27
28
row = 0, column = 1)
29
self.fahrField = self.addFloatField(value = 32.0,
30
row = 1,
31
column = 1,
32
precision = 2)
33
# Celsius to Fahrenheit button
self.addButton(text = ">>>>",
34
35
row = 2, column = 0,
command = self.computeFahr)
36
37
38
39
# Fahrenheit to Celsius button
40
self.addButton(text = "<«««",
41
row = 2, column = 1,
42
command = self.computeCelsius)
43
44
# The controller methods
def computeFahr(self):
"I"Inputs the Celsius degrees
45
46
and outputs the Fahrenheit degrees."""
degrees = self.celsiusField.getNumber()
degrees = degrees * 9 / 5 + 32
self.fahrField.setNumber(degrees)
47
II II II
48
49
50
51
def computeCelsius(self):
II"Inputs the Fahrenheit degrees
52
53
and outputs the Celsius degrees."""
degrees = self.fahrField.getNumber()
degrees = (degrees - 32) * 5 / 9
self.celsiusField.setNumber(degrees)
54
II II II
55
56
57
58
59 def main():
60
III"Instantiate and pop up the window."""
II II II
61
TemperatureConverter ().mainloop()
62
"_main__":
63 if --name_-
main()
==
64
65
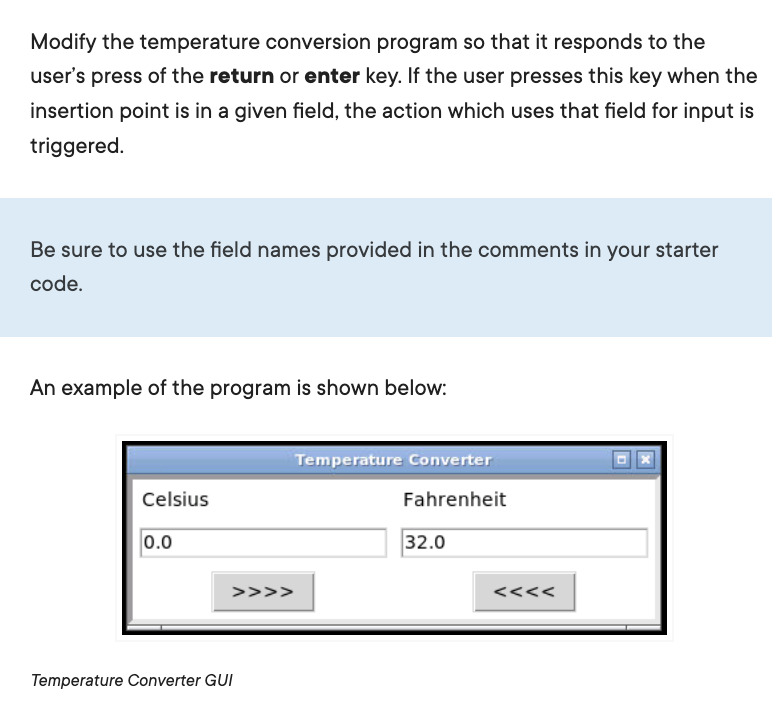
Transcribed Image Text:Modify the temperature conversion program so that it responds to the
user's press of the return or enter key. If the user presses this key when the
insertion point is in a given field, the action which uses that field for input is
triggered.
Be sure to use the field names provided in the comments in your starter
code.
An example of the program is shown below:
Temperature Converter
Celsius
Fahrenheit
0.0
32.0
>>>>
<<<<
Temperature Converter GUI
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
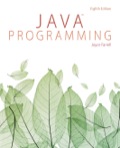
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
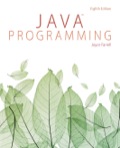
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage