A set of integers 0..MAX may be implemented using an array of boolean values. This particular implementation is called a bit-vector implementation of a Set. Since C doesn't have the Boolean data type, then substitute TRUE with 1 and FALSE with 0, or define 1 and 0 as TRUE and FALSE, respectively. For example, if the integer 3 is an element of the set, then the array element indexed by 3 is TRUE. On the other hand, if 3 is not an element, then the array element indexed by 3 is FALSE. For example: if s= (3,4,6,8), the array looks like this: 0 1 2 3 4 5 6 7 8 9 FALSE FALSE FALSE TRUE TRUE FALSE TRUE FALSE TRUE FALSE Implement a programmer-defined data type called BitSet to represent a set as follows: typedef int BitSet[MAX]; Implement the following functions: void initialize(BitSet s); set all array elements to FALSE void add(int elem, BitSet s); set the item indexed by elem to TRUE void display(BitSet s); display the set on the screen using set notation, e.g. (3,4,5,6) - this means that you will print the index value if the content of that cell is TRUE void getUnion(BitSet result, BitSet s1, BitSet s2); store in the array result the set resulting from the union of s1 and s2 x is an element of s1 union s2 if x is an element of s1 or x is an element of s2 void intersection (BitSet result, BitSet s1, BitSet s2); store in the array result the set resulting from the intersection of s1 and s2 -x is an element of s1 intersection s2 if x is an element of s1 and x is an element of s2 void difference(BitSet result, BitSet s1, BitSet s2); store in the array result the set resulting from the difference of s1 and s2 x is an element of s1 s2 if x is an element of s1 and x is not an element of s2 int is Empty(BitSet s); the set is empty of all array elements are false int contains(BitSet s,int elem); elem is an element of s if the array value indexed by elem is TRUE int disjoint(BitSet s1, BitSet s2); - two sets are disjoint if the intersection is empty int equal(BitSet s1, BitSet s2); - two sets are equal if they have exactly the same elements int cardinality (BitSet s); - the cardinality of the set is the number of TRUE elements int subset(BitSet s 1, BitSet s2); - s1 is a subset of s2 if all elements of s1 are in s2
A set of integers 0..MAX may be implemented using an array of boolean values. This particular implementation is called a bit-vector implementation of a Set. Since C doesn't have the Boolean data type, then substitute TRUE with 1 and FALSE with 0, or define 1 and 0 as TRUE and FALSE, respectively. For example, if the integer 3 is an element of the set, then the array element indexed by 3 is TRUE. On the other hand, if 3 is not an element, then the array element indexed by 3 is FALSE. For example: if s= (3,4,6,8), the array looks like this: 0 1 2 3 4 5 6 7 8 9 FALSE FALSE FALSE TRUE TRUE FALSE TRUE FALSE TRUE FALSE Implement a programmer-defined data type called BitSet to represent a set as follows: typedef int BitSet[MAX]; Implement the following functions: void initialize(BitSet s); set all array elements to FALSE void add(int elem, BitSet s); set the item indexed by elem to TRUE void display(BitSet s); display the set on the screen using set notation, e.g. (3,4,5,6) - this means that you will print the index value if the content of that cell is TRUE void getUnion(BitSet result, BitSet s1, BitSet s2); store in the array result the set resulting from the union of s1 and s2 x is an element of s1 union s2 if x is an element of s1 or x is an element of s2 void intersection (BitSet result, BitSet s1, BitSet s2); store in the array result the set resulting from the intersection of s1 and s2 -x is an element of s1 intersection s2 if x is an element of s1 and x is an element of s2 void difference(BitSet result, BitSet s1, BitSet s2); store in the array result the set resulting from the difference of s1 and s2 x is an element of s1 s2 if x is an element of s1 and x is not an element of s2 int is Empty(BitSet s); the set is empty of all array elements are false int contains(BitSet s,int elem); elem is an element of s if the array value indexed by elem is TRUE int disjoint(BitSet s1, BitSet s2); - two sets are disjoint if the intersection is empty int equal(BitSet s1, BitSet s2); - two sets are equal if they have exactly the same elements int cardinality (BitSet s); - the cardinality of the set is the number of TRUE elements int subset(BitSet s 1, BitSet s2); - s1 is a subset of s2 if all elements of s1 are in s2
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
(CODE THIS IN C)
DO NOT CODE IN C++
![A set of integers 0..MAX may be implemented using an array of boolean values. This
particular implementation is called a bit-vector implementation of a Set. Since C doesn't have
the Boolean data type, then substitute TRUE with 1 and FALSE with 0, or define 1 and 0 as
TRUE and FALSE, respectively.
For example, if the integer 3 is an element of the set, then the array element indexed by 3 is
TRUE. On the other hand, if 3 is not an element, then the array element indexed by 3 is
FALSE.
For example: if s= (3,4,6,8), the array looks like this:
0
1
2
3
4
5
6
7
8
9
FALSE FALSE FALSE TRUE TRUE FALSE TRUE FALSE TRUE FALSE
Implement a programmer-defined data type called BitSet to represent a set as follows:
typedef int BitSet[MAX];
Implement the following functions:
void initialize(BitSet s);
set all array elements to FALSE
void add(int elem, BitSet s);
set the item indexed by elem to TRUE
void display(BitSet s);
display the set on the screen using set notation, e.g. (3,4,5,6)
- this means that you will print the index value if the content of that cell is TRUE
void getUnion(BitSet result, BitSet s1, BitSet s2);
store in the array result the set resulting from the union of s1 and s2
x is an element of s1 union s2 if x is an element of s1 or x is an element of s2
void intersection(BitSet result, BitSet s1, BitSet s2);
store in the array result the set resulting from the intersection of s1 and s2
-x is an element of s1 intersection s2 if x is an element of s1 and x is an element of s2
void difference(BitSet result, BitSet s1, BitSet s2);
store in the array result the set resulting from the difference of s1 and s2
x is an element of s1 s2 if x is an element of s1 and x is not an element of s2
int is Empty(BitSet s);
the set is empty of all array elements are false
int contains(BitSet s,int elem);
elem is an element of s if the array value indexed by elem is TRUE
int disjoint(BitSet s1, BitSet s2);
- two sets are disjoint if the intersection is empty
int equal(BitSet s1, BitSet s2);
- two sets are equal if they have exactly the same elements
int cardinality (BitSet s);
- the cardinality of the set is the number of TRUE elements
int subset(BitSet s 1, BitSet s2);
- s1 is a subset of s2 if all elements of s1 are in s2](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa40901f2-139a-41b3-9e9b-ab3fcb7a750b%2Fae70f619-b0af-46d2-ad7a-950f868f2999%2F276opxb_processed.jpeg&w=3840&q=75)
Transcribed Image Text:A set of integers 0..MAX may be implemented using an array of boolean values. This
particular implementation is called a bit-vector implementation of a Set. Since C doesn't have
the Boolean data type, then substitute TRUE with 1 and FALSE with 0, or define 1 and 0 as
TRUE and FALSE, respectively.
For example, if the integer 3 is an element of the set, then the array element indexed by 3 is
TRUE. On the other hand, if 3 is not an element, then the array element indexed by 3 is
FALSE.
For example: if s= (3,4,6,8), the array looks like this:
0
1
2
3
4
5
6
7
8
9
FALSE FALSE FALSE TRUE TRUE FALSE TRUE FALSE TRUE FALSE
Implement a programmer-defined data type called BitSet to represent a set as follows:
typedef int BitSet[MAX];
Implement the following functions:
void initialize(BitSet s);
set all array elements to FALSE
void add(int elem, BitSet s);
set the item indexed by elem to TRUE
void display(BitSet s);
display the set on the screen using set notation, e.g. (3,4,5,6)
- this means that you will print the index value if the content of that cell is TRUE
void getUnion(BitSet result, BitSet s1, BitSet s2);
store in the array result the set resulting from the union of s1 and s2
x is an element of s1 union s2 if x is an element of s1 or x is an element of s2
void intersection(BitSet result, BitSet s1, BitSet s2);
store in the array result the set resulting from the intersection of s1 and s2
-x is an element of s1 intersection s2 if x is an element of s1 and x is an element of s2
void difference(BitSet result, BitSet s1, BitSet s2);
store in the array result the set resulting from the difference of s1 and s2
x is an element of s1 s2 if x is an element of s1 and x is not an element of s2
int is Empty(BitSet s);
the set is empty of all array elements are false
int contains(BitSet s,int elem);
elem is an element of s if the array value indexed by elem is TRUE
int disjoint(BitSet s1, BitSet s2);
- two sets are disjoint if the intersection is empty
int equal(BitSet s1, BitSet s2);
- two sets are equal if they have exactly the same elements
int cardinality (BitSet s);
- the cardinality of the set is the number of TRUE elements
int subset(BitSet s 1, BitSet s2);
- s1 is a subset of s2 if all elements of s1 are in s2
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 7 images

Recommended textbooks for you
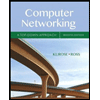
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
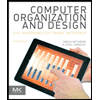
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
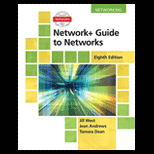
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
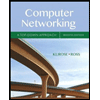
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
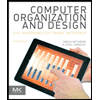
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
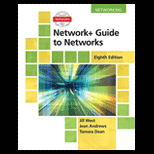
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
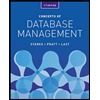
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
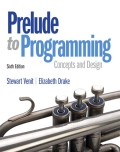
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
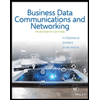
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY