a.)Write a C++ program that consists of a function declaration, a function definition and a main. The function declaration is as follows: void endTime (int ch, int cm, char cap, int dh, int dm, int eh, int em, char eap) // given any current hour, minutes and 'A' or 'P' for am/pm in parameters ch, cm and cap respectively, // and also given the duration (hours and minutes) of an activity in parameters dh and dm, // the function stores the end time in parameters eh, em and eap. Where eh will hold the hour of the end time, em holds the minute and eap holds eithe 'A' or 'P' for the end time. // All values are using a 12 hour clock // Function assumes all activities end in the same day Also include a main program that feeds the following arguments to the functions: 11, 25, 'A', 3, 45, hour, minute, ampm causing the function to calculate the end time as 3 10 P Using the calculated results, the main then outputs: If the current time is 11:25AM and we have 3 hours and 45 minutes to go, we have to sit here until 3:10PM :( The main then calls the function a second time with arguments curHour, curMin, curAP, durHour, durMin, finHour, finMinute, finAmPm, where the parameters are set to the following values before the call: curHour to 9 curMin to 15 curAP to 'A' durHour to 0 durMin to 45 finHour to 3 finMinute to 4 finAmPm to 'P' The main then using the results from the function call, outputs the follows: If the current time is 9:15AM and we have 0 hours and 45 minutes to go, we have to sit here until 10:00AM :) Outputting the smiling and frowning faces are optional, also which one and under what conditions to output are also up to you. b.)changed the start time passed to the function from 12:25 AM to 11:25 AM, which of course will also affect the calculated end time.
a.)Write a C++ program that consists of a function declaration, a function definition and a main. The function declaration is as follows:
void endTime (int ch, int cm, char cap, int dh, int dm, int eh, int em, char eap)
// given any current hour, minutes and 'A' or 'P' for am/pm in parameters ch, cm and cap respectively,
// and also given the duration (hours and minutes) of an activity in parameters dh and dm,
// the function stores the end time in parameters eh, em and eap. Where eh will hold the hour of the end time, em holds the minute and eap holds eithe 'A' or 'P' for the end time.
// All values are using a 12 hour clock
// Function assumes all activities end in the same day
Also include a main program that feeds the following arguments to the functions:
11, 25, 'A', 3, 45, hour, minute, ampm
causing the function to calculate the end time as 3 10 P
Using the calculated results, the main then outputs:
If the current time is 11:25AM and we have 3 hours and 45 minutes to go, we have to sit here until 3:10PM :(
The main then calls the function a second time with arguments curHour, curMin, curAP, durHour, durMin, finHour, finMinute, finAmPm, where the parameters are set to the following values before the call:
curHour to 9
curMin to 15
curAP to 'A'
durHour to 0
durMin to 45
finHour to 3
finMinute to 4
finAmPm to 'P'
The main then using the results from the function call, outputs the follows:
If the current time is 9:15AM and we have 0 hours and 45 minutes to go, we have to sit here until 10:00AM :)
Outputting the smiling and frowning faces are optional, also which one and under what conditions to output are also up to you.
b.)changed the start time passed to the function from 12:25 AM to 11:25 AM, which of course will also affect the calculated end time.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

I dont need to use : #include <bits/stdc++.h>
what is an alternative, I only know basic C++ not that
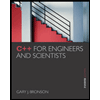
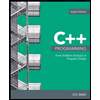
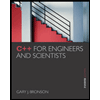
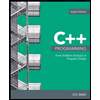