abstract
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 3PE
Related questions
Question
- Polygon Class (abstract superclass)
- Variables
- double width
- double height
- double depth
- Variables
-
- Member functions
- set_values()
- Sets values for height and width based upon user input
- get_values()
- Display the current width and height values
- set_values()
- Polymorphic member functions:
-
- area()
- perimeter()
- draw()
-
- Make Polygon Class Abstract
- Member functions
- Right_Triangle Class (subclass of Polygon)
- Polymorphic member functions:
- area()
- (height *width) /2
- perimeter()
- height + width + (sqrt(height*height + width*width))
- draw()
- area()
- Polymorphic member functions:
- Rectangle Class (subclass of Polygon)
- Variables
- int size = 3
- double temp_result_for_chaining
- double* history_width_ptr
- double* history_height_ptr
- Variables
-
-
- int array_index
- or
- double* temp_history_width_ptr
- double* temp_history_height_ptr
- int array_index
- Functions
- Constructor creates space in free memory for two arrays that can store previous values of width and height:
- history_width_ptr = new double[size] // allocate new memory to previous width values
- history_height_ptr = new double[size] // allocate new memory to previous height values
- Constructor creates space in free memory for two arrays that can store previous values of width and height:
-
-
- Destructor
- delete[] history_width_ptr
- delete[] history_height_ptr
- Destructor
-
- store_in_arrays()
- Stores width and height values into the two arrays in free storage pointed at by history_width_ptr and history_height_ptr
- get_historical_values()
- Display the historical width and height values from the two arrays.
- Polymorphic member functions
- area()
- perimeter()
- Add all the sides.
- draw()
- Example output:
- store_in_arrays()
-----------------
| |
-----------------
-
-
- You don’t have to draw to scale, but show show a symbolic example of a rectangle
-
- Learning from the "this Pointer and Methods/Functions Chaining" example code in the files above, add method/function chaining to class Rectangle.
- Add these methods/functions with the ability to chain:
- multiply_width_by_height()
- multiply_by_depth()
- display_volume()
- Using this-> and the return *this within your chaining functions.
- Add these methods/functions with the ability to chain:
- Within main()
- Two objects are created: a rectangle and a right-triangle.
- User input is aquired 3 times for modifying the two objects in terms of height, width, and depth (depth is used only by the rectangle).
- Demonstrate dynamic polymorphism by calling the polymorphic member functions: area(), circumference(), and draw()
- Also, dynamic memory is implemented through the use of the history arrays in free storage (the heap) used by Rectangle objects.
- Fill the two arrays with historical values of width and height and display their values.
- Demonstrate methods/functions chaining by calling up these functions through a Rectangle object in main.
- multiply_width_by_height()
- multiply_by_depth()
- display_volume()
-
- EXAMPLE OUTPUT:
- You will enter the width and height to create both a triangle object and a rectangle object...
- Please enter the width: 5
- Please enter the height: 4
- For Right-Triangle
- Area = 10
- Perimeter = 15.4
- / |
- / |
- ------
- For Rectangle
- Area = 20
- Perimeter = 18
- -------------
- | |
- -------------
- Please enter the depth: 10
- Volume of Rectangle using method or function chaining = 200
- EXAMPLE OUTPUT:
-
-
- THE ABOVE LOOPS FOR 3 TIMES (TO FILL THE ARRAYS) AND THEN OUTPUTS THE FINAL ARRAYS
- Height Array = 5, ...
- Width Array = 4, ...
- THE ABOVE LOOPS FOR 3 TIMES (TO FILL THE ARRAYS) AND THEN OUTPUTS THE FINAL ARRAYS
-
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
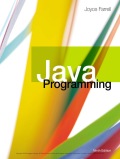
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
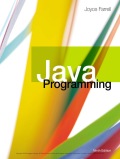
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage