Activity #2: Write a program that computes parking fees for a garage with the fee structure given in the figure below. The program should prompt a user to input from the keyboard the number of hours parked in the garage, and output the total fee for parking. A lost ticket is signified using a negative value for hours. Partial hours are charged as full hours, e.g. 5 hours and 20 minutes is charged as 6 hours. You must take as input the number of hours as a single floating-point number rather than as separate values of "hours" and "minutes" (example: 5.25 hours). Thus, the output should always be an integer. Examples (understand the PARKING FEES O): PARKING FEES PARKING RATES parked for 0 hours, pay $0 parked for 2 hours, pay $4 0-2 Hours parked for 3 hours, pay $4 + $3 = $7 $4.00 $3.00 $1.00 2-4 Hours parked for 5 hours, pay $4 + $3 + $1 = $8 Each Additional Hour parked for 20 hours, pay $23 Maximum Daily Charge $24.00 Lost Ticket Charge $36.00 parked for 22 hours, pay $24 PAY AT FRONT DESK PRIOR parked for 123 hours (5 days, 3 hours), pay 5*$24 + $4 + $3 = $127 TO DEPARTURE parked for 123 hours but lost the ticket (user inputted -123), pay $163 Document your work in file Lab4a_Act2.pdf for submission. You should not code until you develop and check your algorithm by hand, on paper. Then, translate your algorithm to Python as Lab4a_Act2.py:.Verify that your code delivers the correct output for the case data given above. Your program should perform the following general tasks: • Use descriptive prompts telling the user what to enter from the keyboard o Including units, if relevant • Store input in appropriately named variables • Convert the input to a number if you plan to perform mathematical operations with the input value • Perform necessary decisions/procedures/calculations and store results in appropriately named variables • Output results to the screen using the format below Example output (using input 123): Enter the hours parked as a decimal number. Include a negative sign if the ticket is lost. Please enter the hours parked: 123 Parking for 123.0 hours please pay $127
Activity #2: Write a program that computes parking fees for a garage with the fee structure given in the figure below. The program should prompt a user to input from the keyboard the number of hours parked in the garage, and output the total fee for parking. A lost ticket is signified using a negative value for hours. Partial hours are charged as full hours, e.g. 5 hours and 20 minutes is charged as 6 hours. You must take as input the number of hours as a single floating-point number rather than as separate values of "hours" and "minutes" (example: 5.25 hours). Thus, the output should always be an integer. Examples (understand the PARKING FEES O): PARKING FEES PARKING RATES parked for 0 hours, pay $0 parked for 2 hours, pay $4 0-2 Hours parked for 3 hours, pay $4 + $3 = $7 $4.00 $3.00 $1.00 2-4 Hours parked for 5 hours, pay $4 + $3 + $1 = $8 Each Additional Hour parked for 20 hours, pay $23 Maximum Daily Charge $24.00 Lost Ticket Charge $36.00 parked for 22 hours, pay $24 PAY AT FRONT DESK PRIOR parked for 123 hours (5 days, 3 hours), pay 5*$24 + $4 + $3 = $127 TO DEPARTURE parked for 123 hours but lost the ticket (user inputted -123), pay $163 Document your work in file Lab4a_Act2.pdf for submission. You should not code until you develop and check your algorithm by hand, on paper. Then, translate your algorithm to Python as Lab4a_Act2.py:.Verify that your code delivers the correct output for the case data given above. Your program should perform the following general tasks: • Use descriptive prompts telling the user what to enter from the keyboard o Including units, if relevant • Store input in appropriately named variables • Convert the input to a number if you plan to perform mathematical operations with the input value • Perform necessary decisions/procedures/calculations and store results in appropriately named variables • Output results to the screen using the format below Example output (using input 123): Enter the hours parked as a decimal number. Include a negative sign if the ticket is lost. Please enter the hours parked: 123 Parking for 123.0 hours please pay $127
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
4act2 Please help me solve this one question in python
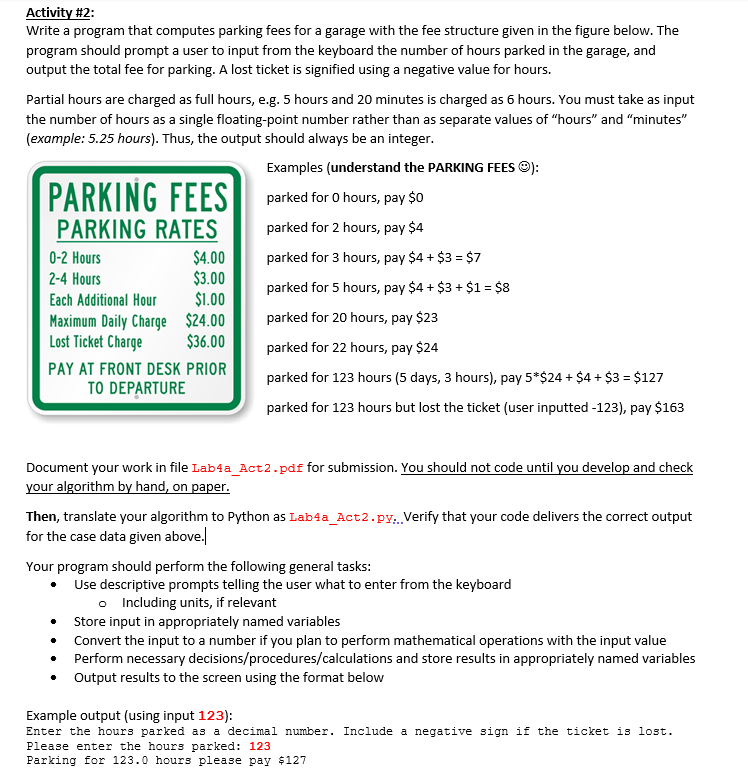
Transcribed Image Text:Activity #2:
Write a program that computes parking fees for a garage with the fee structure given in the figure below. The
program should prompt a user to input from the keyboard the number of hours parked in the garage, and
output the total fee for parking. A lost ticket is signified using a negative value for hours.
Partial hours are charged as full hours, e.g. 5 hours and 20 minutes is charged as 6 hours. You must take as input
the number of hours as a single floating-point number rather than as separate values of "hours" and "minutes"
(example: 5.25 hours). Thus, the output should always be an integer.
Examples (understand the PARKING FEES O):
PARKING FEES
PARKING RATES
parked for 0 hours, pay $0
parked for 2 hours, pay $4
$4.00
$3.00
$1.00
Maximum Daily Charge $24.00
$36.00
0-2 Hours
parked for 3 hours, pay $4 + $3 = $7
2-4 Hours
parked for 5 hours, pay $4 + $3 + $1 = $8
Each Additional Hour
parked for 20 hours, pay $23
Lost Ticket Charge
parked for 22 hours, pay $24
PAY AT FRONT DESK PRIOR
parked for 123 hours (5 days, 3 hours), pay 5*$24 + $4 + $3 = $127
TO DEPARTURE
parked for 123 hours but lost the ticket (user inputted -123), pay $163
Document your work in file Lab4a_Act2.pdf for submission. You should not code until you develop and check
your algorithm by hand, on paper.
Then, translate your algorithm to Python as Lab4a_Act2.py:. Verify that your code delivers the correct output
for the case data given above.
Your program should perform the following general tasks:
Use descriptive prompts telling the user what to enter from the keyboard
o Including units, if relevant
Store input in appropriately named variables
Convert the input to a number if you plan to perform mathematical operations with the input value
Perform necessary decisions/procedures/calculations and store results in appropriately named variables
Output results to the screen using the format below
Example output (using input 123):
Enter the hours parked as a decimal number. Include a negative sign if the ticket is lost.
Please enter the hours parked: 123
Parking for 123.0 hours please pay $127
Expert Solution

Step 1
Objective: This program reads the number of hours parked for vehicles and computes the total charge. The charges for car parking are given in the question. The total charge should be calculated according to those specifications.
Programming language: Python
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Recommended textbooks for you
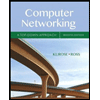
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
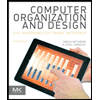
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
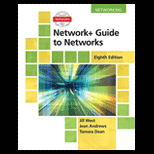
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
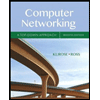
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
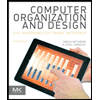
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
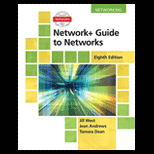
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
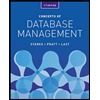
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
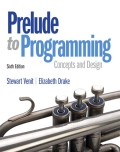
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
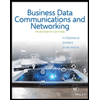
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY