Add three methods to the Student class that compare twoStudent objects. One method (__eq__) should test for equality. A second method (__lt__) should test for less than. The third method (__ge__) should test for greater than or equal to. In each case, the method returns the result of the comparison of the two students’ names. Include a main function that tests all of the comparison operators. Note: The program should output in the following format: False: False True: True True: True False: False True: True True: True True: True True: True True: True True: True code: class Student(object): """Represents a student.""" def __init__(self, name, number): """All scores are initially 0.""" self.name = name self.scores = [] for count in range(number): self.scores.append(0) def getName(self): """Returns the student's name.""" return self.name def setScore(self, i, score): """Resets the ith score, counting from 1.""" self.scores[i - 1] = score def getScore(self, i): """Returns the ith score, counting from 1.""" return self.scores[i - 1] def getAverage(self): """Returns the average score.""" return sum(self.scores) / len(self._scores) def getHighScore(self): """Returns the highest score.""" return max(self.scores) def __str__(self): """Returns the string representation of the student.""" return "Name: " + self.name + "\nScores: " + \ " ".join(map(str, self.scores)) # Write method definitions here def __eq__(self, other): """Returns true if student names are equal.""" return self.name==other.name def __lt__(self, other): """Returns for ab name of a is lexographical before b.""" return self.name>other.name def main(): """A simple test.""" student = Student("Ken", 5) print(student) for i in range(1, 6): student.setScore(i, 100) print(student) # create another student and test for equility student2 = Student("Ken", 6) print(student==student2) #this should print True # create another student and test for le and ge student3 = Student("John", 6) print(student2>student3) #this should print True print(student2
Add three methods to the Student class that compare twoStudent objects. One method (__eq__) should test for equality. A second method (__lt__) should test for less than. The third method (__ge__) should test for greater than or equal to. In each case, the method returns the result of the comparison of the two students’ names. Include a main function that tests all of the comparison operators. Note: The program should output in the following format: False: False True: True True: True False: False True: True True: True True: True True: True True: True True: True code: class Student(object): """Represents a student.""" def __init__(self, name, number): """All scores are initially 0.""" self.name = name self.scores = [] for count in range(number): self.scores.append(0) def getName(self): """Returns the student's name.""" return self.name def setScore(self, i, score): """Resets the ith score, counting from 1.""" self.scores[i - 1] = score def getScore(self, i): """Returns the ith score, counting from 1.""" return self.scores[i - 1] def getAverage(self): """Returns the average score.""" return sum(self.scores) / len(self._scores) def getHighScore(self): """Returns the highest score.""" return max(self.scores) def __str__(self): """Returns the string representation of the student.""" return "Name: " + self.name + "\nScores: " + \ " ".join(map(str, self.scores)) # Write method definitions here def __eq__(self, other): """Returns true if student names are equal.""" return self.name==other.name def __lt__(self, other): """Returns for ab name of a is lexographical before b.""" return self.name>other.name def main(): """A simple test.""" student = Student("Ken", 5) print(student) for i in range(1, 6): student.setScore(i, 100) print(student) # create another student and test for equility student2 = Student("Ken", 6) print(student==student2) #this should print True # create another student and test for le and ge student3 = Student("John", 6) print(student2>student3) #this should print True print(student2
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 2CP
Related questions
Question
Add three methods to the Student class that compare twoStudent objects. One method (__eq__) should test for equality. A second method (__lt__) should test for less than. The third method (__ge__) should test for greater than or equal to. In each case, the method returns the result of the comparison of the two students’ names. Include a main function that tests all of the comparison operators.
Note: The program should output in the following format:
False: False
True: True
True: True
False: False
True: True
True: True
True: True
True: True
True: True
True: True
code:
class Student(object):
"""Represents a student."""
def __init__(self, name, number):
"""All scores are initially 0."""
self.name = name
self.scores = []
for count in range(number):
self.scores.append(0)
def getName(self):
"""Returns the student's name."""
return self.name
def setScore(self, i, score):
"""Resets the ith score, counting from 1."""
self.scores[i - 1] = score
def getScore(self, i):
"""Returns the ith score, counting from 1."""
return self.scores[i - 1]
def getAverage(self):
"""Returns the average score."""
return sum(self.scores) / len(self._scores)
def getHighScore(self):
"""Returns the highest score."""
return max(self.scores)
def __str__(self):
"""Returns the string representation of the student."""
return "Name: " + self.name + "\nScores: " + \
" ".join(map(str, self.scores))
# Write method definitions here
def __eq__(self, other):
"""Returns true if student names are equal."""
return self.name==other.name
def __lt__(self, other):
"""Returns for a<b name of a is lexographical before b."""
return self.name<other.name
def __ge__(self, other):
"""Returns for a>b name of a is lexographical before b."""
return self.name>other.name
def main():
"""A simple test."""
student = Student("Ken", 5)
print(student)
for i in range(1, 6):
student.setScore(i, 100)
print(student)
# create another student and test for equility
student2 = Student("Ken", 6)
print(student==student2) #this should print True
# create another student and test for le and ge
student3 = Student("John", 6)
print(student2>student3) #this should print True
print(student2<student3) #this should print False
if __name__ == "__main__":
main()
Add method to test for greater than or equal toExpert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
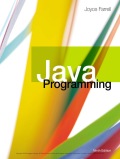
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
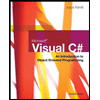
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
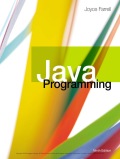
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
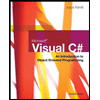
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,