Analyze the time complexity of this program in big-O notation, assuming that the total number of numbers and symbols in the expression is n. Expect any type of numbers including integers, floats/doubles static double evalPostFix() { Stack s = new Stack(); String token; Double a, b, result=0.0; boolean isNumber; Scanner sc = new Scanner(System.in); token = sc.next(); while (token.charAt(0) != '=') { try { isNumber = true; result = Double.parseDouble(token); } catch (Exception e) { isNumber = false; } if (isNumber) s.push(result); else { switch (token.charAt(0)) { case ’+’: a = s.pop(); b = s.pop(); s.push(a+b); break; case ’-’: a = s.pop(); b = s.pop(); s.push(a-b); break; case ’*’: a = s.pop(); b = s.pop(); s.push(a*b); break; case ’/’: a = s.pop(); b = s.pop(); s.push(a/b); break; case ’^’: a = s.pop(); b = s.pop(); s.push(Math.exp(a*Math.log(b))); break; } } token = sc.next(); } return s.peek(); }
Analyze the time complexity of this
static double evalPostFix()
{
Stack<Double> s = new Stack<Double>();
String token;
Double a, b, result=0.0;
boolean isNumber;
Scanner sc = new Scanner(System.in);
token = sc.next();
while (token.charAt(0) != '=')
{
try
{
isNumber = true;
result = Double.parseDouble(token);
}
catch (Exception e)
{
isNumber = false;
}
if (isNumber)
s.push(result);
else
{
switch (token.charAt(0))
{
case ’+’: a = s.pop(); b = s.pop();
s.push(a+b); break;
case ’-’: a = s.pop(); b = s.pop();
s.push(a-b); break;
case ’*’: a = s.pop(); b = s.pop();
s.push(a*b); break;
case ’/’: a = s.pop(); b = s.pop();
s.push(a/b); break;
case ’^’: a = s.pop(); b = s.pop();
s.push(Math.exp(a*Math.log(b)));
break;
}
}
token = sc.next();
}
return s.peek();
}

Step by step
Solved in 3 steps

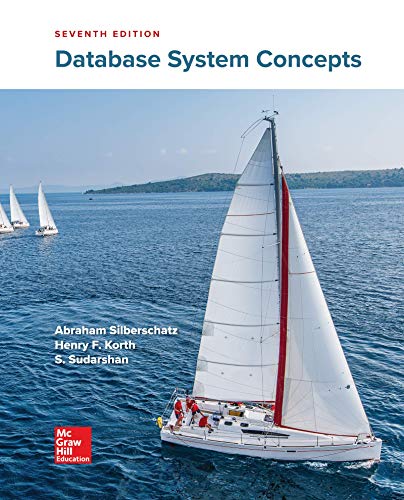
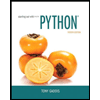
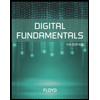
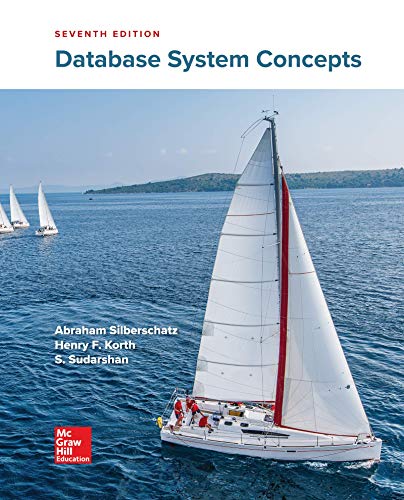
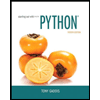
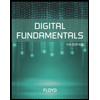
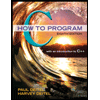
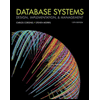
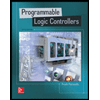