Answer in Swift language please: Urgent Class design and implementation. create a command line in which you should design and implement a math operation class for fraction calculation. The class name is MOpt, which should include numerator and denominator and support the functions: add, subtract, multiply, and divide. You should also implement the operation reduce, where all the operations performed (+, -, *, /) must be reduced to the simplest form before being returned. Your class should perform the following math operations: 1/4 + 3/4 = 4/4 = 1 1/4 – 3/4 = -1/2 (not -2/4 using operation reduce) 3/4 – 1/4 = 1/2 (not 2/4) 1/2 * 2/5 = 1/5 (not 2/10) 1/4 / 1/2 = 1/2 (not 2/4) Your code should look like this: let m1 = MOpt(1, 4) let m2 = MOpt(3, 4) let m3 = MOpt(1, 2) let m4 = MOpt(2, 5) let m5 = m1.add(m2) let m6 = m1.subtract(m2) let m7 = m2.subtract(m1) let m8 = m3.multiply(m4) let m9 = m1.divide(m3) The toString() method should be create to print out the fraction number, for example, m1.toString() should output 1/4 string.
Answer in Swift language please: Urgent
Class design and implementation. create a command line in which you should design and implement a math operation class for fraction calculation. The class name is MOpt, which should include numerator and denominator and support the functions: add, subtract, multiply, and divide.
You should also implement the operation reduce, where all the operations performed (+, -, *, /) must be reduced to the simplest form before being returned.
Your class should perform the following math operations: 1/4 + 3/4 = 4/4 = 1
1/4 – 3/4 = -1/2 (not -2/4 using operation reduce)
3/4 – 1/4 = 1/2 (not 2/4)
1/2 * 2/5 = 1/5 (not 2/10)
1/4 / 1/2 = 1/2 (not 2/4)
Your code should look like this:
let m1 = MOpt(1, 4)
let m2 = MOpt(3, 4)
let m3 = MOpt(1, 2)
let m4 = MOpt(2, 5)
let m5 = m1.add(m2)
let m6 = m1.subtract(m2)
let m7 = m2.subtract(m1)
let m8 = m3.multiply(m4)
let m9 = m1.divide(m3)
The toString() method should be create to print out the fraction number, for example, m1.toString() should output 1/4 string.

Step by step
Solved in 2 steps

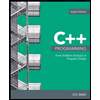
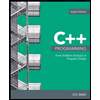