Appendix 1 (bisection algorithm) index 50 To find the square root of a number N the bisection method works as follows: | create 2 variables Left and Right, where Left = 0 and Right = number Find the midpoint between Left and Right If Midpoint Midpoint < number, then modify the value of the Left to be the value of midpoint • Otherwise, modify the value of the Right to be the value of the Midpoint. Step 1 Step 2 Repeat step 2 as much as needed! |For this TMA, you need to repeat step 2 only 5 times. Graphical Explanation of the bisection method for finding the square root of 50: Let L represent Left, R represent Right, and M represent Midpoint M25 R50 25* 25 > 50 M-12.5 R-25 12.5 125 > 50 6.25 * 6.25 < 50 M6.25 R12.5 9.375 * 9.375 > 50 Lu6.25 Re125 Now Left should be 6.25, Right should be 9.375 and the algorithm continues. To find the cube root of a number N the bisection method works as follows: Step 1 if the radicand is negative, then create a new radicand that is the opposite of the original number, which is positive! create 2 variables Left and Right, where Left = 0 and Right = number • Find the midpoint between Left and Right • f Midpoint * Midpoint Midpoint < number, then modify the value of the Left to be the value of midpoint Step 2 Step 3 Otherwise, modify the value of the Right to be the value of the Midpoint. Repeat step 2 as much as needed! For this TMA, you need to repeat step 2 only 5 times. If the radicand is negative, the radical should be the negative value of the number found, otherwise the radical should be the number found. Step 4
Appendix 1 (bisection algorithm) index 50 To find the square root of a number N the bisection method works as follows: | create 2 variables Left and Right, where Left = 0 and Right = number Find the midpoint between Left and Right If Midpoint Midpoint < number, then modify the value of the Left to be the value of midpoint • Otherwise, modify the value of the Right to be the value of the Midpoint. Step 1 Step 2 Repeat step 2 as much as needed! |For this TMA, you need to repeat step 2 only 5 times. Graphical Explanation of the bisection method for finding the square root of 50: Let L represent Left, R represent Right, and M represent Midpoint M25 R50 25* 25 > 50 M-12.5 R-25 12.5 125 > 50 6.25 * 6.25 < 50 M6.25 R12.5 9.375 * 9.375 > 50 Lu6.25 Re125 Now Left should be 6.25, Right should be 9.375 and the algorithm continues. To find the cube root of a number N the bisection method works as follows: Step 1 if the radicand is negative, then create a new radicand that is the opposite of the original number, which is positive! create 2 variables Left and Right, where Left = 0 and Right = number • Find the midpoint between Left and Right • f Midpoint * Midpoint Midpoint < number, then modify the value of the Left to be the value of midpoint Step 2 Step 3 Otherwise, modify the value of the Right to be the value of the Midpoint. Repeat step 2 as much as needed! For this TMA, you need to repeat step 2 only 5 times. If the radicand is negative, the radical should be the negative value of the number found, otherwise the radical should be the number found. Step 4
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Solve them by using Appendix 1
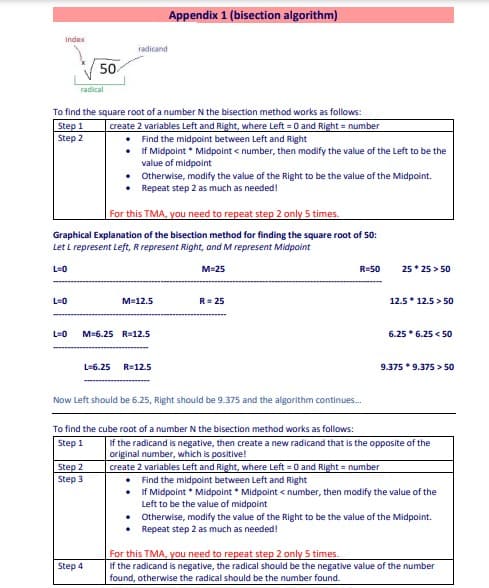
Transcribed Image Text:Appendix 1 (bisection algorithm)
Index
radicand
50
radical
To find the square root of a number N the bisection method works as follows:
create 2 variables Left and Right, where Left = 0 and Right = number
Step 1
Step 2
Find the midpoint between Left and Right
If Midpoint * Midpoint < number, then modify the value of the Left to be the
value of midpoint
Otherwise, modify the value of the Right to be the value of the Midpoint.
Repeat step 2 as much as needed!
For this TMA, you need to repeat step 2 only 5 times.
Graphical Explanation of the bisection method for finding the square root of 50:
Let L represent Left, R represent Right, and M represent Midpoint
L=0
M=25
R=50
25* 25 > 50
L=0
M=12.5
R= 25
12.5* 12.5 > 50
L=0
M=6.25 R=12.5
6.25 * 6.25 < 50
L=6.25
R=12.5
9.375 * 9.375 > 50
Now Left should be 6.25, Right should be 9.375 and the algorithm continues.
To find the cube root of a number N the bisection method works as follows:
Step 1
If the radicand is negative, then create a new radicand that is the opposite of the
original number, which is positive!
create 2 variables Left and Right, where Left = 0 and Right = number
Step 2
Step 3
Find the midpoint between Left and Right
If Midpoint * Midpoint * Midpoint < number, then modify the value of the
Left to be the value of midpoint
• Otherwise, modify the value of the Right to be the value of the Midpoint.
Repeat step 2 as much as needed!
For this TMA, you need to repeat step 2 only 5 times.
If the radicand is negative, the radical should be the negative value of the number
found, otherwise the radical should be the number found.
Step 4
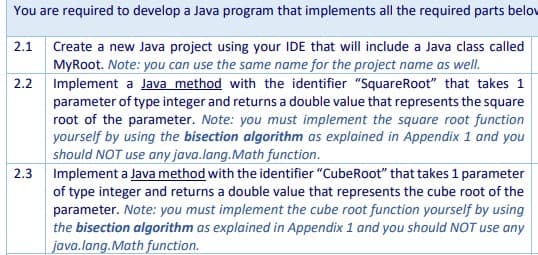
Transcribed Image Text:You are required to develop a Java program that implements all the required parts belov
2.1 Create a new Java project using your IDE that will include a Java class called
MyRoot. Note: you can use the same name for the project name as well.
2.2 Implement a Java method with the identifier "SquareRoot" that takes 1
parameter of type integer and returns a double value that represents the square
root of the parameter. Note: you must implement the square root function
yourself by using the bisection algorithm as explained in Appendix 1 and you
should NOT use any java.lang.Math function.
2.3 Implement a Java method with the identifier "CubeRoot" that takes 1 parameter
of type integer and returns a double value that represents the cube root of the
parameter. Note: you must implement the cube root function yourself by using
the bisection algorithm as explained in Appendix 1 and you should NOT use any
java.lang.Math function.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

Recommended textbooks for you
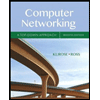
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
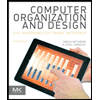
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
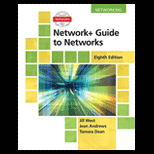
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
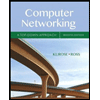
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
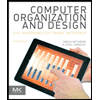
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
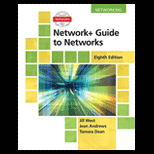
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
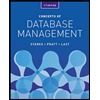
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
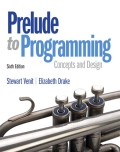
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
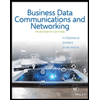
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY