Arman has a shop where he provides stuff on rent. The whole payment system is computerised. A customer has to enter the item name, item type, cost, and the number of days they have taken the item for rent. The system then tells them the cost per day. But there is a problem with the machine that if the number of days entered is not an integer value then the system would crash. To handle this, Arman decided to upgrade the software and use exception handling in case the customer entered any invalid input it should display "Number of days x is invalid". Write a C++ program to find the item cost per day with the cost for n days given. The arithmetic exception has to be thrown if the n is less than or equal to zero. Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. The class Item has the following private data members. Data type string string double Variable Name name type cost In the main method, get the necessary inputs and number of days from the user and display the item details, if the number of days is not less than or equal to zero. Input and output format: Display the Item Details with cost per day with respect to two decimal places. Refer Sample input and output for formatting specifications [All text in bold corresponds to input and rest corresponds to output] Sample Input and Output 1: Enter item name: table Enter item type: furniture Enter item cost: 30000 Enter number of days: 30 Item name:table Item type:furniture Item cost day:1000.00 Sample Input and Output 2: Enter item name: pen Enter item type: stationary Enter item cost: 2762 Enter number of days: Arithmetic Exception:Number of days 0 is invalid
QUESTION PROVIDED IN ATTACH IMAGE KINDLY SEE.
AND MATCH OUTPUT WITH QUESTION OUTPUT AS IT IS.
TEMPLATE PROVIDED BELOW USES IT WHILE MAKING SOLUTIONS.
------------------------------------------------------------------------------
MAIN.CPP
#include <iostream> // Fill the code to handle the Arithmetic Exception return 0;
|
Item.cpp
#include <iostream> using namespace std; class Item |
![Arman has a shop where he provides stuff on rent. The whole payment system is computerised. A
customer has to enter the item name, item type, cost, and the number of days they have taken the item
for rent. The system then tells them the cost per day. But there is a problem with the machine that if
the number of days entered is not an integer value then the system would crash. To handle this, Arman
decided to upgrade the software and use exception handling in case the customer entered any invalid
input it should display " Number of days x is invalid".
Write a C++ program to find the item cost per day with the cost for n days given. The arithmetic
exception has to be thrown if the n is less than or equal to zero.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class
names, member variable names, and function names should be the same as specified in the problem
statement.
The class Item has the following private data members.
Data type
string
string
double
Variable Name
name
type
cost
In the main method, get the necessary inputs and number of days from the user and display the item
details, if the number of days is not less than or equal to zero.
Input and output format:
Display the Item Details with cost per day with respect to two decimal places.
Refer Sample input and output for formatting specifications
[All text in bold corresponds to input and rest corresponds to output]
Sample Input and Output 1:
Enter item name:
table
Enter item type:
furniture
Enter item cost:
30000
Enter number of days:
30
Item name:table
Item type:furniture
Item cost per day:1000.00
Sample Input and Output 2:
Enter item name:
pen
Enter item type:
stationary
Enter item cost:
2762
Enter number of days:
Arithmetic Exception:Number of days 0 is invalid](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8deb4dee-94e2-4016-aac5-3aeff4bdb06f%2F1a65e79f-81c7-4bbb-aa7f-897bb86495a2%2F9uc9jep_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 2 images

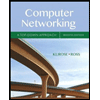
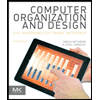
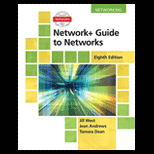
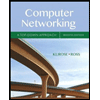
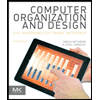
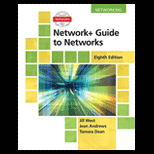
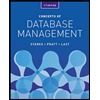
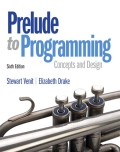
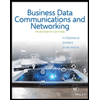