As described in the reading and lecture, an adjacency matrix for a graph with n vertices number 0, 1, …, n – 1 is an n by n array matrix such that matrix[i][j] is 1 (or true) if there is an edge from vertex i to vertex j, and 0 (or false) otherwise. 2. In this assignment, you will implement the methods identified in the stub code below in support of the Graph ADT that uses an adjacency matrix to represent an undirected, unweighted graph with no self-loops. import java.util.*; // for all needed JCF classes public class Graph { private int[][] matrix;// the adjacency matrix of the graph. // Creates an n x n arra
P R O B L E M D E S C R I P T I O N :
1. As described in the reading and lecture, an adjacency matrix for a graph with n vertices
number 0, 1, …, n – 1 is an n by n array matrix such that matrix[i][j] is 1 (or true) if
there is an edge from vertex i to vertex j, and 0 (or false) otherwise.
2. In this assignment, you will implement the methods identified in the stub code below in
support of the Graph ADT that uses an adjacency matrix to represent an undirected,
unweighted graph with no self-loops.
import java.util.*; // for all needed JCF classes
public class Graph {
private int[][] matrix;// the adjacency matrix of the graph.
// Creates an n x n array with all values initialized to 0.
public Graph(int n) {
// your code here
} // end constructor
// This method returns the number of nodes in the graph.
public int getNumVertices() {
// your code here
} // end getNumVertices
// This method returns the number of edges in the graph.
public int getNumEdges() {
// your code here
} // end getNumEdges
// This method returns true if node v and node w are
// neighbors; false otherwise. If either node does not
// exist in the graph, then an exception is thrown.
public boolean isEdge(int v, int w) throws GraphException {
// your code here
} // end getEdgeWeight
// This method adds an edge between node v and node w (changes
// the appropriate value in the adjacency matrix to 1). If
// either node does not exist in the graph, then an exception
// is thrown.
public void addEdge(int v, int w) throws GraphException {
// your code here
} // addEdge
// This method removes an edge between node v and node w (changes
// the appropriate value in the adjacency matrix to 0), should it
// exist. If either node does not exist in the graph, then an
// exception is thrown.
public void removeEdge(int v, int w) throws GraphException {
// your code here
} // end removeEdge
// This method returns the length of the shortest path between Node
// v and Node w. As an unweighted graph, each edge is considered to
// be length 1. It is recommended that a variation of a BFS is used
// starting at Node v. A HashMap can be used to store the distance
// from Node v to the current node.
public int shortestPathLength(int v, int w) {
// your code here
} // end shortestPathLength
// This method returns a string representation of the adjacency matrix.
// It is provided for testing purposes but does not display well for
// large graphs.
@Override
public String toString() {
String s = "";
for (int i = 0; i < matrix.length; i++) {
for (int j = 0; j < matrix[i].length; j++) {
s += matrix[i][j];
s += " ";
}
s += "\n";
}
return s;
} // end toString
} // end Graph
3. The methods that you are required to implement should, for the most part, be selfexplanatory from examining the provided code above. The code comments also provide
additional guidance.
a. You should not need to add any additional fields or methods to your
implementation.
b. No recursion is necessary for this assignment.
c. You will need to implement (but not submit the GraphException class).
d. Your implementation should not rely on you submitting any other class files.
e. Hints: For the graph traversal in the shortestPathLength method, you will
need to use classes from the Java Collections Framework instead of implementing
any additionally needed ADTs.
f. Start early and see me during Office Hours with any problems.
4. Your program should work in either a Windows environment or a Linux environment.
5. You should submit your work on the Linux server. Upload a backup copy to D2L.
6. Your program should work in either a Windows environment or a Linux environment. You
should submit your work on the Linux server. This is done from the command prompt:

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

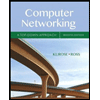
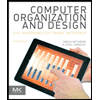
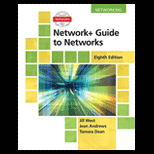
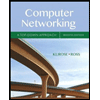
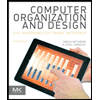
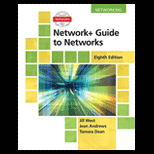
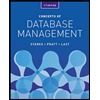
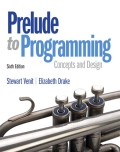
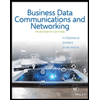