Assume p1, p2, and p3 are pointers to integer numbers. As an exampl consider int n1 = 33; int n2 = 11; int n3 = 22; You are asked to implement the function
Assume p1, p2, and p3 are pointers to integer numbers. As an exampl consider int n1 = 33; int n2 = 11; int n3 = 22; You are asked to implement the function
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter10: Pointers
Section: Chapter Questions
Problem 3PP
Related questions
Question
100%
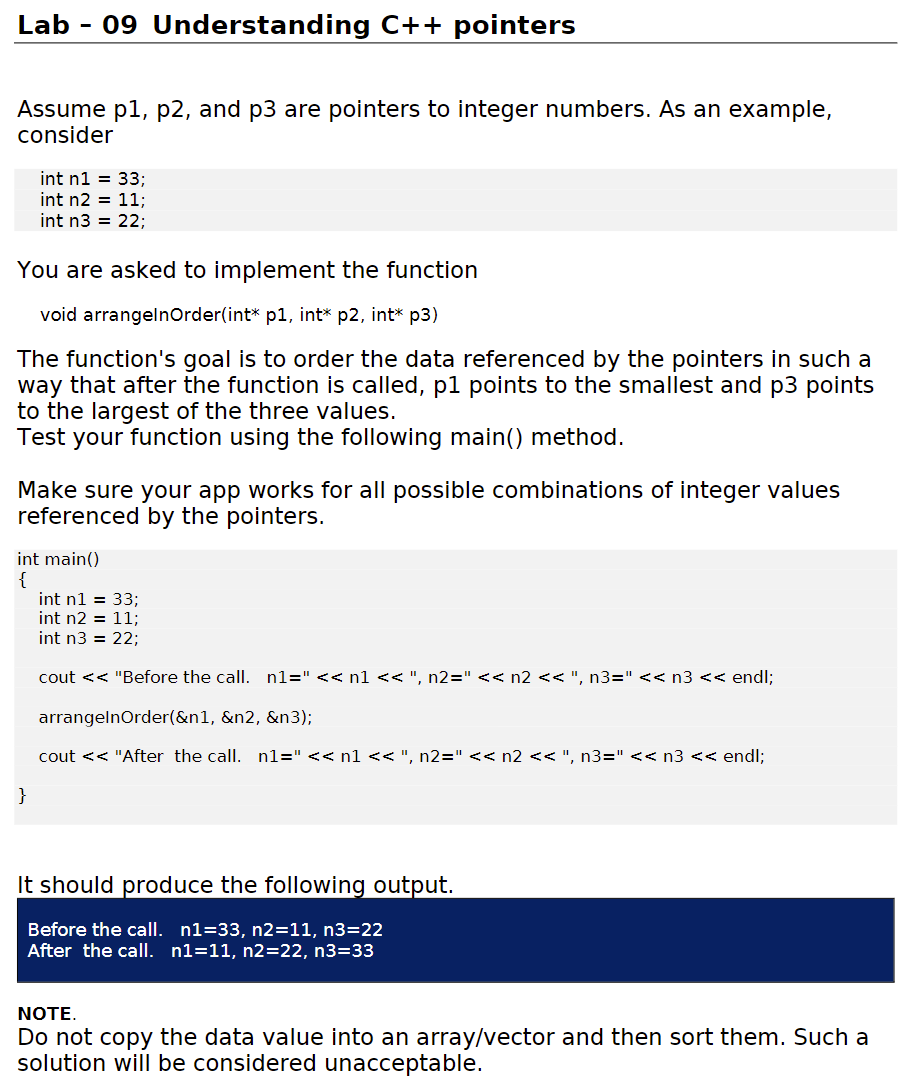
Transcribed Image Text:Lab
09 Understanding C++ pointers
Assume p1, p2, and p3 are pointers to integer numbers. As an example,
consider
int n1 = 33;
int n2 = 11;
int n3 = 22;
You are asked to implement the function
void arrangelnOrder(int* p1, int* p2, int* p3)
The function's goal is to order the data referenced by the pointers in such a
way that after the function is called, p1 points to the smallest and p3 points
to the largest of the three values.
Test your function using the following main() method.
Make sure your app works for all possible combinations of integer values
referenced by the pointers.
int main()
{
int n1 = 33;
int n2 = 11;
int n3 = 22;
cout << "Before the call. n1=" << n1<< ", n2="<< n2 << ", n3=" << n3 << endl;
arrangelnOrder(&n1, &n2, &n3);
cout << "After the call. n1=" << n1 << ", n2=" << n2 << ", n3=" << n3 << endl;
}
It should produce the following output.
Before the call. n1=33, n2=11, n3=22
After the call. n1=11, n2=22, n3=33
NOTE.
Do not copy the data value into an array/vector and then sort them. Such a
solution will be considered unacceptable.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
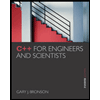
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
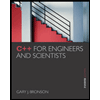
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr