at many prime numbers. Iterator() returns an Iterator object that will iterate through the primes, and toString() returns a string representation.
Consider the following class PrimeNumbers, which has three methods. The first, computePrimes() takes one integer input and calculates that many prime numbers. Iterator() returns an Iterator object that will iterate through the primes, and toString() returns a string representation.
public class PrimeNumbers implements Iterable<Integer>
{
private List<Integer> primes = new ArrayList<Integer>();
public void computePrimes (int n)
{
int count = 1; // count of primes
int number = 2; // number tested for primeness
boolean isPrime; // is this number a prime
while (count <= n)
{
isPrime = true;
for (int divisor = 2; divisor <= number / 2; divisor++)
{
if (number % divisor == 0)
{
isPrime = false;
break; // for loop
}
}
if (isPrime && (number % 10 != 9)) // FAULT
{
primes.add (number);
count++;
}
number++;
}
}
@Override public Iterator<Integer> iterator()
{
return primes.iterator();
}
2
@Override public String toString()
{
return primes.toString();
}
}
computePrimes() has a fault that causes it not to include prime numbers whose last digit is 9 (for example, it omits 19, 29, 59, 79, 89, 109, ...).
Normally, this problem is solved with the Sieve of Eratosthenes. The change in
(a) Reimplement the algorithm for calculating primes using the Sieve of Eratosthenes approach, but leave the fault in place.
(b) What is the first false positive and how many primes must a test case generate before encountering it?
(c) A test must reach a location in a

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

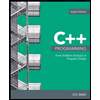
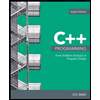