B. Project description For this project, you will create two ADTs: • A generic ADT Binary Search Tree • An ADT Class database: This ADT contains a list of class sections. Therefore, a class representing a class section must be created.
B. Project description For this project, you will create two ADTs: • A generic ADT Binary Search Tree • An ADT Class database: This ADT contains a list of class sections. Therefore, a class representing a class section must be created.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
B. Project description
For this project, you will create two ADTs:
• A generic ADT Binary Search Tree
• An ADT Class
must be created.
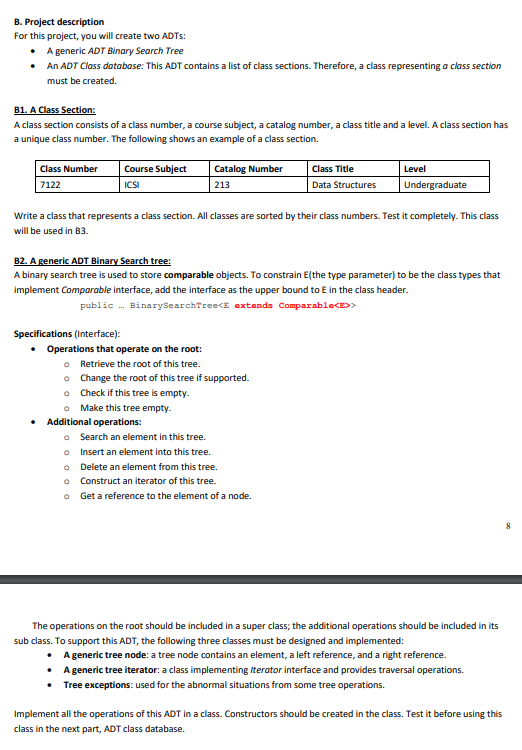
Transcribed Image Text:B. Project description
For this project, you will create two ADTS:
• A generic ADT Binary Search Tree
• An ADT Class database: This ADT contains a list of class sections. Therefore, a class representing a class section
must be created.
B1. A Class Section:
A class section consists of a class number, a course subject, a catalog number, a class title and a level. A class section has
a unique class number. The following shows an example of a class section.
Class Number
| Course Subject
Catalog Number
Class Title
Level
7122
ICSI
213
Data Structures
Undergraduate
Write a class that represents a class section. All classes are sorted by their class numbers. Test it completely. This class
will be used in B3.
B2. A generic ADT Binary Search tree:
A binary search tree is used to store comparable objects. To constrain E(the type parameter) to be the class types that
implement Comparable interface, add the interface as the upper bound to E in the class header.
public . BinarySearchTreecE extends Comparable<E>>
Specifications (Interface):
Operations that operate on the root:
o Retrieve the root of this tree.
o Change the root of this tree if supported.
o Check if this tree is empty.
O Make this tree empty.
Additional operations:
o Search an element in this tree.
o Insert an element into this tree.
o Delete an element from this tree.
o Construct an iterator of this tree.
o Get a reference to the element of a node.
The operations on the root should be included in a super class; the additional operations should be included in its
sub class. To support this ADT, the following three classes must be designed and implemented:
• A generic tree node: a tree node contains an element, a left reference, and a right reference.
• A generic tree iterator: a class implementing Iterator interface and provides traversal operations.
• Tree exceptions: used for the abnormal situations from some tree operations.
Implement all the operations of this ADT in a class. Constructors should be created in the class. Test it before using this
class in the next part, ADT class database.
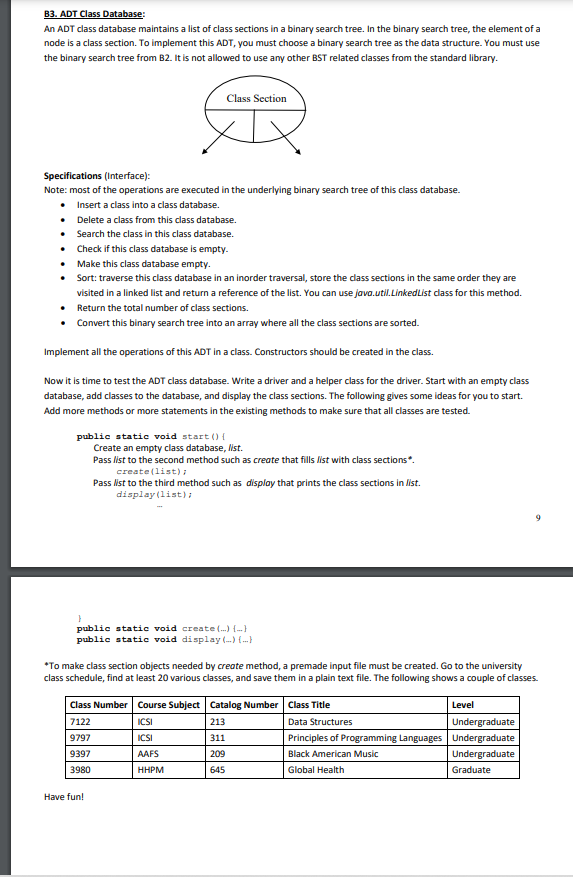
Transcribed Image Text:B3. ADT Class Database:
An ADT class database maintains a list of class sections in a binary search tree. In the binary search tree, the element of a
node is a class section. To implement this ADT, you must choose a binary search tree as the data structure. You must use
the binary search tree from B2. It is not allowed to use any other BST related classes from the standard library.
Class Section
Specifications (Interface):
Note: most of the operations are executed in the underlying binary search tree of this class database.
• Insert a class into a class database.
• Delete a class from this class database.
• Search the class in this class database.
• Check if this class database is empty.
• Make this class database empty.
• Sort: traverse this class database in an inorder traversal, store the dass sections in the same order they are
visited in a linked list and return a reference of the list. You can use java.util.LinkedList dass for this method.
• Return the total number of class sections.
• Convert this binary search tree into an array where all the class sections are sorted.
Implement all the operations of this ADT in a class. Constructors should be created in the class.
Now it is time to test the ADT class database. Write a driver and a helper class for the driver. Start with an empty class
database, add classes to the database, and display the class sections. The following gives some ideas for you to start.
Add more methods or more statements in the existing methods to make sure that all classes are tested.
public static void start () {
Create an empty class database, list.
Pass list to the second method such as create that fills list with class sections".
create (list);
Pass Nst to the third method such as display that prints the class sections in list.
display(list):
public static void create (.) {--}
public static void display (-) (--)
*To make class section objects needed by create method, a premade input file must be created. Go to the university
class schedule, find at least 20 various classes, and save them in a plain text file. The following shows a couple of classes.
Class Number Course Subject Catalog Number Class Title
Level
7122
ICSI
213
Data Structures
Undergraduate
9797
ICSI
311
Principles of Programming Languages Undergraduate
9397
AAFS
209
Black American Music
Undergraduate
3980
ННРМ
645
Global Health
Graduate
Have fun!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
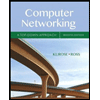
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
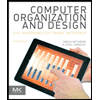
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
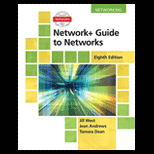
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
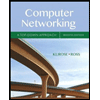
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
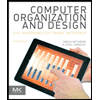
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
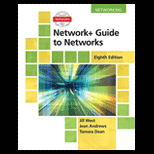
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
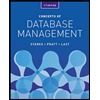
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
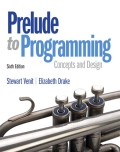
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
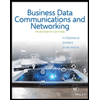
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY