Below is the code I typed and I attach two screenshots one with the prompt and the other is the feedback. If possible can someone fix my code base of the feedback or improve the entire code by using mainly loops in Python. Thank you. # Initialize the client list and balance NewClient = [] Balance = [] while True: print("1- Add a new client") print("2- Remove a client") print("3- Insert an item to the list") print("4- Search for the client based on firstName") print("5- Search for the client based on lastName") print("6- Sort the list in descending order (firstName)") print("7- Quit the program") option = input("Please enter your option: ") if option == "1": client_name = input("Please enter the client's name: ") balance = float(input("Please enter the client's balance: ")) NewClient.append(client_name) Balance.append(balance) elif option == "2": client_name = input("Please enter the client's name to remove: ") if client_name in NewClient: index = NewClient.index(client_name) NewClient.pop(index) Balance.pop(index) else: print("Client not found in the list.") elif option == "3": index = int(input("Where do you want to insert the client? (Enter the index number) ")) client_info = input("Please enter the client info: ") NewClient.insert(index, client_info) elif option == "4": first_name = input("Please enter the first name to search for: ") for i in range(len(NewClient)): if first_name in NewClient[i]: print("Client found:", NewClient[i]) print("Balance:", Balance[i]) break else: print("Client not found.") elif option == "6": NewClient.sort(reverse=True) elif option == "7": break else: print("Invalid option. Please choose a valid option.") # Display the updated list print("Your original List:") print("Client=",NewClient) print("Balance=", Balance) # After the loop ends print("Goodbye!")
Below is the code I typed and I attach two screenshots one with the prompt and the other is the feedback. If possible can someone fix my code base of the feedback or improve the entire code by using mainly loops in Python. Thank you.
# Initialize the client list and balance
NewClient = []
Balance = []
while True:
print("1- Add a new client")
print("2- Remove a client")
print("3- Insert an item to the list")
print("4- Search for the client based on firstName")
print("5- Search for the client based on lastName")
print("6- Sort the list in descending order (firstName)")
print("7- Quit the program")
option = input("Please enter your option: ")
if option == "1":
client_name = input("Please enter the client's name: ")
balance = float(input("Please enter the client's balance: "))
NewClient.append(client_name)
Balance.append(balance)
elif option == "2":
client_name = input("Please enter the client's name to remove: ")
if client_name in NewClient:
index = NewClient.index(client_name)
NewClient.pop(index)
Balance.pop(index)
else:
print("Client not found in the list.")
elif option == "3":
index = int(input("Where do you want to insert the client? (Enter the index number) "))
client_info = input("Please enter the client info: ")
NewClient.insert(index, client_info)
elif option == "4":
first_name = input("Please enter the first name to search for: ")
for i in range(len(NewClient)):
if first_name in NewClient[i]:
print("Client found:", NewClient[i])
print("Balance:", Balance[i])
break
else:
print("Client not found.")
elif option == "6":
NewClient.sort(reverse=True)
elif option == "7":
break
else:
print("Invalid option. Please choose a valid option.")
# Display the updated list
print("Your original List:")
print("Client=",NewClient)
print("Balance=", Balance)
# After the loop ends
print("Goodbye!")
![Create a menu to allow the banker to add a client, insert the client into a special location in the list, closing the account(remove), find the client based on firstName, and sort the list in
descending order (larger to smaller value).
NewClient=["Mike navarro","Miguel saba","Maria Rami"] Balance= [900.00, 400.00, 450.00]
You may use any of build in functions. Here is an sample output:
1- Add a new client
2- Remove a client
3- Insert an item to the list
4- Search for the client based on firstName
5-Search for the client based on lastName
6- Sort the list in descending order(firstName)
7- Quit the program
Please enter your option: 3
Where do you want to insert the client? (Enter the index number) 1
Please enter the client info: "Tina Mari"
Your original List: ["Mike navarro","Miguel saba","Maria Rami"]
After inserting an item into index 1:
["Mike navarro","Tina Mari", "Miguel saba","Maria Rami"]
Once the user enters an option, your program should execute the code for that option and displays the list before and after the operations. Make sure to use a while loop so the program
runs until user enters the quit option.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd451f972-af09-4f22-bc9e-c3f92099038f%2F17f46336-d51c-4355-83cd-eff156651f13%2F7fgofuu_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

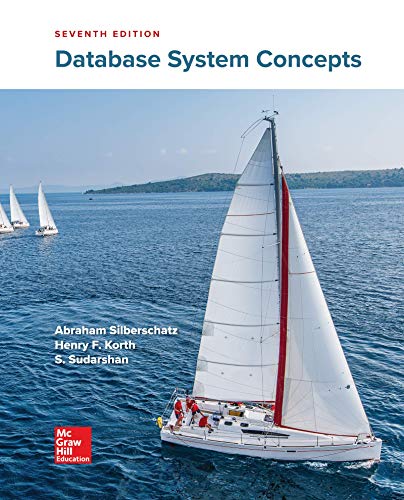
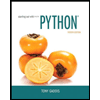
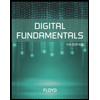
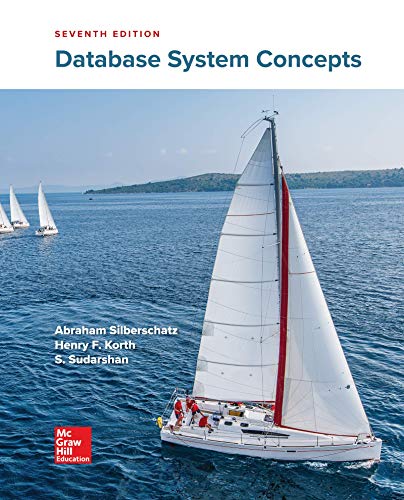
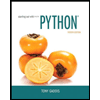
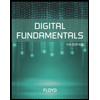
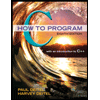
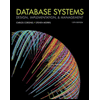
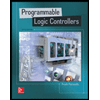