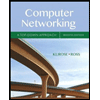
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Python
![Binary search can be implemented as a recursive algorithm. Each call makes a recursive call on one-half of the list the call received as an
argument.
Complete the recursive function binary_search() with the following specifications:
1. Parameters:
o a list of integers
o a target integer
o lower and upper bounds within which the recursive call will search
2. Return value:
o if found, the index within the list where the target is located
o -1 if target is not found
The algorithm begins by choosing an index midway between the lower and upper bounds.
1. If target == nums[index] return index
2. If lower == upper, return lower if target == nums[lower] else -1 to indicate not found
3. Otherwise call the function recursively with half the list as an argument:
o If nums [index] < target, search the list from index to upper
o If nums [index] > target, search the list from lower to index
The list must be ordered, but duplicates are allowed.
the search algorithm works correctly, add the following to binary_search():
4. Count the number of calls to binary_search().
5 Count the number of times when the target is compared to an element of the list. Note: 1lower == upper should not be counted
Hint: Use a global variable to count calls and comparisons.
The input of the program consists of integers on one line followed by a target integer on the second.
The template provides the main program and a helper function that reads a list from input.
Ex: If the input is:
1 234 5 6789
the output is:
index: 1, recursions: 2, comparisons: 3](https://content.bartleby.com/qna-images/question/6046cbb1-e308-4521-9255-72f2b16e119a/ed55dbe5-e8a6-4a93-8369-2836e5f8f7d2/vkxfqro_thumbnail.jpeg)
Transcribed Image Text:Binary search can be implemented as a recursive algorithm. Each call makes a recursive call on one-half of the list the call received as an
argument.
Complete the recursive function binary_search() with the following specifications:
1. Parameters:
o a list of integers
o a target integer
o lower and upper bounds within which the recursive call will search
2. Return value:
o if found, the index within the list where the target is located
o -1 if target is not found
The algorithm begins by choosing an index midway between the lower and upper bounds.
1. If target == nums[index] return index
2. If lower == upper, return lower if target == nums[lower] else -1 to indicate not found
3. Otherwise call the function recursively with half the list as an argument:
o If nums [index] < target, search the list from index to upper
o If nums [index] > target, search the list from lower to index
The list must be ordered, but duplicates are allowed.
the search algorithm works correctly, add the following to binary_search():
4. Count the number of calls to binary_search().
5 Count the number of times when the target is compared to an element of the list. Note: 1lower == upper should not be counted
Hint: Use a global variable to count calls and comparisons.
The input of the program consists of integers on one line followed by a target integer on the second.
The template provides the main program and a helper function that reads a list from input.
Ex: If the input is:
1 234 5 6789
the output is:
index: 1, recursions: 2, comparisons: 3
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Similar questions
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
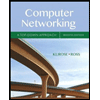
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
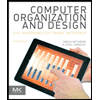
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
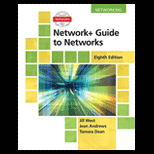
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
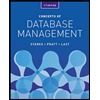
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
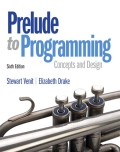
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
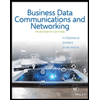
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY