Binary Search Trees
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
C++ PROGRAMMING
Topic: Binary Search Trees
Explain the c++ code below.:
It doesn't have to be long, as long as you explain what the important parts of the code do. (The code is already implemented and correct, only the explanation needed)
It doesn't have to be long, as long as you explain what the important parts of the code do. (The code is already implemented and correct, only the explanation needed)
node* left(node* p) {
return p->left;
}
node* right(node* p) {
return p->right;
}
node* sibling(node* p){
if(p != root){
node* P = p->parent;
if(left(P) != NULL && right(P) != NULL){
if(left(P) == p){
return right(P);
}
return left(P);
}
}
return NULL;
}
node* addRoot(int e) {
if(size != 0){
cout<<"Error"<<endl;
return NULL;
}
root = create_node(e,NULL);
size++;
return root;
}
node* addLeft(node* p, int e) {
if(p->left == NULL){
node* newLeft = create_node(e,NULL);
newLeft->parent = p;
p->left = newLeft;
size++;
return left(p);
}
cout << "Error" << endl;
return NULL;
}
node* addRight(node* p, int e) {
if(p->right == NULL){
node* newRight = create_node(e,NULL);
newRight->parent = p;
p->right = newRight;
size++;
return right(p);
}
cout << "Error" << endl;
return NULL;
}
int _size() {
return size;
}
bool isEmpty() {
return (size==0);
}
int childCount(node* p) {
bool Cright = false;
bool Cleft = false;
if(p->right != NULL){
Cright = true;
}
if(p->left != NULL){
Cleft = true;
}
if(Cleft == true && Cright== true){
return 2;
}
if(Cright){
return 1;
}
if(Cleft){
return -1;
}
return 0;
}
int set(node* p, int e) {
int elem = p->element;
p->element = e;
return elem;
}
node* addSibling(node* p, int e) {
node* P = p->parent;
if(P != NULL){
if(sibling(p) == NULL){
if(sibling(p) == left(P)){
return addLeft(P,e);
}
return addRight(P,e);
}
}
cout<<"Error"<<endl;
return NULL;
}
void clear(){
clear_postorder(root);
}
void attach(node* p, BTree* t1, BTree* t2) {
if(p->right == NULL && p->left == NULL){
if(t1->root != NULL){
t1->root->parent = p;
p->left = t1->root;
}
if(t2->root != NULL){
t2->root->parent = p;
p->right = t2->root;
}
return;
}
cout<<"Error"<<endl;
}
int remove(node* p) {
if(left(p) == NULL || right(p) == NULL){
node* P = NULL;
node* childNode = NULL;
if(p->parent != NULL){
P = p->parent;
}
else{
P = p;
if(left(P) != NULL){
childNode = P->left;
childNode->parent = NULL;
root = childNode;
}
else{
childNode = P->right;
childNode->parent = NULL;
root = childNode;
}
int elem = P->element;
free(P);
size--;
return elem;
}
if(left(P) == p){
if(left(p) != NULL){
P->left = childNode;
childNode = p->left;
if(p->left != NULL || p->right != NULL){
childNode->parent = P;
}
}
else{
childNode = p->right;
P->left = childNode;
if(p->left != NULL || p->right != NULL){
childNode->parent = P;
}
}
}
else{
if(left(p) != NULL){
childNode = p->left;
P->right = childNode;
if(p->left != NULL || p->right != NULL){
childNode->parent = P;
}
}
else{
childNode = p->right;
P->right = childNode;
if(p->left != NULL || p->right != NULL){
childNode->parent = P;
}
}
}
int elem = p->element;
free(p);
return elem;
size--;
}
cout<<"Error"<<endl;
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
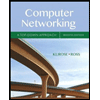
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
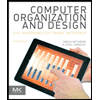
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
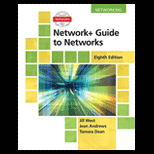
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
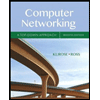
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
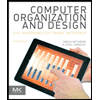
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
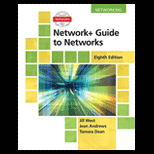
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
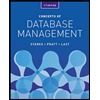
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
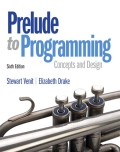
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
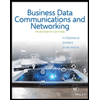
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY