but I continue to get the same error message regardless of what I fix:
I have the following code:
import java.util.*;
class Money {
private int dollar;
private int cent;
final static int MIN_CENT_VALUE=0;
final static int MAX_CENT_VALUE=99;
public Money(){
this.dollar=0;
this.cent=0;
}
public Money(int dollar,int cent){
this.dollar=dollar;
if(cent>=MIN_CENT_VALUE&¢<=MAX_CENT_VALUE){
this.cent=cent;
}else{
cent=0;
}
}
public int getDollar(){
return this.dollar;
}
public void setDollar(int dol){
this.dollar=dol;
}
public int getCent(){
return this.cent;
}
public void setCent(int c){
if(c>=MIN_CENT_VALUE&&c<=MAX_CENT_VALUE){
this.cent=c;
}
}
public Money add(Money otherMoney)
{
Money m = new Money();
m.dollar=dollar + otherMoney.dollar;
m.cent=cent + otherMoney.cent;
if(m.cent >= 100)
{
m.dollar++;
m.cent -= 100;
}
return m;
}
public Money subtract(Money otherMoney)
{
Money m = new Money();
m.dollar=dollar - otherMoney.dollar;
m.cent=cent -otherMoney.cent;
if(m.dollar<0||m.cent<0){
return null;
}
return m;
}
public boolean equals(Money otherMoney){
return this==otherMoney;
}
public int compareTo(Money otherMoney){
if(this.dollar<otherMoney.dollar){
return 1;
}else if(this.dollar==otherMoney.dollar){
return 0;
}else{
return -1;
}
}
public String toString(){
return "Dollar is "+this.dollar+" and cents is "+ this.cent;
}
public static Money max(Money[] m)
{
int max = m[0].dollar;
int index = 0;
for(int i=0;i<m.length;i++)
{
if(max < m[i].dollar)
{
max = m[i].dollar;
index = i;
}
}
return m[index];
}
public static void selectionSort(Money arr[])
{
int n = arr.length;
for (int i = 0; i < n-1; i++)
{
int min = i;
for (int j = i+1; j < n; j++)
if (arr[j].dollar < arr[min].dollar)
min = j;
// Swap the found minimum element with the first element
Money temp = new Money();
temp = arr[min];
arr[min] = arr[i];
arr[i] = temp;
}
}
public static void printList(Money[] m) //This method takes an array of object as parameter
{
for(Money mm : m)
System.out.println(mm);
}
}
________________________________________________________
to be used with the following driver program:
import java.util.*;
public class TestMoney
{
public static void main(String[] args)
{
Random rnd = new Random(10);
Money m1 = new Money(5,45);
Money m2 = new Money(10,95);
m1.setCent(101);
Money sum = m1.add(m2);
System.out.println(m1+" + "+m2+" is "+sum);
Money diff = m1.subtract(m2);
System.out.println(m1+" - "+m2+" is "+diff);
diff = m2.subtract(m1);
System.out.println(m2+" - "+m1+" is "+diff);
System.out.println("equals method result: "+m1.equals(m2));
System.out.println("compareTo method result: "+m1.compareTo(m2));
Money[] list = new Money[15];
// Generate 15 random Money objects
for(int i=0;i<list.length;++i)
{
list[i] = new Money((int)(1+rnd.nextInt(30)),rnd.nextInt(100));
}
// print the list of Money objects, 10 per line
System.out.println("Unsorted list");
Money.printList(list,10);
// Find the largest Money object
System.out.println("The Max is "+Money.max(list));
// Sort and print
System.out.println("Sorted list");
Money.selectionSort(list);
Money.printList(list,10);
}
}
________________________________________
but I continue to get the same error message regardless of what I fix:
![Failed to compile
TestMoney.java:24: warning: [cast] redundant cast to int
list[i] = new Money ((int) (1+rnd.nextInt (30)), rnd.nextInt (100));
TestMoney.java:28: error: method printList in class Money cannot be applied to given types;
Money.printList (list, 10);
required: Money []
found: Money [], int
reason: actual and formal argument lists differ in length
TestMoney.java:34: error: method printList in class Money cannot be applied to given types;
Money.printList (list, 10);
required: Money []
found: Money [], int
reason: actual and formal argument lists differ in length
2 errors
1 warning](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe4e826cb-c133-47e8-aeaa-858e7b3a09da%2Fdb0c65bf-dc93-48a6-a53f-3383cf61cc96%2Fb0qdee5_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 2 images

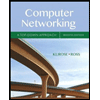
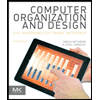
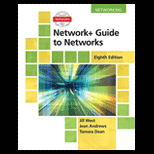
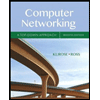
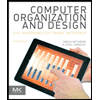
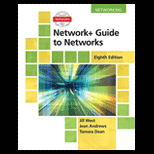
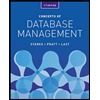
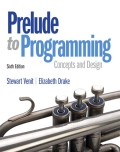
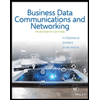