By given the following models and database context classes, answer the following questions or complete the missing codes: using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.ComponentModel.DataAnnotations.Schema; using System.ComponentModel.DataAnnotations; namespace UniversityAssociationMvc.Models { public class Student { public int StudentID { get; set; } public string LastName { get; set; } public string FirstName { get; set; } public string Phone { get; set; } public string Email { get; set; } public DateTime RegistrationDate { get; set; } public virtual ICollection Memberships { get; set; } } } using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.ComponentModel.DataAnnotations; namespace UniversityAssociationMvc.Models { public class Club { public int ClubID { get; set; } public string ClubName { get; set; } public DateTime OpenDate { get; set; } public decimal RegistrationFee { get; set; } public virtual Center Center { get; set; } public virtual ICollection Memberships { get; set; } public virtual ICollection Managers { get; set; } } } using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.ComponentModel.DataAnnotations; namespace UniversityAssociationMvc.Models { public class Membership { public int MembershipID { get; set; } public int ClubID { get; set; } public int StudentID { get; set; } public DateTime ExpireDate { get; set; } public virtual Student student { get; set; } public virtual Club club { get; set; } } } using System.Data.Entity; using UniversityAssociationMvc.Models; namespace UniversityAssociationMvc.Models { public class UniversityAssociationDB : DbContext { public UniversityAssociationDB() : base("name=UniversityAssociationDB") { } public DbSet Students { get; set; } public DbSet Managers { get; set; } public DbSet Clubs { get; set; } public DbSet Memberships { get; set; } public DbSet Centers { get; set; } } } Assume the Index view for Home controller lists all clubs with details for user to choose one for registration. Please complete the following HomeController class to fulfil the following requirements: If searchName is given and is not an empty string, only those clubs whose name contains searchNamewill be displayed. If maxRegistrationFee is not 0, only those clubs whose registration fee is less than or equal to maxRegistrationFee will be displayed. If minRegistrationFee is not 0, only those clubs whose registration fee is more than or equal to minRegistrationFee will be displayed. using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Mvc; namespace UniversityAssociationMvc.Controllers { public class HomeController : Controller { UniversityAssociationDB db = new UniversityAssociationDB(); public ActionResult Index() { Q1 .______________ } [HttpPost] public ActionResult Index(string searchName, decimal maxRegistrationFee, decimal minRegistrationFee) { //Complete this part to fulfil the requirements. Q2 . __________________ } } } There is an Edit view for Student controller. A student user can modify his/her profile in this page. Please complete the following methods in StudentController class to derive the function for editing: using System; using System.Collections.Generic; using System.Data; using System.Data.Entity; using System.Linq; using System.Web; using System.Web.Mvc; using UniversityAssociationMvc.Models; namespace UniversityAssociationMvc.Controllers { public class StudentController : Controller { private UniversityAssociationDB db = new UniversityAssociationDB(); /* Other part of the class */ public ActionResult Edit(int id = 0) { Q3 .________________ } [HttpPost] public ActionResult Edit(Student student) { Q4._____________________ } } }
By given the following models and
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.ComponentModel.DataAnnotations.Schema;
using System.ComponentModel.DataAnnotations;
namespace UniversityAssociationMvc.Models
{
public class Student
{
public int StudentID { get; set; }
public string LastName { get; set; }
public string FirstName { get; set; }
public string Phone { get; set; }
public string Email { get; set; }
public DateTime RegistrationDate { get; set; }
public virtual ICollection<Membership> Memberships { get; set; }
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.ComponentModel.DataAnnotations;
namespace UniversityAssociationMvc.Models
{
public class Club
{
public int ClubID { get; set; }
public string ClubName { get; set; }
public DateTime OpenDate { get; set; }
public decimal RegistrationFee { get; set; }
public virtual Center Center { get; set; }
public virtual ICollection<Membership> Memberships { get; set; }
public virtual ICollection<President> Managers { get; set; }
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.ComponentModel.DataAnnotations;
namespace UniversityAssociationMvc.Models
{
public class Membership
{
public int MembershipID { get; set; }
public int ClubID { get; set; }
public int StudentID { get; set; }
public DateTime ExpireDate { get; set; }
public virtual Student student { get; set; }
public virtual Club club { get; set; }
}
}
using System.Data.Entity;
using UniversityAssociationMvc.Models;
namespace UniversityAssociationMvc.Models
{
public class UniversityAssociationDB : DbContext
{
public UniversityAssociationDB() : base("name=UniversityAssociationDB")
{
}
public DbSet<Student> Students { get; set; }
public DbSet<President> Managers { get; set; }
public DbSet<Club> Clubs { get; set; }
public DbSet<Membership> Memberships { get; set; }
public DbSet<Center> Centers { get; set; }
}
}
Assume the Index view for Home controller lists all clubs with details for user to choose one for registration. Please complete the following HomeController class to fulfil the following requirements:
- If searchName is given and is not an empty string, only those clubs whose name contains searchNamewill be displayed.
- If maxRegistrationFee is not 0, only those clubs whose registration fee is less than or equal to maxRegistrationFee will be displayed.
- If minRegistrationFee is not 0, only those clubs whose registration fee is more than or equal to minRegistrationFee will be displayed.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace UniversityAssociationMvc.Controllers
{
public class HomeController : Controller
{
UniversityAssociationDB db = new UniversityAssociationDB();
public ActionResult Index()
{
Q1 .______________
}
[HttpPost]
public ActionResult Index(string searchName, decimal maxRegistrationFee, decimal minRegistrationFee)
{
//Complete this part to fulfil the requirements.
Q2 . __________________
}
}
}
There is an Edit view for Student controller. A student user can modify his/her profile in this page. Please complete the following methods in StudentController class to derive the function for editing:
using System;
using System.Collections.Generic;
using System.Data;
using System.Data.Entity;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using UniversityAssociationMvc.Models;
namespace UniversityAssociationMvc.Controllers
{
public class StudentController : Controller
{
private UniversityAssociationDB db = new UniversityAssociationDB();
/*
Other part of the class
*/
public ActionResult Edit(int id = 0)
{
Q3 .________________
}
[HttpPost]
public ActionResult Edit(Student student)
{
Q4._____________________
}
}
}

Step by step
Solved in 2 steps with 9 images

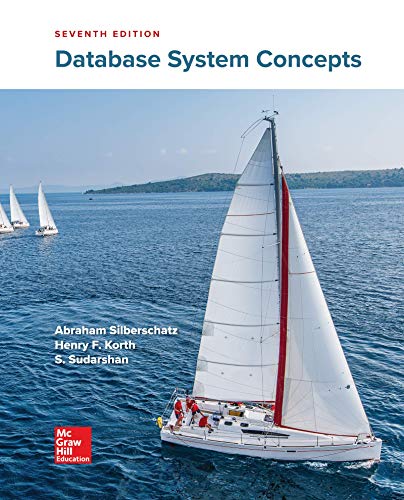
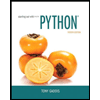
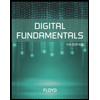
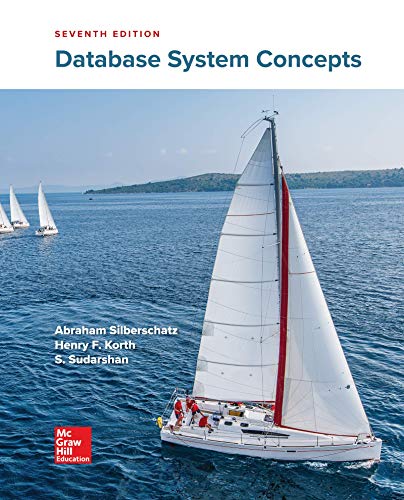
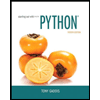
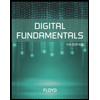
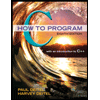
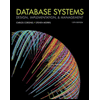
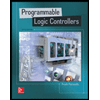