c++ a. run program as is. b. change it so the use have option to input the key (target) save 2 program for end of semester gradin. c. implement sequential search to you student record program, and look for a stundet name, then display all records (name, grades, sum, average, letter grade of that student, if found) #include #include using namespace std; const int SIZE = 12; /*======================= Prototype Declarations ====================================== */ int seqSearch(int list[], int last, int target, int& locn); // 4 parameters , array, size, target and location //========================================== main ==================================== int main() { /* Local Declarations */ int i; // Loop control variable int locn; // Another variable to hold / store index if it is found int ary[SIZE] = { 4, 21, 36, 14, 62, 91, 8, 22, 7, 81, 77, 10 }; //array is initialized cout << "\nIndexes : "; // Print the index for better visualization on out put for (i = 0; i < SIZE; i++) cout << setw(3) << i; cout << "\nList data: "; for (i = 0; i < SIZE; i++) // Print the array for use to see it on screen cout << setw(3) << ary[i]; cout << endl; if (seqSearch(ary, SIZE - 1, 9, locn)) // Calling finction pass proper arguments, and some items(target) cout << "Found 9 at location " << locn << endl; // target 1 , is number 9 else cout << " 9 Not Found "<< endl; if (seqSearch(ary, SIZE - 1, 19, locn)) cout << "Found 19 at location " << locn << endl; // target 1 , is number 19 else cout << "19 Not Found at " << endl; if (seqSearch(ary, SIZE - 1, 10, locn)) // target 1 , is number 10 cout << "Found 10 at location " << locn << endl; else cout << "10 Not Found at " << locn << endl; if (seqSearch(ary, SIZE - 1, 0, locn)) cout << "Found 0 at location " << endl; // target 1 , is number 0 else cout << "0 Not Found at " << locn << endl; cout << endl; return 0; } /* Locate the target in an unordered list of size elements. Pre: list must contain at least one item.last contains index to last element in list target contains the data to be located Post: FOUND: matching index stored in locn address return 1 (found) NOT FOUND: last stored in locn address. return 0 (not found) */ int seqSearch(int list[], int last, int target, int& locn) { // Local Declarations int looker; // looker is a variable that starts at index 0, and test it with last or size looker = 0; // initialize looker while (looker < last && target != list[looker]) looker++; //increment the loop variable and test next item in the array locn = looker; // set the looker (loop variable) and return (target == list[looker]); //send message that it is same as target and it is found }
c++
a. run program as is.
b. change it so the use have option to input the key (target)
save 2 program for end of semester gradin.
c. implement sequential search to you student record program, and look for a stundet name, then display all records (name, grades, sum, average, letter grade of that student, if found)
#include <iostream>
#include <iomanip >
using namespace std;
const int SIZE = 12;
/*======================= Prototype Declarations ====================================== */
int seqSearch(int list[], int last, int target, int& locn); // 4 parameters , array, size, target and location
//========================================== main ====================================
int main()
{ /* Local Declarations */
int i; // Loop control variable
int locn; // Another variable to hold / store index if it is found
int ary[SIZE] = { 4, 21, 36, 14, 62, 91, 8, 22, 7, 81, 77, 10 }; //array is initialized
cout << "\nIndexes : "; // Print the index for better visualization on out put
for (i = 0; i < SIZE; i++)
cout << setw(3) << i;
cout << "\nList data: ";
for (i = 0; i < SIZE; i++) // Print the array for use to see it on screen
cout << setw(3) << ary[i];
cout << endl;
if (seqSearch(ary, SIZE - 1, 9, locn)) // Calling finction pass proper arguments, and some items(target)
cout << "Found 9 at location " << locn << endl; // target 1 , is number 9
else
cout << " 9 Not Found "<< endl;
if (seqSearch(ary, SIZE - 1, 19, locn))
cout << "Found 19 at location " << locn << endl; // target 1 , is number 19
else
cout << "19 Not Found at " << endl;
if (seqSearch(ary, SIZE - 1, 10, locn)) // target 1 , is number 10
cout << "Found 10 at location " << locn << endl;
else
cout << "10 Not Found at " << locn << endl;
if (seqSearch(ary, SIZE - 1, 0, locn))
cout << "Found 0 at location " << endl; // target 1 , is number 0
else
cout << "0 Not Found at " << locn << endl;
cout << endl;
return 0;
}
/* Locate the target in an unordered list of size elements.
Pre: list must contain at least one item.last contains index to last element in list target contains the data to be located
Post: FOUND: matching index stored in locn address return 1 (found)
NOT FOUND: last stored in locn address. return 0 (not found)
*/
int seqSearch(int list[], int last, int target, int& locn)
{ // Local Declarations
int looker; // looker is a variable that starts at index 0, and test it with last or size
looker = 0; // initialize looker
while (looker < last && target != list[looker])
looker++; //increment the loop variable and test next item in the array
locn = looker; // set the looker (loop variable) and
return (target == list[looker]); //send message that it is same as target and it is found
}

Step by step
Solved in 4 steps

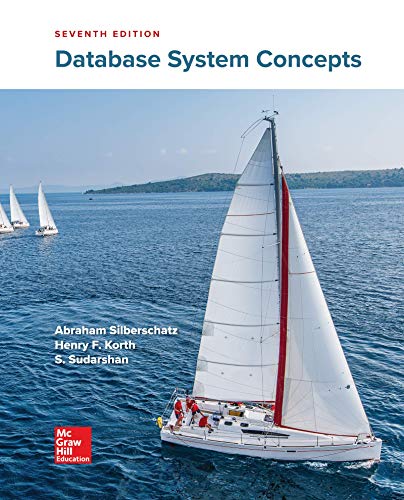
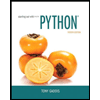
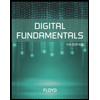
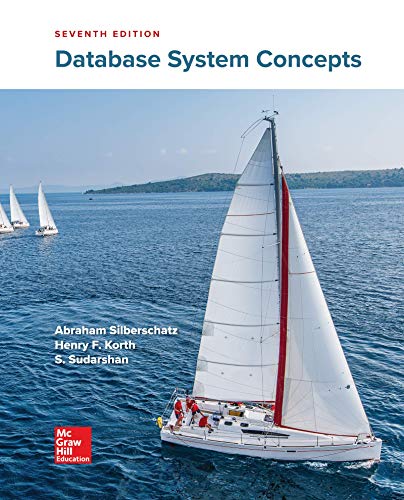
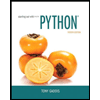
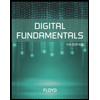
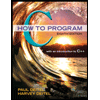
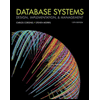
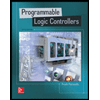