C++ Implement a Rational Number class with the following specifications. Data members a) numerator and denominator Functions a) Constructors: 1) default constructor 2) single parameter constructor to create numerator/1 3) dual parameter constructor to create numerator/denominator 4) Use constructor delegation across all constructors. b) Accessors and Mutators for both data members. c) Static recursive GCD function using Euclid’s algorithm. d) Static LCM function for two numbers. e) Reduce function simplify a rational number. This function modifies its calling object. f) Your program should work with the supplied driver program Notes LCM (Least Common Multiple) This function returns the smallest multiple of a and b. Step 1: Multiply a and b to find a common multiple. Step 2: Divide the common multiple by the GCD of a and b. Step 3: Return the result of Step 2. Reduce: This function reduces a fraction to simplest terms (i.e. 9/12 to 3/4). Step 1: Find the GCD of the numerator and denominator. Step 2: Divide the numerator by GCD and store as the new numerator. Step 3: Divide the denominator by GCD and store as the new denominator. Static Functions Recall that static functions are class functions and not associated with instances of the class (objects). In this class, the static functions GCD and LCM should accept inputs any input pair (a and b) and return an answer based upon that input pair. As such, these functions can be used by the programmer upon Rational Number objects or random values for a and b. Use the following main function to test your program. (have to use this main function) (From here please follow the image that i attached & make sure the output looks same as the image) (Please make sure output comes same as the image and share me the code & output please)
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
C++
Implement a Rational Number class with the following specifications.
Data members
a) numerator and denominator
Functions
a) Constructors:
1) default constructor
2) single parameter constructor to create numerator/1
3) dual parameter constructor to create numerator/denominator
4) Use constructor delegation across all constructors.
b) Accessors and Mutators for both data members.
c) Static recursive GCD function using Euclid’s
d) Static LCM function for two numbers.
e) Reduce function simplify a rational number. This function modifies its calling object.
f) Your
Notes
LCM (Least Common Multiple)
This function returns the smallest multiple of a and b.
Step 1: Multiply a and b to find a common multiple.
Step 2: Divide the common multiple by the GCD of a and b.
Step 3: Return the result of Step 2.
Reduce:
This function reduces a fraction to simplest terms (i.e. 9/12 to 3/4).
Step 1: Find the GCD of the numerator and denominator.
Step 2: Divide the numerator by GCD and store as the new numerator.
Step 3: Divide the denominator by GCD and store as the new denominator.
Static Functions
Recall that static functions are class functions and not associated with instances of the class (objects). In this class, the static functions GCD and LCM should accept inputs any input pair (a and b) and return an answer based upon that input pair. As such, these functions can be used by the programmer upon Rational Number objects or random values for a and b.
Use the following main function to test your program. (have to use this main function)
(From here please follow the image that i attached & make sure the output looks same as the image)
(Please make sure output comes same as the image and share me the code & output please)
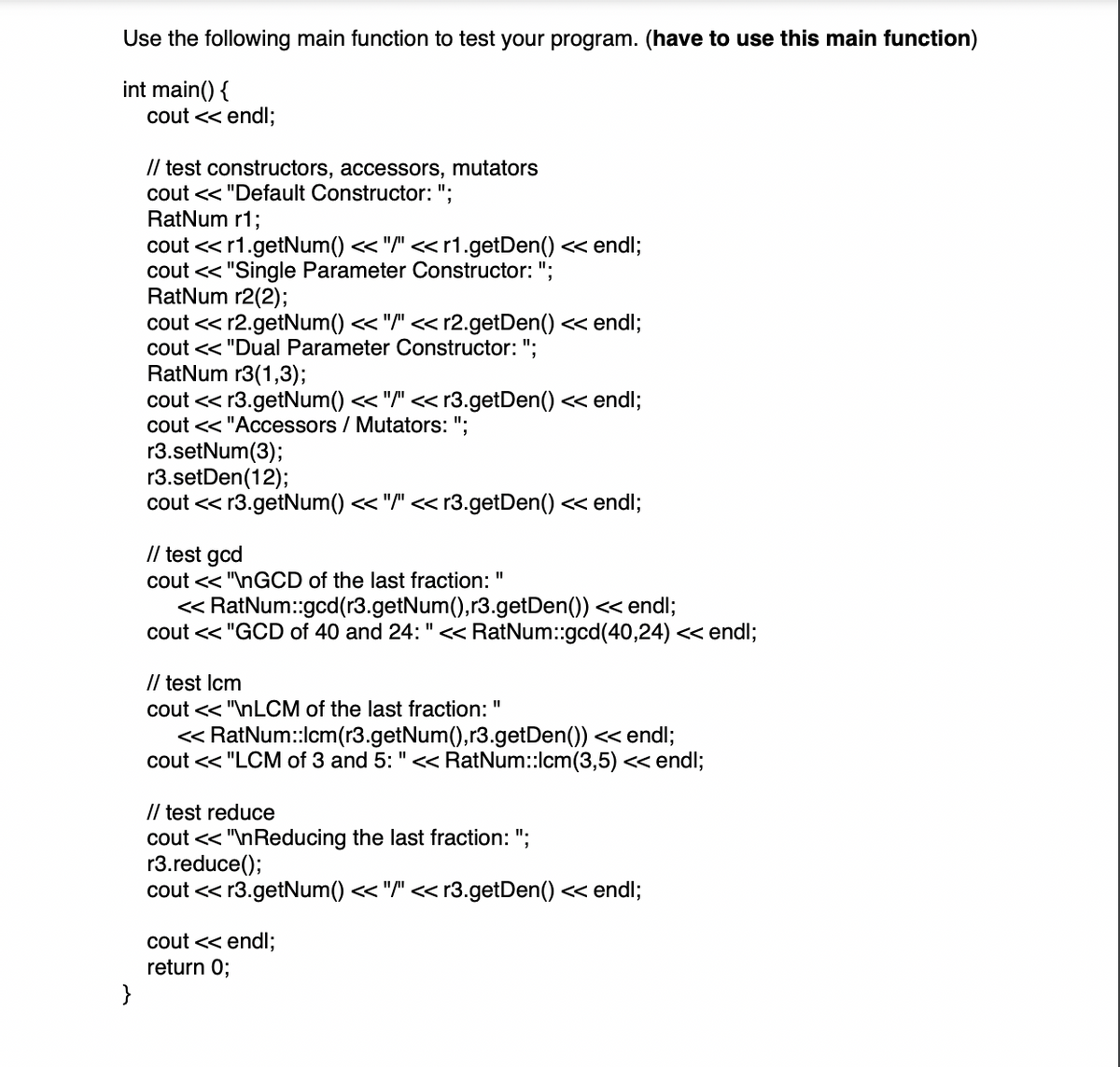
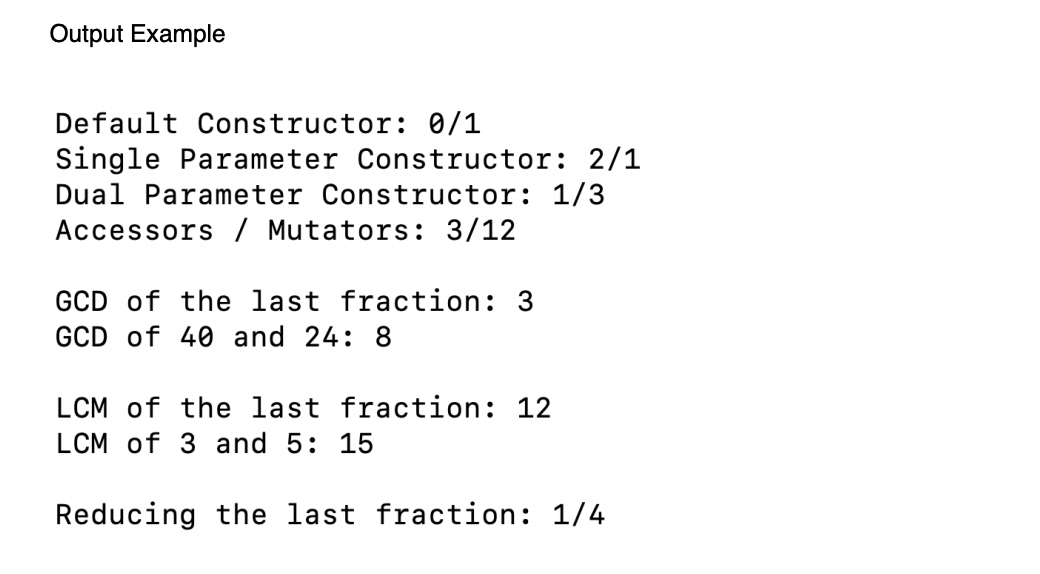

In this question we have to write a C++ program for implementing a Rational Number class in C++. The task is to create a class that represents rational numbers (fractions) with a numerator and denominator, and to implement various member functions such as constructors, accessors, mutators, a static recursive GCD function using Euclid's algorithm, a static LCM function, and a reduce function to simplify a rational number. The provided main function tests the implementation of these functions.
Let's code and hope this helps if you find any query on the solution utilize threaded question feature.
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

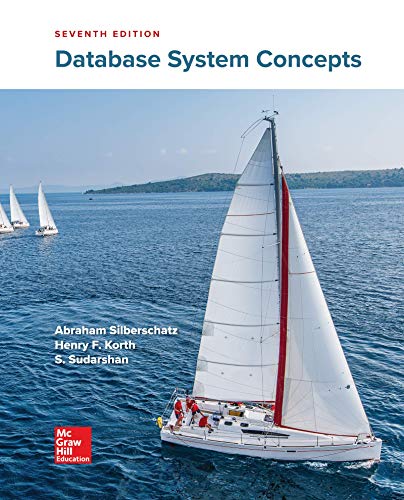
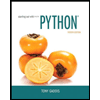
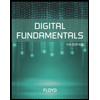
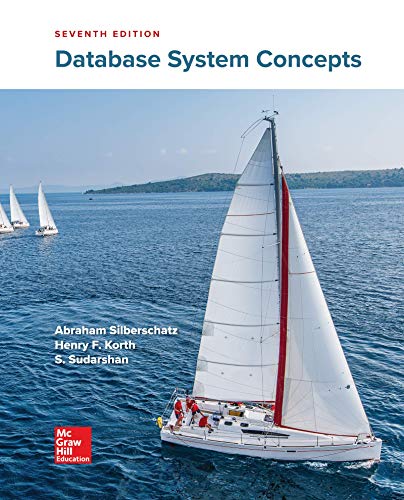
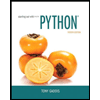
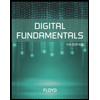
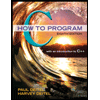
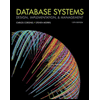
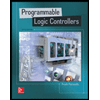