C++ In this task, you are required to write a code that converts an Octal number into its equivalent Decimal value or vice versa. The user first selects the conversion choice by inserting 1 or 2. Choice 1 is followed by inserting an Octal integer and getting its Decimal equivalent value as an output. Choice 2 requires inserting a Decimal integer and prints out its Octal equivalent. The Octal numeral system, is the base-8 number system, and uses the digits 0 to 7, that is to say 10 octal represents 8 Decimal and 100 octal represents 64 Decimal. Convertion from an Octal integer into Decimal In the Decimal system, each number is the digit multiplied by 10 to the power of its location (starting from 0). For example: 74 decimal = 7 x 10^1 + 4 x 10^0 1252 decimal = 1 x 10^3 + 2 x 10^2 + 5 x 10^1 + 2 x 10^0 each number is the digit multiplied by 8 to the power of its location (starting from 0). For example: 112 octal = 1 x 8^2 + 1 x 8^1 + 2 x 8^0 Converting an Octal integer into Decimal can be done by performing the last calculation. Hence, 112 in Octal is equal to 64 + 8 + 2 = 74 in Decimal. Conversion from a Decimal integer into Octal On the other hand, to convert a Decimal integer into Octal, follow the follwoing steps: Divide the number by 8, you get a quotient and a remainder. Take note of the remainder. It can be any number between 0 to 7 . This number will be the last digit of the octal number, i.e., the rightmost digit. Use the quotient from step 1 as your new initial number. Repeat steps 1-3, and keep concatenating the remainder to the left of the previously obtained digits. Stop when the quaotient is zero. Example: convert Decimal number 6521 to octal representation:
C++
In this task, you are required to write a code that converts an Octal number into its equivalent Decimal value or vice versa. The user first selects the conversion choice by inserting 1 or 2.
Choice 1 is followed by inserting an Octal integer and getting its Decimal equivalent value as an output.
Choice 2 requires inserting a Decimal integer and prints out its Octal equivalent.
The Octal numeral system, is the base-8 number system, and uses the digits 0 to 7, that is to say 10 octal represents 8 Decimal and 100 octal represents 64 Decimal.
Convertion from an Octal integer into Decimal
In the Decimal system, each number is the digit multiplied by 10 to the power of its location (starting from 0). For example:
74 decimal = 7 x 10^1 + 4 x 10^0
1252 decimal = 1 x 10^3 + 2 x 10^2 + 5 x 10^1 + 2 x 10^0
each number is the digit multiplied by 8 to the power of its location (starting from 0). For example:
112 octal = 1 x 8^2 + 1 x 8^1 + 2 x 8^0
Converting an Octal integer into Decimal can be done by performing the last calculation. Hence, 112 in Octal is equal to 64 + 8 + 2 = 74 in Decimal.
Conversion from a Decimal integer into Octal
On the other hand, to convert a Decimal integer into Octal, follow the follwoing steps:
Divide the number by 8, you get a quotient and a remainder.
Take note of the remainder. It can be any number between 0 to 7 . This number will be the last digit of the octal number, i.e., the rightmost digit.
Use the quotient from step 1 as your new initial number.
Repeat steps 1-3, and keep concatenating the remainder to the left of the previously obtained digits. Stop when the quaotient is zero.
Example: convert Decimal number 6521 to octal representation:
Divide by 8, i.e., 6521/8 = 815, remainder is 1.
Now, we will use the quotient from step 1, i.e., 815 as the new number, and repeat step 1.
We will continue repeating the above steps until we get 0 as the quotient.
System Message: WARNING/2 (<string>, line 35)
Block quote ends without a blank line; unexpected unindent.
Reading the remainders in reverse order, the number 14571 is the Octal value for 6521 in Decimal
Important Notes:
If the inserted choice is wrong, the output will be "Invalid".
If the inserted Octal or Decimal number is a negative value, the output will be " Negative".
If the inserted Octal number has at least one digit which is 8 or 9, the output will be "Invalid".
You are not allowed to add any additional headers/libraries to the code
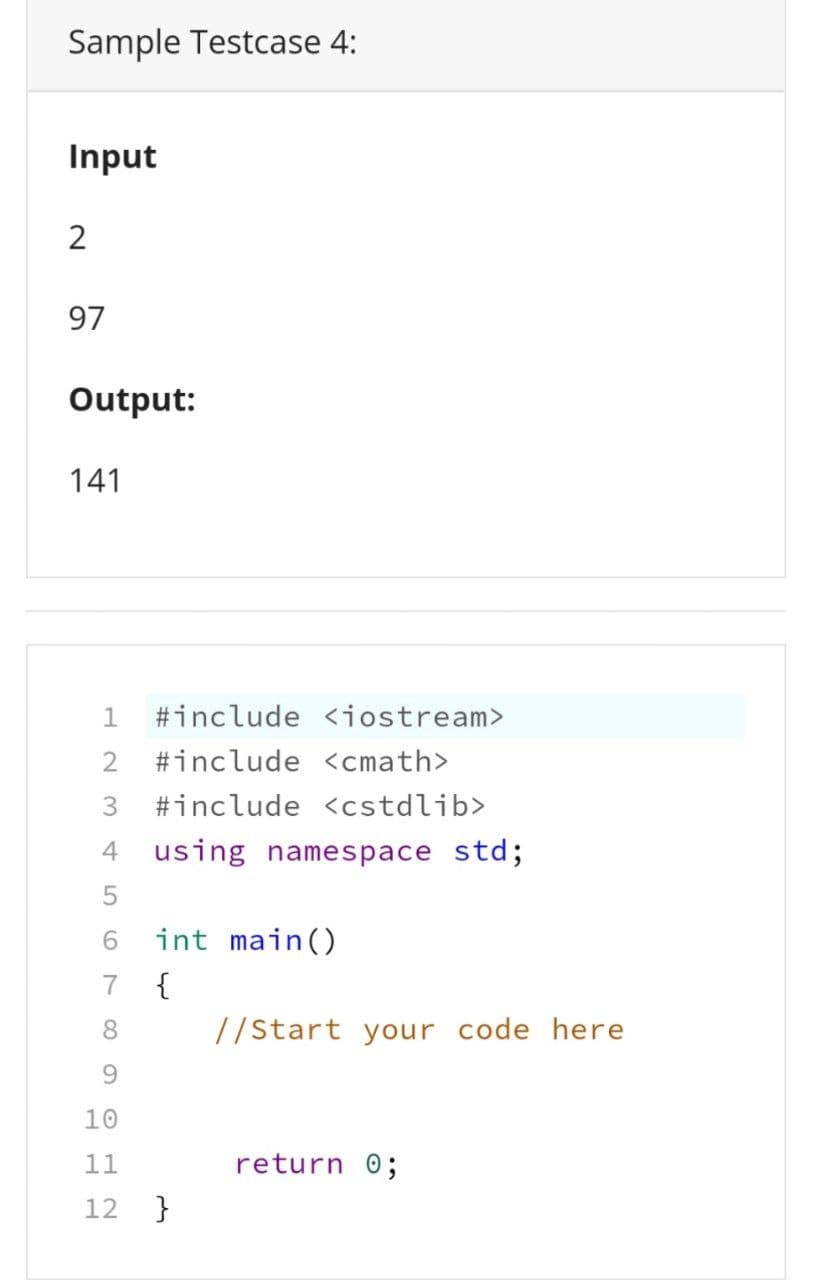
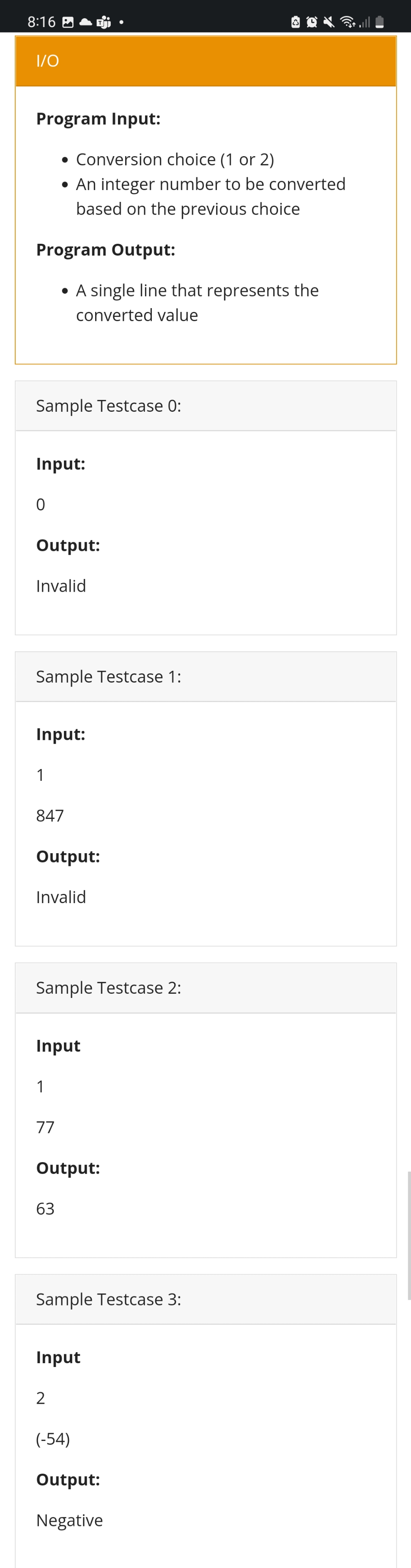

Step by step
Solved in 4 steps with 6 images

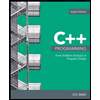
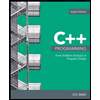