C++ Programming: I need help writing a void function that prints sales details using 3 for loops. Please see my code below ... not sure how to write the function. But this is what i've got so far. Please leave main alone. What the output should look like is posted below the code. Thanks :) #include #include #include using namespace std; int const LOCATION_DIM_SZ = 2; int const TIME_DIM_SZ = 2; int const PRODUCT_DIM_SIZE = 3; string Location[] = { "FL", "TX" }; string Period[] = { "Jan", "FEB" }; string Product[] = { "iPhone","iPad","MacBookPro" }; /*1*/ void printSalesDetails(double sales[LOCATION_DIM_SZ][TIME_DIM_SZ][PRODUCT_DIM_SIZE]) { int i(0), j(0), k(0); int sum = 0; cout << "Sales Details per Location per Period per Product" << endl; cout << "=================================================" << endl; for (i = 0; i < 2; i++){ double salesPerLocation = 0; sum = 0; for (j = 0; j < 2; j++){ double salesPerPeriod = 0; for (k = 0; k < 3; k++){ double salesPerProduct = 0; sum += sales[i][k][j]; } } cout << "Sales for location " << Location[i] << '\\' << Period[i] << '\\' << Product[i] << "\t=$" << sum << endl; } cout << "===================================================" << endl; } int main(int argc, const char* argv[]) { double sales[LOCATION_DIM_SZ][TIME_DIM_SZ][PRODUCT_DIM_SIZE] = { 0 }; sales[0][0][0] = 45; sales[0][0][1] = 8; sales[0][0][2] = 4; sales[0][1][0] = 35; sales[0][1][1] = 10; sales[0][1][2] = 5; sales[1][0][0] = 75; sales[1][0][1] = 15; sales[1][0][2] = 13; sales[1][1][0] = 45; sales[1][1][1] = 17; sales[1][1][2] = 23.1; printSalesDetails(sales); return 0; } DESIRED OUTPUT: Sales Details per Location per Period per Product ================================================sales for Location FL/Jan/iPhone =$ 45.00 millions sales for Location FL/Jan/iPad =$ 8.00 millions sales for Location FL/Jan/MacBookPro =$ 4.00 millions sales for Location FL/FEB/iPhone =$ 35.00 millions sales for Location FL/FEB/iPad =$ 10.00 millions sales for Location FL/FEB/MacBookPro =$ 5.00 millions sales for Location TX/Jan/iPhone =$ 75.00 millions sales for Location TX/Jan/iPad =$ 15.00 millions sales for Location TX/Jan/MacBookPro =$ 13.00 millions sales for Location TX/FEB/iPhone =$ 45.00 millions sales for Location TX/FEB/iPad =$ 17.00 millions sales for Location TX/FEB/MacBookPro =$ 23.10 millions Sum of Sales for all Apple =$ 295 millions
C++ Programming:
I need help writing a void function that prints sales details using 3 for loops. Please see my code below ... not sure how to write the function. But this is what i've got so far. Please leave main alone. What the output should look like is posted below the code. Thanks :)
#include <iostream>
#include <string>
#include <iomanip>
using namespace std;
int const LOCATION_DIM_SZ = 2;
int const TIME_DIM_SZ = 2;
int const PRODUCT_DIM_SIZE = 3;
string Location[] = { "FL", "TX" };
string Period[] = { "Jan", "FEB" };
string Product[] = { "iPhone","iPad","MacBookPro" };
/*1*/
void printSalesDetails(double sales[LOCATION_DIM_SZ][TIME_DIM_SZ][PRODUCT_DIM_SIZE]) {
int i(0), j(0), k(0);
int sum = 0;
cout << "Sales Details per Location per Period per Product" << endl;
cout << "=================================================" << endl;
for (i = 0; i < 2; i++){
double salesPerLocation = 0;
sum = 0;
for (j = 0; j < 2; j++){
double salesPerPeriod = 0;
for (k = 0; k < 3; k++){
double salesPerProduct = 0;
sum += sales[i][k][j];
}
}
cout << "Sales for location " << Location[i] << '\\' << Period[i] << '\\' << Product[i] << "\t=$" << sum << endl;
}
cout << "===================================================" << endl;
}
int main(int argc, const char* argv[]) {
double sales[LOCATION_DIM_SZ][TIME_DIM_SZ][PRODUCT_DIM_SIZE] = { 0 };
sales[0][0][0] = 45;
sales[0][0][1] = 8;
sales[0][0][2] = 4;
sales[0][1][0] = 35;
sales[0][1][1] = 10;
sales[0][1][2] = 5;
sales[1][0][0] = 75;
sales[1][0][1] = 15;
sales[1][0][2] = 13;
sales[1][1][0] = 45;
sales[1][1][1] = 17;
sales[1][1][2] = 23.1;
printSalesDetails(sales);
return 0;
}
DESIRED OUTPUT:
Sales Details per Location per Period per Product ================================================sales for Location FL/Jan/iPhone =$ 45.00 millions
sales for Location FL/Jan/iPad =$ 8.00 millions
sales for Location FL/Jan/MacBookPro =$ 4.00 millions
sales for Location FL/FEB/iPhone =$ 35.00 millions
sales for Location FL/FEB/iPad =$ 10.00 millions
sales for Location FL/FEB/MacBookPro =$ 5.00 millions
sales for Location TX/Jan/iPhone =$ 75.00 millions
sales for Location TX/Jan/iPad =$ 15.00 millions
sales for Location TX/Jan/MacBookPro =$ 13.00 millions
sales for Location TX/FEB/iPhone =$ 45.00 millions
sales for Location TX/FEB/iPad =$ 17.00 millions
sales for Location TX/FEB/MacBookPro =$ 23.10 millions
Sum of Sales for all Apple =$ 295 millions

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

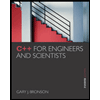
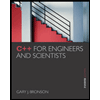