C# programming question. This task will be a car dealership where you’ll help the user find the car(s) they want. This program should keep going until the user either decides to not continue with the purchase, or the purchase is complete, and no more order is being processed. You will need 3 structs to store: • client information • order information • used_car information Each struct would have the following fields: struct used_car { int carID; char brand[length]; char make[length]; int year; int mileage; float price; }; struct order { int numCosigned; int carID; float pricePerPerson; }; struct client { char firstName[length]; char lastName[length]; bool isEmployed; int creditScore; }; I. global variables: • Create an array of struct used_car and add some cars in there. I put 4 cars. You can do as many as you want (at least 3). • Make sure to put the struct definition above the array creation, otherwise, it won’t work. o This is similar to you trying to call a function X that’s not declared above of main or any other function that calls X. II. main(): • Print a welcome message. • Call a function that utilize all the cars available (from array created above) and print them, so the client knows what options they have. • Ask them to choose the which car they want. They can input their answer based on the carID field from available_car struct. o Make sure to check for invalid answers. Ask for reinput until it’s valid. o I made my ID from 1->N. § You can tell them to input 0 if they don’t want to buy anything. § If this is the case, write some message and quit the program. • Ask the user if they will be the sole owner or will they cosign and save that info into order structure. o We assume that if there are 2 co-signers, each will pay 50%, 3 co-signers would be 33.33% each, and so on... • Print the price for the car that they have chosen, calculate how much EACH cosigner has to pay, and ask them if you are okay with this price and would like to process o If they answer No, write some message and quit the program. o If they answer Yes, § call another function to get the co-signers information (struct client) § when done getting the info, call another function to print back all the clients information § you can also print the price per person in the end when printing out each person’s cost. It’s up to you. III. other functions (it’ up to you to have more functions, I’m giving the 3 required functions. Doesn’t have to be exactly the same. This is a guide.): § printCars() function: o prints all the used cars the dealership have from the global array. § getClientInfo() function (or whatever you want to call it): o take an order struct o create an array of struct client. o loop through each client and get their information (name, creditScore,...) and save the info in the struct client array. o call printClientInfo() function to print back the information after. (You should not print the information right after the scanning the information, it should be done at the end and all the passengers info are printed together). § printClientInfo() function (or whatever you want to call it): o take an array of struct client o loop through each client and print out their information
C# programming question. This task will be a car dealership where you’ll help the user find the car(s) they want. This program should keep going until the user either decides to not continue with the purchase, or the purchase is complete, and no more order is being processed. You will need 3 structs to store: • client information • order information • used_car information Each struct would have the following fields: struct used_car { int carID; char brand[length]; char make[length]; int year; int mileage; float price; }; struct order { int numCosigned; int carID; float pricePerPerson; }; struct client { char firstName[length]; char lastName[length]; bool isEmployed; int creditScore; }; I. global variables: • Create an array of struct used_car and add some cars in there. I put 4 cars. You can do as many as you want (at least 3). • Make sure to put the struct definition above the array creation, otherwise, it won’t work. o This is similar to you trying to call a function X that’s not declared above of main or any other function that calls X. II. main(): • Print a welcome message. • Call a function that utilize all the cars available (from array created above) and print them, so the client knows what options they have. • Ask them to choose the which car they want. They can input their answer based on the carID field from available_car struct. o Make sure to check for invalid answers. Ask for reinput until it’s valid. o I made my ID from 1->N. § You can tell them to input 0 if they don’t want to buy anything. § If this is the case, write some message and quit the program. • Ask the user if they will be the sole owner or will they cosign and save that info into order structure. o We assume that if there are 2 co-signers, each will pay 50%, 3 co-signers would be 33.33% each, and so on... • Print the price for the car that they have chosen, calculate how much EACH cosigner has to pay, and ask them if you are okay with this price and would like to process o If they answer No, write some message and quit the program. o If they answer Yes, § call another function to get the co-signers information (struct client) § when done getting the info, call another function to print back all the clients information § you can also print the price per person in the end when printing out each person’s cost. It’s up to you. III. other functions (it’ up to you to have more functions, I’m giving the 3 required functions. Doesn’t have to be exactly the same. This is a guide.): § printCars() function: o prints all the used cars the dealership have from the global array. § getClientInfo() function (or whatever you want to call it): o take an order struct o create an array of struct client. o loop through each client and get their information (name, creditScore,...) and save the info in the struct client array. o call printClientInfo() function to print back the information after. (You should not print the information right after the scanning the information, it should be done at the end and all the passengers info are printed together). § printClientInfo() function (or whatever you want to call it): o take an array of struct client o loop through each client and print out their information
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
C# programming question.
This task will be a car dealership where you’ll help the user find the car(s) they want. This
program should keep going until the user either decides to not continue with the purchase, or the
purchase is complete, and no more order is being processed.
You will need 3 structs to store:
• client information
• order information
• used_car information
Each struct would have the following fields:
struct used_car
{
int carID;
char brand[length];
char make[length];
int year;
int mileage;
float price;
};
struct order
{
int numCosigned;
int carID;
float pricePerPerson;
};
struct client
{
char firstName[length];
char lastName[length];
bool isEmployed;
int creditScore;
};
I. global variables:
• Create an array of struct used_car and add some cars in there. I put 4 cars. You can do as
many as you want (at least 3).
• Make sure to put the struct definition above the array creation, otherwise, it won’t work.
o This is similar to you trying to call a function X that’s not declared above of main
or any other function that calls X.
II. main():
program should keep going until the user either decides to not continue with the purchase, or the
purchase is complete, and no more order is being processed.
You will need 3 structs to store:
• client information
• order information
• used_car information
Each struct would have the following fields:
struct used_car
{
int carID;
char brand[length];
char make[length];
int year;
int mileage;
float price;
};
struct order
{
int numCosigned;
int carID;
float pricePerPerson;
};
struct client
{
char firstName[length];
char lastName[length];
bool isEmployed;
int creditScore;
};
I. global variables:
• Create an array of struct used_car and add some cars in there. I put 4 cars. You can do as
many as you want (at least 3).
• Make sure to put the struct definition above the array creation, otherwise, it won’t work.
o This is similar to you trying to call a function X that’s not declared above of main
or any other function that calls X.
II. main():
• Print a welcome message.
• Call a function that utilize all the cars available (from array created above) and print
them, so the client knows what options they have.
• Ask them to choose the which car they want. They can input their answer based on the
carID field from available_car struct.
o Make sure to check for invalid answers. Ask for reinput until it’s valid.
o I made my ID from 1->N.
§ You can tell them to input 0 if they don’t want to buy anything.
§ If this is the case, write some message and quit the program.
• Ask the user if they will be the sole owner or will they cosign and save that info into
order structure.
o We assume that if there are 2 co-signers, each will pay 50%, 3 co-signers would
be 33.33% each, and so on...
• Print the price for the car that they have chosen, calculate how much EACH cosigner has
to pay, and ask them if you are okay with this price and would like to process
o If they answer No, write some message and quit the program.
o If they answer Yes,
§ call another function to get the co-signers information (struct client)
§ when done getting the info, call another function to print back all the
clients information
§ you can also print the price per person in the end when printing out each
person’s cost. It’s up to you.
III. other functions (it’ up to you to have more functions, I’m giving the 3 required
functions. Doesn’t have to be exactly the same. This is a guide.):
§ printCars() function:
o prints all the used cars the dealership have from the global array.
§ getClientInfo() function (or whatever you want to call it):
o take an order struct
o create an array of struct client.
o loop through each client and get their information (name, creditScore,...) and
save the info in the struct client array.
o call printClientInfo() function to print back the information after.
(You should not print the information right after the scanning the information, it should
be done at the end and all the passengers info are printed together).
§ printClientInfo() function (or whatever you want to call it):
o take an array of struct client
o loop through each client and print out their information
• Call a function that utilize all the cars available (from array created above) and print
them, so the client knows what options they have.
• Ask them to choose the which car they want. They can input their answer based on the
carID field from available_car struct.
o Make sure to check for invalid answers. Ask for reinput until it’s valid.
o I made my ID from 1->N.
§ You can tell them to input 0 if they don’t want to buy anything.
§ If this is the case, write some message and quit the program.
• Ask the user if they will be the sole owner or will they cosign and save that info into
order structure.
o We assume that if there are 2 co-signers, each will pay 50%, 3 co-signers would
be 33.33% each, and so on...
• Print the price for the car that they have chosen, calculate how much EACH cosigner has
to pay, and ask them if you are okay with this price and would like to process
o If they answer No, write some message and quit the program.
o If they answer Yes,
§ call another function to get the co-signers information (struct client)
§ when done getting the info, call another function to print back all the
clients information
§ you can also print the price per person in the end when printing out each
person’s cost. It’s up to you.
III. other functions (it’ up to you to have more functions, I’m giving the 3 required
functions. Doesn’t have to be exactly the same. This is a guide.):
§ printCars() function:
o prints all the used cars the dealership have from the global array.
§ getClientInfo() function (or whatever you want to call it):
o take an order struct
o create an array of struct client.
o loop through each client and get their information (name, creditScore,...) and
save the info in the struct client array.
o call printClientInfo() function to print back the information after.
(You should not print the information right after the scanning the information, it should
be done at the end and all the passengers info are printed together).
§ printClientInfo() function (or whatever you want to call it):
o take an array of struct client
o loop through each client and print out their information
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 10 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Recommended textbooks for you
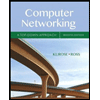
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
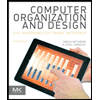
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
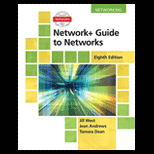
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
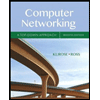
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
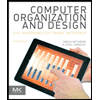
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
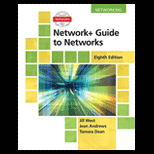
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
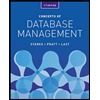
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
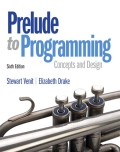
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
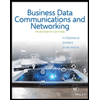
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY