C# Student Loan Calculator Loan Amount Number of Years In Defement? O Yes 3 O No Interest Rates Options O No Missed Payments O Automatic Withdrawal Calculate Annual Payment: 0.00 (Note: An "automatic withdrawal" occurs when you grant your bank permission to make a regular payment to a creditor from your bank account automatically - for example, mortgage loans, utility bills, health club memberships, or insurance premiums.) Your task You will implement a student loan calculator, utilizing the fundamentals of GUI development and basic coding as specified below: C# window should contain the following nodes/controls 1. Window Title – "Student Loan Calculator" 2. Label – "Loan Amount" 3. TextBox – input field for the amount of the loan 4. Label – "Number of Years" 5. ListBox - displays years 1- 20 via a scroll bar and allows the user to select only one year 6. Label – "Interest Rates" 7. ComboBox - displays rates (3.00, 3.50, 4.00, 4.50, 5.00, 5.50, 6.00, 6.50, 7.00, 7.50) via a drop-down menu and allows the user to select only one rate 8. GroupBox – named "In Deferment?", containing two RadioButtons labeled "Yes" and "No", RadioButton “No" should be selected as the default, only one RadioButton may be selected (not both) 9. GroupBox – named "Options", containing two CheckBoxes labeled "No Missed Payments" and "Automatic Withdrawal", one or both CheckBoxes may be checked 10. Label – "Annual Payment: " 11.Label – displays the calculated annual loan payment amount, has a default value of "0.00" 12.Button – labeled "Calculate", which performs the actions listed below in the section "Programming Logic"
C# Student Loan Calculator Loan Amount Number of Years In Defement? O Yes 3 O No Interest Rates Options O No Missed Payments O Automatic Withdrawal Calculate Annual Payment: 0.00 (Note: An "automatic withdrawal" occurs when you grant your bank permission to make a regular payment to a creditor from your bank account automatically - for example, mortgage loans, utility bills, health club memberships, or insurance premiums.) Your task You will implement a student loan calculator, utilizing the fundamentals of GUI development and basic coding as specified below: C# window should contain the following nodes/controls 1. Window Title – "Student Loan Calculator" 2. Label – "Loan Amount" 3. TextBox – input field for the amount of the loan 4. Label – "Number of Years" 5. ListBox - displays years 1- 20 via a scroll bar and allows the user to select only one year 6. Label – "Interest Rates" 7. ComboBox - displays rates (3.00, 3.50, 4.00, 4.50, 5.00, 5.50, 6.00, 6.50, 7.00, 7.50) via a drop-down menu and allows the user to select only one rate 8. GroupBox – named "In Deferment?", containing two RadioButtons labeled "Yes" and "No", RadioButton “No" should be selected as the default, only one RadioButton may be selected (not both) 9. GroupBox – named "Options", containing two CheckBoxes labeled "No Missed Payments" and "Automatic Withdrawal", one or both CheckBoxes may be checked 10. Label – "Annual Payment: " 11.Label – displays the calculated annual loan payment amount, has a default value of "0.00" 12.Button – labeled "Calculate", which performs the actions listed below in the section "Programming Logic"
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Help me with the code on C# please.
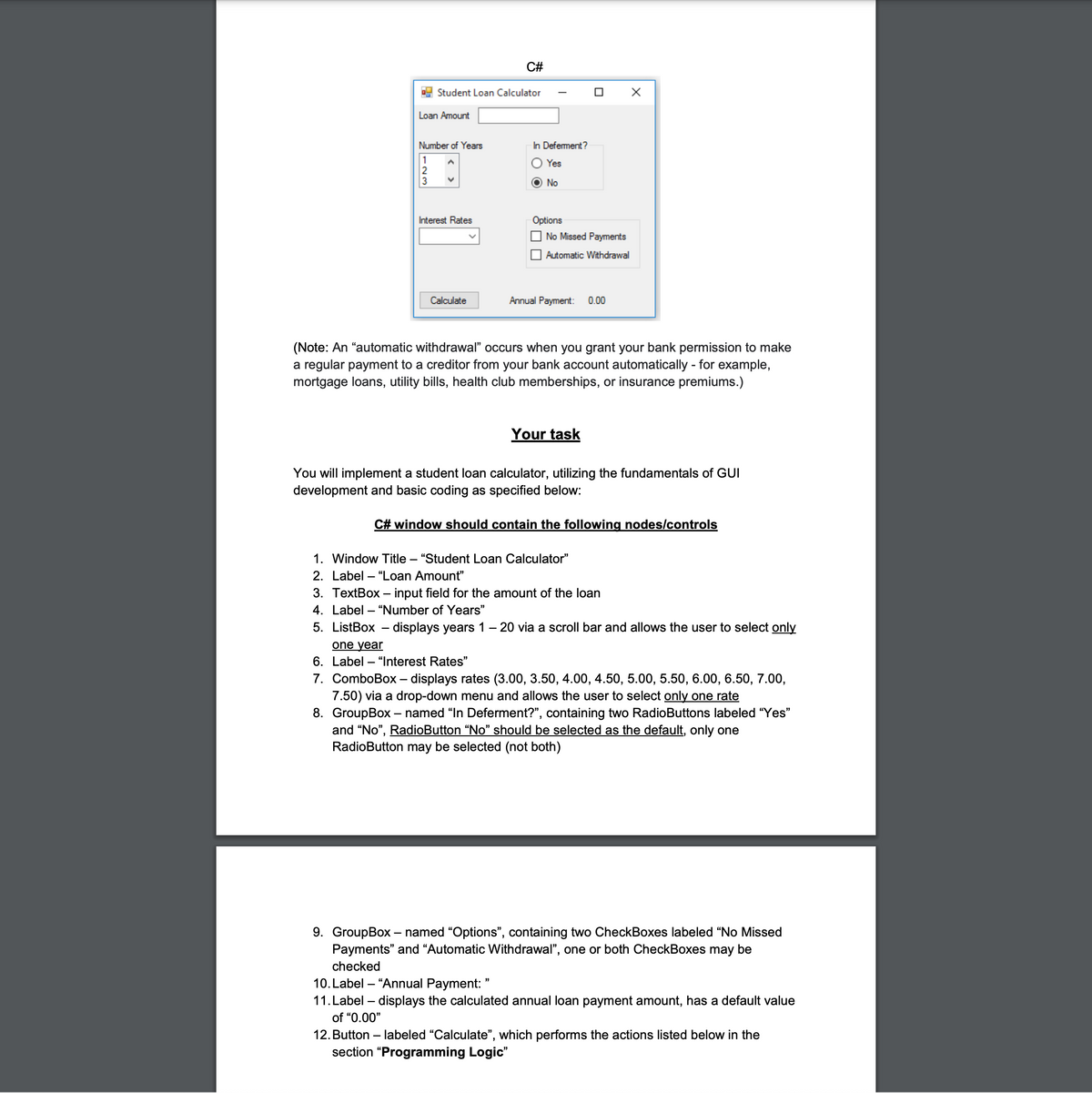
Transcribed Image Text:C#
Student Loan Calculator
Loan Amount
Number of Years
In Defement?
1
2
3
Yes
O No
Interest Rates
Options
No Missed Payments
Automatic Withdrawal
Calculate
Annual Payment:
0.00
(Note: An "automatic withdrawal" occurs when you grant your bank permission to make
a regular payment to a creditor from your bank account automatically - for example,
mortgage loans, utility bills, health club memberships, or insurance premiums.)
Your task
You will implement a student loan calculator, utilizing the fundamentals of GUI
development and basic coding as specified below:
C# window should contain the following nodes/controls
1. Window Title – "Student Loan Calculator"
2. Label – "Loan Amount"
3. TextBox – input field for the amount of the loan
4. Label – “Number of Years"
5. ListBox – displays years 1- 20 via a scroll bar and allows the user to select only
one year
6. Label – “Interest Rates"
7. ComboBox - displays rates (3.00, 3.50, 4.00, 4.50, 5.00, 5.50, 6.00, 6.50, 7.00,
7.50) via a drop-down menu and allows the user to select only one rate
8. GroupBox – named "In Deferment?", containing two RadioButtons labeled "Yes"
and “No", RadioButton “No" should be selected as the default, only one
RadioButton may be selected (not both)
9. GroupBox – named "Options", containing two CheckBoxes labeled "No Missed
Payments" and “Automatic Withdrawal", one or both CheckBoxes may be
checked
10. Label – “Annual Payment: "
11. Label – displays the calculated annual loan payment amount, has a default value
of "0.00"
12. Button – labeled "Calculate", which performs the actions listed below in the
section “Programming Logic"
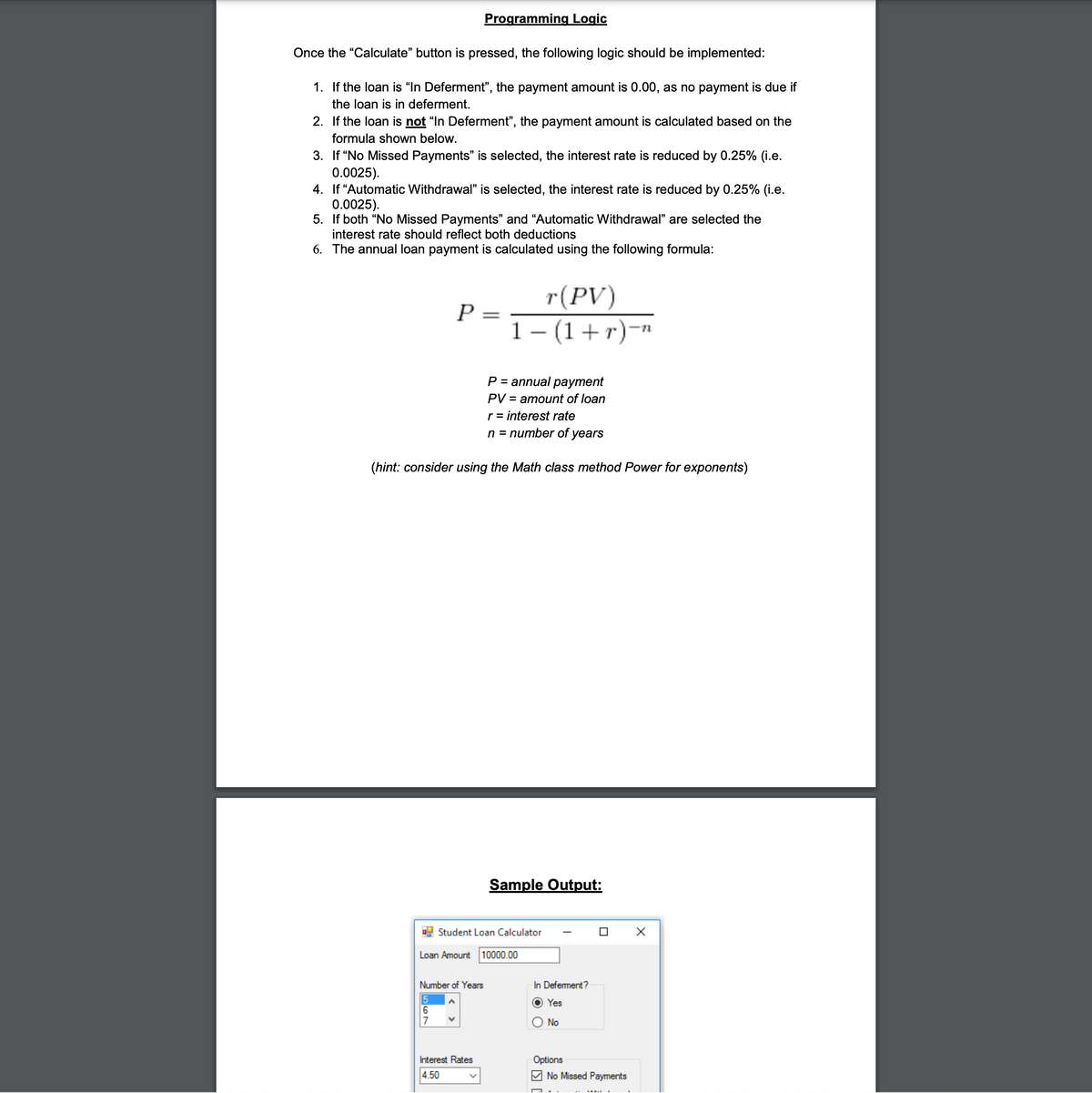
Transcribed Image Text:Programming Logic
Once the "Calculate" button is pressed, the following logic should be implemented:
1. If the loan is "In Deferment", the payment amount is 0.00, as no payment is due if
the loan is in deferment.
2. If the loan is not "In Deferment", the payment amount is calculated based on the
formula shown below.
3. If “No Missed Payments" is selected, the interest rate is reduced by 0.25% (i.e.
0.0025).
4. If “Automatic Withdrawal" is selected, the interest rate is reduced by 0.25% (i.e.
0.0025).
5. If both "No Missed Payments" and "Automatic Withdrawal" are selected the
interest rate should reflect both deductions
6. The annual loan payment is calculated using the following formula:
r(PV)
P =
1– (1+r)-
P = annual payment
PV = amount of loan
r = interest rate
n = number of years
(hint: consider using the Math class method Power for exponents)
Sample Output:
Student Loan Calculator
Loan Amount 10000.00
Number of Years
In Defement?
5
Yes
No
Interest Rates
Options
4.50
M No Missed Payments
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Recommended textbooks for you
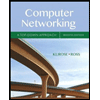
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
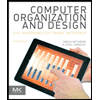
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
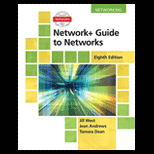
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
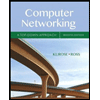
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
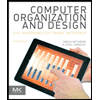
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
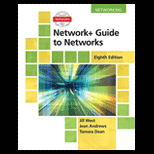
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
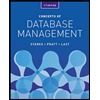
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
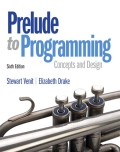
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
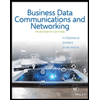
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY