c void main(String[] args) { Scanner scnr = new Scanner(System.in); ContactNode head = null; ContactNode current = null; // Read the name and phone number for three contacts and build a linked list. for (int i = 1; i <= 3; i++) { System.out.print(" " + i + ": "); String name = scnr.next(); System.out.print(" " + i + ": "); String phoneNumber = scnr.n
import java.util.Scanner;
public class ContactList {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
ContactNode head = null;
ContactNode current = null;
// Read the name and phone number for three contacts and build a linked list.
for (int i = 1; i <= 3; i++) {
System.out.print(" " + i + ": ");
String name = scnr.next();
System.out.print(" " + i + ": ");
String phoneNumber = scnr.next();
ContactNode newContact = new ContactNode(name, phoneNumber);
if (head == null) {
head = newContact;
current = head;
} else {
current.insertAfter(newContact);
current = newContact;
}
}
// Output each contact.
current = head;
for (int i = 1; current != null; i++) {
System.out.print("Person " + i + ": ");
current.printContactNode();
current = current.getNext();
}
}
}
class ContactNode {
private String contactName;
private String contactPhoneNumber;
private ContactNode nextNodePtr;
public ContactNode(String name, String phoneNumber) {
this.contactName = name;
this.contactPhoneNumber = phoneNumber;
this.nextNodePtr = null;
}
public String getName() {
return contactName;
}
public String getPhoneNumber() {
return contactPhoneNumber;
}
public ContactNode getNext() {
return nextNodePtr;
}
public void insertAfter(ContactNode node) {
ContactNode temp = this.nextNodePtr;
this.nextNodePtr = node;
node.nextNodePtr = temp;
}
public void printContactNode() {
System.out.println("Name: " + contactName + " Phone number: " + contactPhoneNumber);
}
}
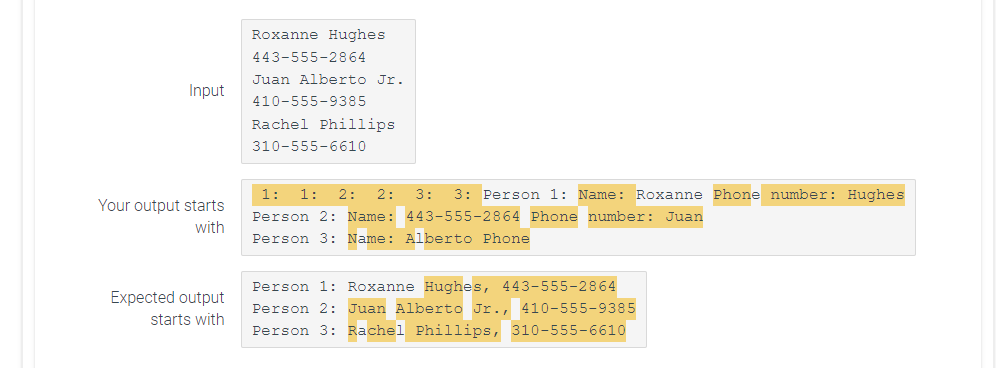
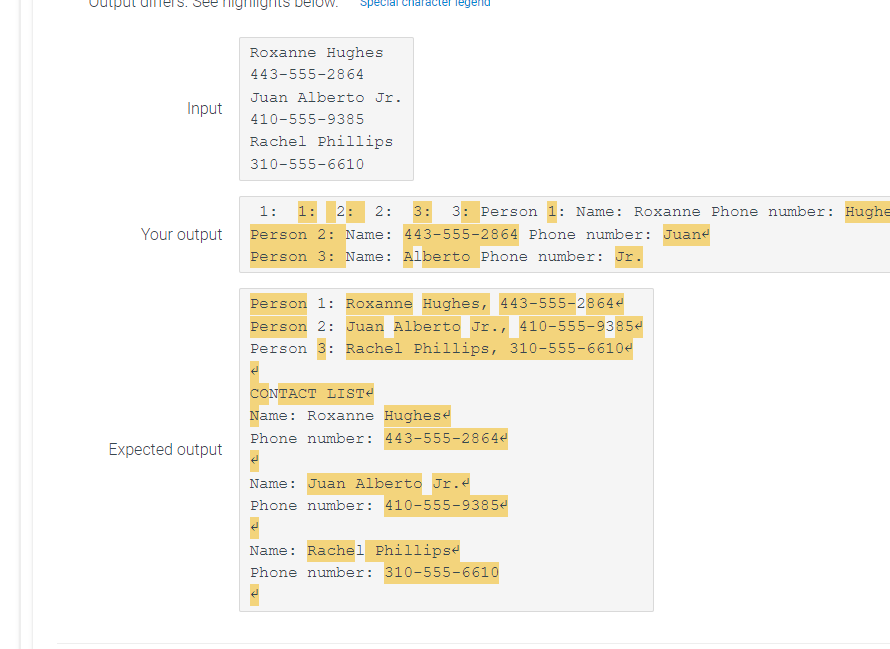

Step by step
Solved in 3 steps
